In this post, we will learn how to write a python program to find the Sum of digits of a given number with its algorithm and explanation in detail.
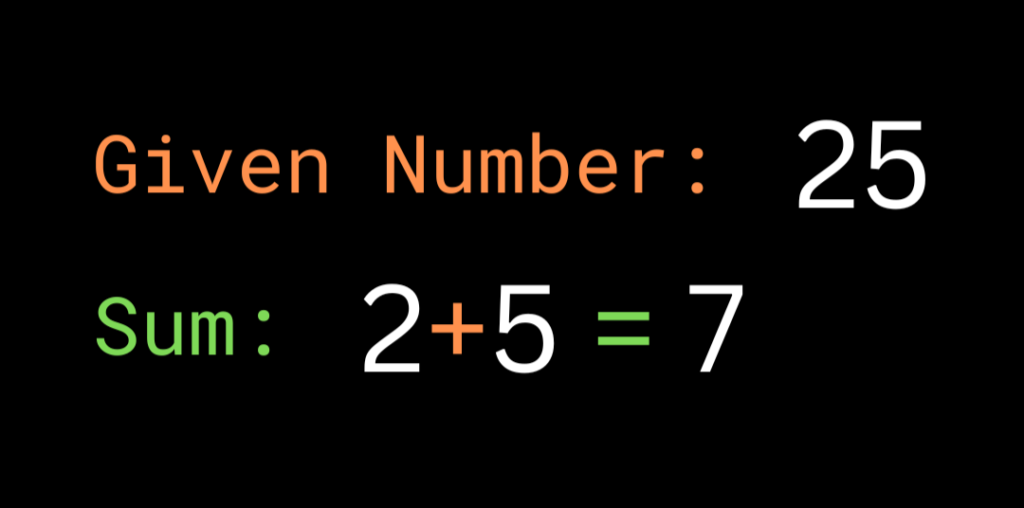
So let’s start with the algorithm.
Algorithm for Sum of Digits of a number
1. | Take integer input from the User and store it in a variable called “num“ |
2. | Take one variable to store the sum of digits and initially, it is zero ( sum_of_digit = 0 ). |
3. | while num != 0 do—-> sum_of_digit += num % 10 —-> num = num // 10 |
4. | Exit from the loop and print(sum_of_digit) |
From the above algorithm, we understood how to implement a python program to find the sum of digits of a number but before jumping into the programming part few programming concepts you have to know:
Source Code
num = int(input('Enter a number: '))
sum_of_digits = 0
while num != 0:
sum_of_digits += num % 10
num = num//10
print(f"Sum of Digits of a given number is {sum_of_digits}")
Output
Enter a number: 56
Sum of Digits of a given number is 11
Now let’s modify the above program and write it using a user-defined function.
Sum of Digits of a number in python Using Function
But before writing this program you should know about:
Source Code
def sum_of_digit(n):
total = 0
while n != 0:
total += n % 10
n = n // 10
return total
num = int(input('Enter a number: '))
print(f"Sum of Digits of a given number is {sum_of_digit(num)}")
Output
Enter a number: 78
Sum of Digits of a given number is 15
Sum of Digits of a number in python Using for-loop
There is one pythonic way to write this program in which we use the for-loop, str(), and int() functions. So let’s write this program using the pythonic approach and see the output.
But before writing this program you should know about:
Source Code
num = input('Enter a number : ')
# by default input() function take input in string
# that's why there is no need to convert into string using str() function
# but if you want to write directly without taking input from user
# then this is the step
# num = 25
# num = str(num)
sum_of_digits = 0
for i in num:
sum_of_digits += int(i)
print(f"Sum of Digits of a given number is {sum_of_digits}")
Output
Enter a number : 25
Sum of Digits of a given number is 7