In this post, we will learn how to print Floyd’s triangle in Python with a detailed explanation and example, but before we jump into the coding part, let’s first understand what Floyd’s triangle is.
What is Floyd’s Triangle?
Floyd’s Triangle is a right-angled triangular array of natural numbers. It is named after Robert W. Floyd. The triangle is constructed in such a way that the first row contains one element, the second row contains two elements, the third row contains three elements, and so on.
The numbers are sequentially arranged starting from 1 in the top left corner and increasing by 1 with each new element.
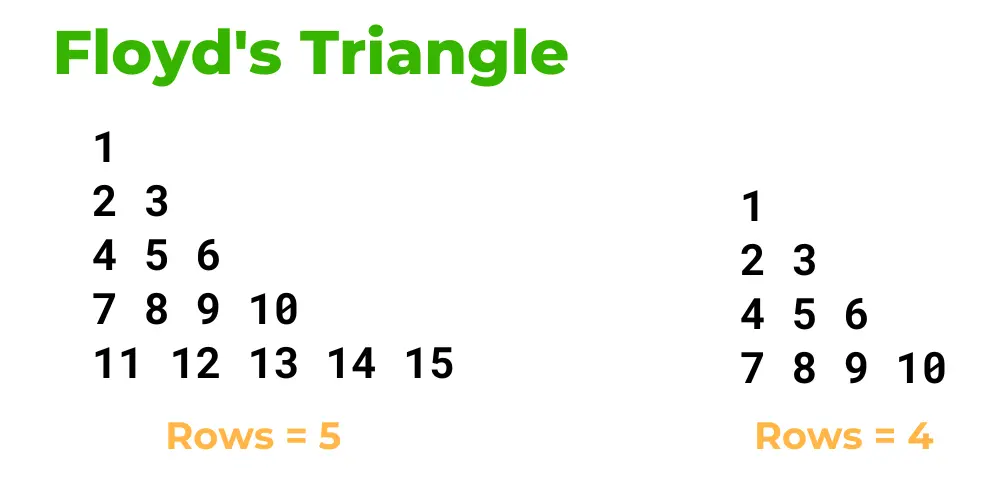
Algorithm
- Ask the user to enter the number of rows (
n
). - Set a variable called
num
to 1. - Use a nested for loop:
a. The outer loop iterates from 1 to n (inclusive) to represent each row.
b. The inner loop iterates from 1 to the current row number (inclusive). - Inside the nested loop:
a. Print the value of num.
b. Increment num by 1. - After the inner loop, print a newline character to move to the next row.
Now we know what Floyd’s triangle is and how we will implement it using Python, but before we jump into the program part, there are a few programming concepts you must know:
Source Code
n = int(input("Enter a number of rows: "))
num = 1
for i in range(1, n + 1):
for j in range(1, i + 1):
print(num, end=' ')
num += 1
print()
Output
Enter a number of rows: 5
1
2 3
4 5 6
7 8 9 10
11 12 13 14 15
We will also modify the above program and rewrite it using a Python function. Let’s see how.
Floyd’s Triangle in Python Using Function
def floyds_triangle(n):
num = 1
for i in range(1, n + 1):
for j in range(1, i + 1):
print(num, end=' ')
num += 1
print()
# Example usage
n = int(input("Enter a number of rows: "))
floyds_triangle(n)
Output
Enter a number of rows: 4
1
2 3
4 5 6
7 8 9 10