In this post, you will learn Python Operators with detailed explanations and examples. So let us start learning with the very basics.
Types of Operators in Python
There are seven types of operators in python they are as follows:
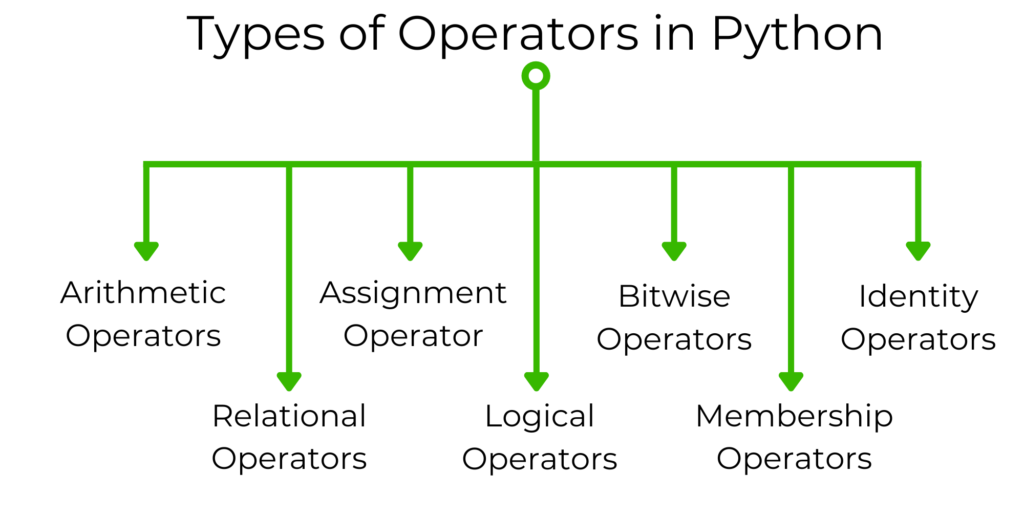
- Arithmetic Operators
- Relational Operators
- Assignment Operator
- Logical Operators
- Bitwise Operators
- Membership Operators
- Identity Operators
Arithmetic Operators
all mathematical operations such as addition, subtraction, multiplication, division, modulo, exponential, etc are included in Arithmetic Operators.
OPERATORS | USES |
+ | Use for addition |
– | Use for Substraction |
* | Use for Multiplication |
** | Use for exponential |
// | use for integer division |
/ | Use for Float division |
% | Gives remainder |
Let’s understand each operator seen in the above table with an example. Examples give you more clarity.
Addition & Substractions
# Addition and substraction
a = 2
b = 7
print(a+b)
print(a-b)
c = 4.5
d = 3
print(c+d)
print(c-d)
Output:
9
-5
7.5
1.5
Take Note if the addition of an integer number with a float number will give output as a float number.
This is because of Python auto type-casting, Python automatically promotes a lower data type to a higher data type while performing any arithmetic operation with two different data types like int or float as int is a lower data type as compared to float that’s why Python automatically promotes it to float to ensure that the operation is performed accurately.
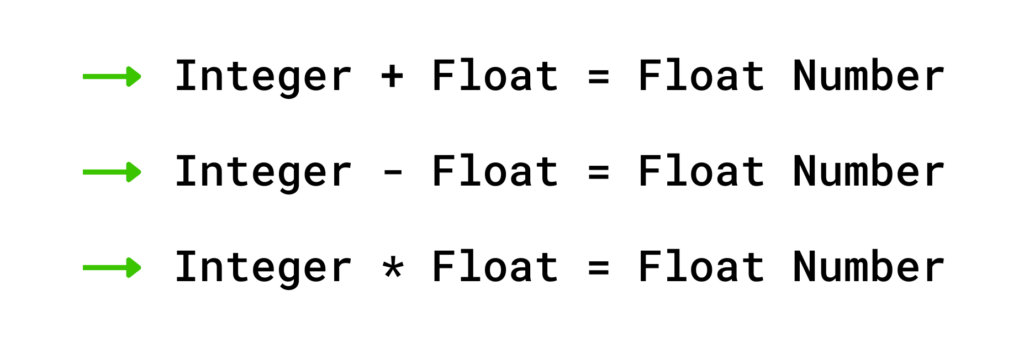
Multiplication (*) and Exponential (**)
# multiplication
a = 5
b = 7
print(a*b)
print(3.5*2)
# exponential ---> gives m^n (m power n)
print(2**3)
print(2.1**2)
Output:
35
7.0
8
4.41
Integer (//) & Float (/) division
# integer division
a = 5
b = 2
print(a//b) # remove number after the point (give wrong answer in this case)
# float division
print(a/b) # give right answer (recommended)
Output:
2
2.5
In integer division value after the point is not considered, which means if your answer is 2.5 it gives only 2.
float division is considered number after the point, Means float division is recommended way to perform division operations.
Modulo operator (%)
modulo operator gives remainder as output (modulo divide two numbers and give remainder as output)
a = 5
b = 3
print(a%b)
print(10%2)
Output:
2
0
Relational Operators
less than (<), greater than (>), not equal to (!=), less than equal to (<=), greater than equal to (>=) all are relational operators.
OPERATOR | USES |
< | Less than |
> | greater than |
<= | less than equal to |
>= | greater than equal to |
!= | not equal to |
let’s understand all the relational operators with the help of an example.
# less then and less then equal to
print(2<1) # if correct then return true other wise false
print(13<=15)
print(9<7)
# greater then and greater then equal to
print(23>20) # if correct then return true other wise false
print(15>=15)
print(12>15)
# not equal to
print(2!=2)
print(7!=5)
Output:
False
True
False
True
True
False
False
True
Assignment Operators
Assign (=), addition then assign (+=), subtraction then assign (-=), multiplication then assign (*=), etc are known as assignment operators.
take a look below table to understand all the assignment operators with there explaination.
OPERATOR | USES | EXAMPLES |
= | Assign value to left operant | a = 5 |
+= | add then assign to left operand | a+=7 is same as -> a = a+7 |
-= | Substract then assign to left operand | b-=5 is same as -> b = b-5 |
*= | Multiply then assign to left operand | c*=3 is same as -> c = c*3 |
/= | float division then assign to left operand | d/=2 is same as -> d = d/2 |
//= | integer division then assign to left operand | d/=4 is same as -> d = d/4 |
%= | gives remainder then assign to left operand | e%=2 is same as -> e = e%2 |
lets understand all assigment operators with the help of Example.
# Assign value to left operant (=)
num = 5
print(num)
# (+=)
num+=10 #num = num+10
print(num)
# (-=)
num-=10 #num = num-10
print(num)
# (*=)
num*=5 #num = num*5
print(num)
# (//=)
num//=2 #num =num//2
print(num)
# (/=)
num/=3 #num =num/3
print(num)
# (%=)
num%=2 #num = num%2
print(num)
Output:
5
15
5
25
12
4.0
0.0
Logical Operators
and, or and not are Known as logical operators.
OPERATOR | USES |
and | return true if both the condition is true |
or | return true if any one condition is true |
not | if condition true the return false or if condition is false then return true |
let’s understand logical operators with the help of examples
# logical operators
# and
print(5>3 and 4>1) # return true because both condition is true
print(5>3 and 4<1) # return False because one condition is false
# or
# return true if any one condition is true
print(10<5 or 5>3)
print(2<5 or 7<0)
# not
# reverse the answer
print(not(10>5))
Output:
True
False
True
True
False
Bitwise Operators
NOT (~), OR (|), AND (&), XOR (^) etc are known as bitwise operators
OPERATOR | USES |
~ | perform NOT operation |
| | perform OR operation |
& | perform AND operation |
^ | perform XOR operation |
example for bitwise operators.
# OR
a = 2 # in binary 2 = 1 0
b = 3 # in binary 3 = 1 1
c = a|b # perform OR-> 1 1 (any one value is one then output is one)
print("OR",c) #output: 1 1 (in binary) which is equal to 3
# AND
d = a&b
#2 # 1 0
#3 # 1 1
# AND 1 0 (in AND operation output is one if and only if both the value is 1)
print("AND",d)
# XOR
# (if one input is one then output is one)
e = a^b
# 1 0
# 1 1
# 0 1
print("XOR",e)
Output:
OR 3
AND 2
XOR 1
Membership Operators
in
and not in
are known as Membership Operators.
Membership Operator is used to check whether an element is present in sequence (list, tuple, string, etc) or not.
let’s understand Membership Operators with the help of examples
l1 = [1,2,3,4,5]
# in return True if element present in sequence otherwise False
print(1 in l1)
print(7 in l1)
# not in return True if element not present in sequence otherwise False
print(1 not in l1)
print(7 not in l1)
# string
s1 = 'allinpython'
print('a' in s1)
print('a' not in s1)
Output
True
False
False
True
True
False
Identity Operators
is
and not is
are known as Identity Operators.
is
returns True if two variables refer to the same object otherwise returns False
is not
returns True if two variables do not refer to the same object otherwise returns False
let’s understand Identity Operators with the help of examples
l1 = [1,2,3]
l2 = l1
print(l1 is l2) #return true because both are refer same object
l3 = [1,2,3]
print(l1 is l3) # return false because both are not refer to same object
print(l1 is not l3)
Output
True
False
True
hope this post adds some value to your life – thank you.
3 Comments
Op
Thank u for giving me a right knowledge.
your welcome