In this post, you will learn how to write a python program for the Fibonacci series with an algorithm but before we jump into the program let’s understand first what is Fibonacci series and its algorithm to implement it.
What is Fibonacci Series?
The first two numbers are fixed which is 0,1 and the sum of the previous two numbers will give the next number and that number sequence is known as the Fibonacci series.
Example:
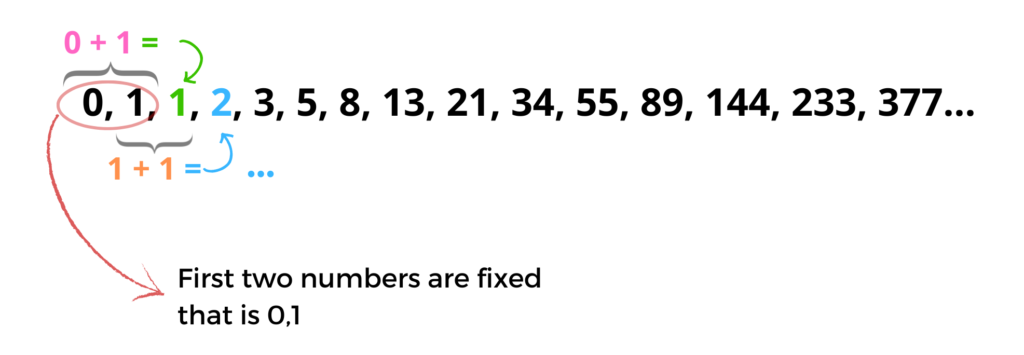
Algorithm for Fibonacci Series
Suppose you want to find the Fibonacci series for N numbers then the algorithm is:
- Take input from the user (
n
) - Take two variables
a
andb
, Initiallya = 0
&b = 1
. if n == 1
thenprint(a)
if n == 2
thenprint(a,b)
i = 0
while i < n-2
doc = a + b
andprint(c)
- After printing
c
, updatea = b
&b = c
. - At the end Increment
i+=1
. - End loop
Example using Algorithm:
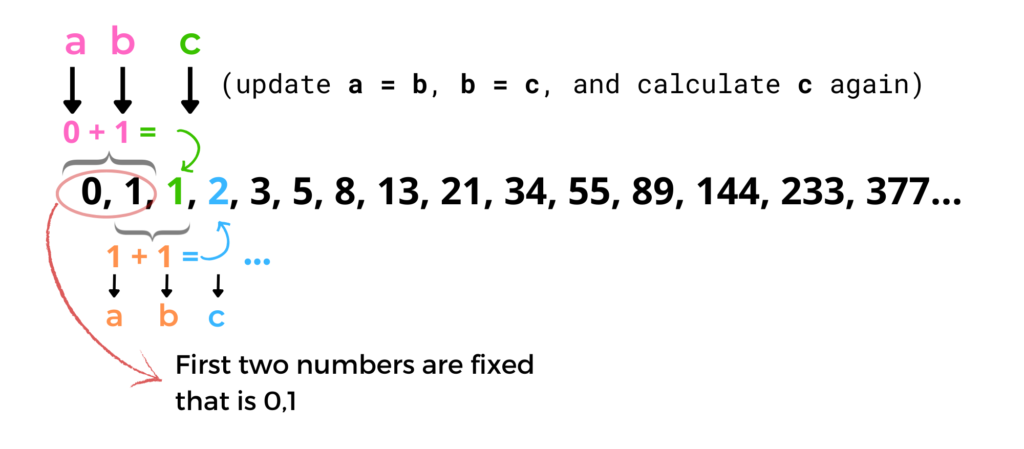
Now we understood how to implement a python program to print the Fibonacci series with the help of the above example and algorithm so let us start writing a program for it.
Write a python program for Fibonacci Series using while loop
Before writing this program few programming concepts you have to know and those are:
Source code
# 0 1 1 2 3 5 8 13 ...
# a b c
# a b c
# a b c
n = int(input("enter number for series :"))
a = 0
b = 1
if n <= 0:
print('Enter valid number (>0)')
elif n == 1:
print(a)
elif n == 2:
print(a,b)
else:
print(a, end=" ")
print(b, end=" ")
i = 0
while i < n-2:
c = a + b
a=b
b=c
print(c, end = " ")
i+=1
Output
enter number for series :11
0 1 1 2 3 5 8 13 21 34 55
Write a python program for Fibonacci Series using for loop
Before writing this program few programming concepts you have to know and those are:
Source code
n = int(input("enter number for series :"))
a = 0
b = 1
if n <= 0:
print('Enter valid number (>0)')
elif n == 1:
print(a)
elif n == 2:
print(a,b)
else:
print(a, end=" ")
print(b, end=" ")
for i in range(n-2):
c = a + b
a=b
b=c
print(c, end = " ")
Output
enter number for series :17
0 1 1 2 3 5 8 13 21 34 55 89 144 233 377 610 987
Write a python program for Fibonacci Series using the list
Before writing this program few programming concepts you have to know and those are:
Source code
n = int(input("enter number for series :"))
a = 0
b = 1
fibo_list = []
if n <= 0:
print('Enter valid number (>0)')
elif n == 1:
fibo_list.append(a)
print(fibo_list)
elif n == 2:
fibo_list.append(a)
fibo_list.append(b)
print(fibo_list)
else:
fibo_list.append(a)
fibo_list.append(b)
for i in range(n-2):
c = a + b
fibo_list.append(c)
a=b
b=c
print(fibo_list)
Output
enter number for series :10
[0, 1, 1, 2, 3, 5, 8, 13, 21, 34]
Write a python program for Fibonacci Series using the function
Before writing this program few programming concepts you have to know and those are:
Source code
def fibonacci(num):
a = 0
b = 1
if num == 1:
print(a, end=" ")
elif num == 2:
print(a,b)
else:
print(a, end=" ")
print(b, end=" ")
i = 0
while i < num-2:
c = a + b
a=b
b=c
print(c, end = " ")
i+=1
# return c
n = int(input("enter number for series :"))
if n<= 0:
print('Enter number greater then 0')
else:
fibonacci(n)
Output
enter number for series :5
0 1 1 2 3
Write a python program for Fibonacci Series using the recursion
Before writing this program few programming concepts you have to know and those are:
- if-elif-else
- while loop &
- function in python
- recursion
Source code
def fibo(n):
a = 0
b = 1
if n == 1:
return a
elif n == 2:
return b
else:
return fibo(n-1) + fibo(n-2)
n = int(input('ENter a number: '))
if n <=0:
print('Enter valid number (>0)')
else:
l = [str(fibo(i)) for i in range(1,n+1)]
# print(l)
print(','.join(l))
Output
ENter a number: 7
0,1,1,2,3,5,8