In this post, you will learn about the if-elif-else
statement in python with detailed explanations and examples.
So let’s start learning from the very basics:
if-elif-else in Python
if-elif-else
statements allow you to check multiple conditions in order, and once a condition is found to be true, the corresponding block of code is executed, and the program exits from the if-elif-else
statements. If no conditions are true, the code in the else
block is executed.
num1 = 5
num2 = 7
if num1 > num2:
print('num1 is greater then num2')
elif num1 < num2:
print('num1 is not greater then num2')
else:
print('both are equal')
Output:
num1 is not greater then num2
Syntax of if-elif-else block
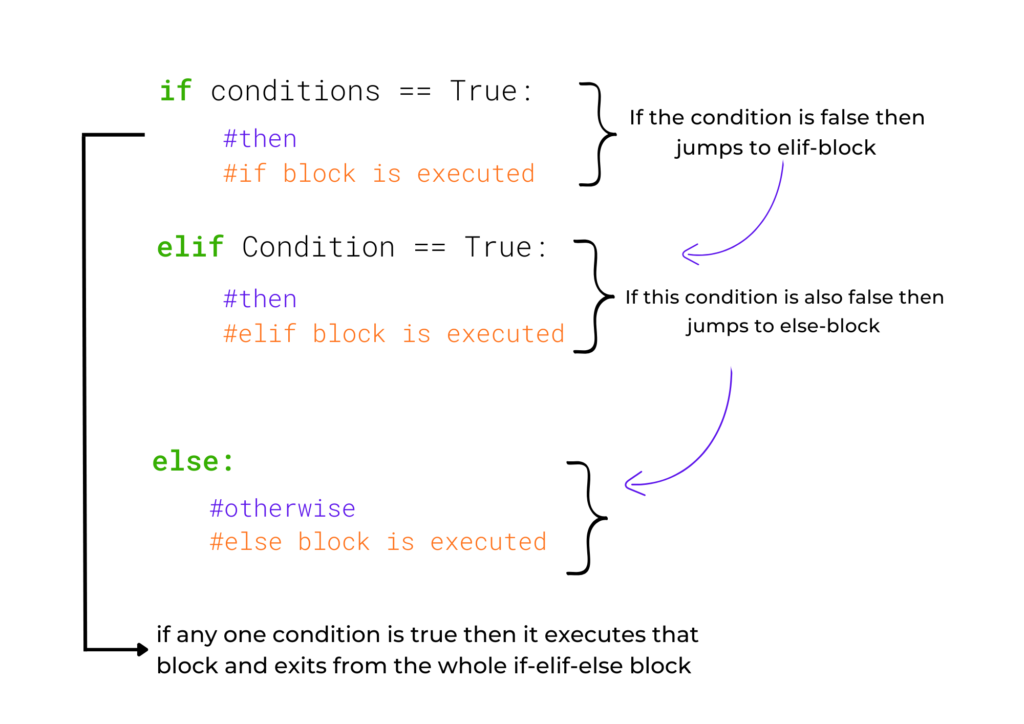
- First, if-block is checked if the condition is true then it is executed otherwise it jumps to elif-block.
- then elif-block is checked if the condition is true then it is executed otherwise it jumps to else-block.
- after that else block is executed
NOTE:
- else-block is optional.
- you can add multiple elif-blocks after the if-block.
#ticket price
# 1-3year old -->free
# 4-10year old----> 150rs
# 11-60year old---> 250rs
# above 60 old--->200rs
age=int(input("enter your age: "))
if age==0 or age<0:
print("invalid age please enter right age:")
elif 0<age<=3:
print("your ticket price is FREE")
elif 3<age<=10:
print("your ticket price is rs 150")
elif 10<age<=60:
print("your ticket price is rs 250")
else:
print("your ticket price is rs 200")
Output-1:
enter your age: 10
your ticket price is rs 150
Output-2
enter your age: 75
your ticket price is rs 200
Remember that if-elif-else
statements let you check multiple conditions in a specific order and when a condition is found to be true, the corresponding code block is executed, while the other code blocks are skipped. If none of the conditions are true, the code in the else
block is executed.
Examples for Practice
Question: Python program to print Fibonacci numbers sequence.
def fibo(num):
a = 0
b = 1
if num == 1:
print(a, end=" ")
elif num == 2:
print(a,b)
else:
print(a, end=" ")
print(b, end=" ")
i = 0
while i < num-1:
c = a + b
a=b
b=c
print(c, end = " ")
i+=1
n = int(input("enter number for series :"))
fibo(n)
Output-1:
enter number for series :10
0 1 1 2 3 5 8 13 21 34 55
Output-2:
enter number for series :5
0 1 1 2 3 5
Related topics: