In this post, you will learn 5 different ways to swap two numbers in python with a detailed explanation.
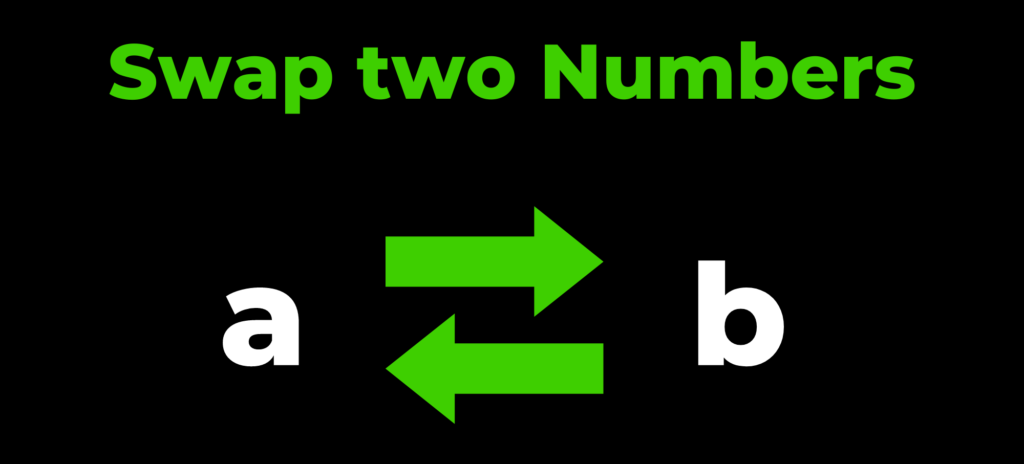
So let us start learning all the 5 ways one by one.
Swap two numbers using comma Operator
It is very simple to swap two numbers in python using the comma operator.
let’s see it with the help of the example.
Source Code
a = int(input('Enter first number (a) : '))
b = int(input('Enter second number (b) : '))
print('before swapping')
print('a =',a)
print('b =',b)
# Swap two numbers using comma Operator
a,b = b,a
print('After swapping')
print('a =',a)
print('b =',b)
Output
Enter first number (a) : 5 Enter second number (b) : 7 before swapping a = 5 b = 7 After swapping a = 7 b = 5 |
Swap two numbers using a temporary variable
It is a very basic way to swap two numbers Using a temporary variable and a very common way to swap two numbers in any programming language.
let’s see it with the help of the example.
Source Code
a = int(input('Enter first number (a) : '))
b = int(input('Enter second number (b) : '))
print('before swapping')
print('a =',a)
print('b =',b)
# taking third Variable 'temp' to swap two numbers
temp = a
a = b
b = temp
print('After swapping')
print('a =',a)
print('b =',b)
Output
Enter first number (a) : 11 Enter second number (b) : 4 before swapping a = 11 b = 4 After swapping a = 4 b = 11 |
Here Variable temp
act as a container and with the help of Variable temp
we swap a
& b
.
Swap two numbers using Arithmetic Operator (+,-)
With the help of the arithmetic operator (+
, -
) you can easily swap two numbers.
Below example helps you to understand how to swap two numbers using the arithmetic operator (+
, -
).
Source Code
a = int(input('Enter first number (a) : '))
b = int(input('Enter second number (b) : '))
print('before swapping')
print('a =',a)
print('b =',b)
# Swap two numbers Using addition and subtraction operator
a = a + b
b = a - b
a = a - b
print('After swapping')
print('a =',a)
print('b =',b)
Output
Enter first number (a) : 8 Enter second number (b) : 9 before swapping a = 8 b = 9 After swapping a = 9 b = 8 |
Swap two numbers using Arithmetic Operator (*,/)
With the help of the arithmetic operator (*
, /
) you can easily swap two numbers.
let’s see it with the help of the example.
Source Code
a = int(input('Enter first number (a) : '))
b = int(input('Enter second number (b) : '))
print('before swapping')
print('a =',a)
print('b =',b)
# Swap two numbers Using multiplication and division operator
a = a*b
b = a//b
a = a//b
print('After swapping')
print('a =',a)
print('b =',b)
Output
Enter first number (a) : 19 Enter second number (b) : 12 before swapping a = 19 b = 12 After swapping a = 12 b = 19 |
Swap two numbers using XOR (^) Operator
You can also use XOR (^) operator to swap two numbers.
let’s see the source code for a better understanding.
Source Code
a = int(input('Enter first number (a) : '))
b = int(input('Enter second number (b) : '))
print('before swapping')
print('a =',a)
print('b =',b)
# Swap two numbers Using XOR (^) operator
a = a^b
b = a^b
a = a^b
print('After swapping')
print('a =',a)
print('b =',b)
Output
Enter first number (a) : 3 Enter second number (b) : 1 before swapping a = 3 b = 1 After swapping a = 1 b = 3 |
1 Comment
This is the type of content a beginner searchs for, each and every step is clearly shown.
There are five solution of a problem,. It helps to build logic.
That’s amazing 🥳