In this post, you will learn the python program to transpose a matrix with user input but before writing a program let’s understand Transpose of a matrix and the algorithm to implement it.
What is the Transpose of a Matrix?
If a number of elements present in rows interchange with a number of elements present in the column of the matrix then it is known as the Transpose of a matrix.
For Example:
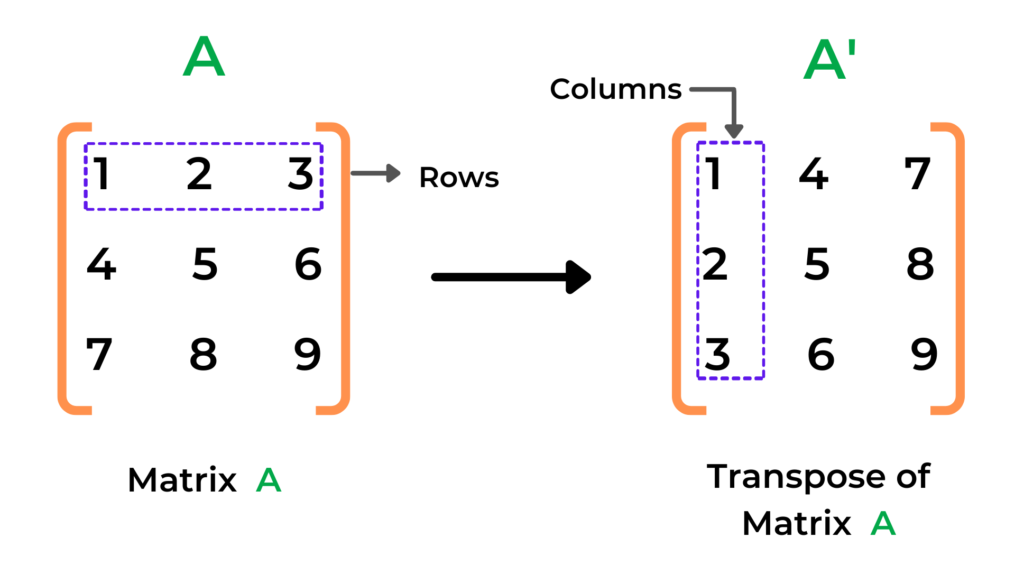
Algorithm for Transpose of a Matrix
1 | Ask the user for the inputs of Rows & Columns of the matrix |
2 | Create the matrix of m x n by using the input() function and nested list comprehension. Here m = number of Rows and n = number of columns |
3 | For storing the result create another matrix of n x m basically, the resultant matrix is inverted of your original matrix and initially, it is Zero. |
4 | use nested for-loop and do transpose[j][i] = matrix[i][j] |
5 | the last step is to print the transpose of a matrix. |
Through the above algorithm hope you understand how to implement the transpose of a matrix but before writing a program few programming concepts you have to know and they are:
Source code
row = int(input('Enter number of row: '))
col = int(input('Enter number of col: '))
# create a matrix using input() function and nested list comprehension
matrix = [[int(input(f'column {j+1} -> ENter {i+1} element:')) for j in range(col)] for i in range(row)]
# create another list where we store result (transpose of matrix)
transpose = [[0 for i in range(row)] for j in range(col)]
print() # for new line
# print matrix
print('matrix: ')
for i in matrix:
print(i)
# transpose of matrix
for i in range(row):
for j in range(col):
transpose[j][i] = matrix[i][j]
print() # new line
# print transpose of matrix
print('Transpose of matrix: ')
for i in transpose:
print(i)
Output-1
Enter number of row: 3
Enter number of col: 3
column 1 -> ENter 1 element:1
column 2 -> ENter 1 element:2
column 3 -> ENter 1 element:3
column 1 -> ENter 2 element:4
column 2 -> ENter 2 element:5
column 3 -> ENter 2 element:6
column 1 -> ENter 3 element:7
column 2 -> ENter 3 element:8
column 3 -> ENter 3 element:9
matrix:
[1, 2, 3]
[4, 5, 6]
[7, 8, 9]
Transpose of matrix:
[1, 4, 7]
[2, 5, 8]
[3, 6, 9]
Output-2
Enter number of row: 2
Enter number of col: 3
column 1 -> ENter 1 element:1
column 2 -> ENter 1 element:2
column 3 -> ENter 1 element:3
column 1 -> ENter 2 element:4
column 2 -> ENter 2 element:5
column 3 -> ENter 2 element:6
matrix:
[1, 2, 3]
[4, 5, 6]
Transpose of matrix:
[1, 4]
[2, 5]
[3, 6]
1 Comment
This post help me lot keep doing brother