In this post, we will write a Python program to sort words in alphabetic order with detailed explanation and example.
But before we jump into the programming part, let’s first discuss algorithms for how we can sort the words in alphabetical order.
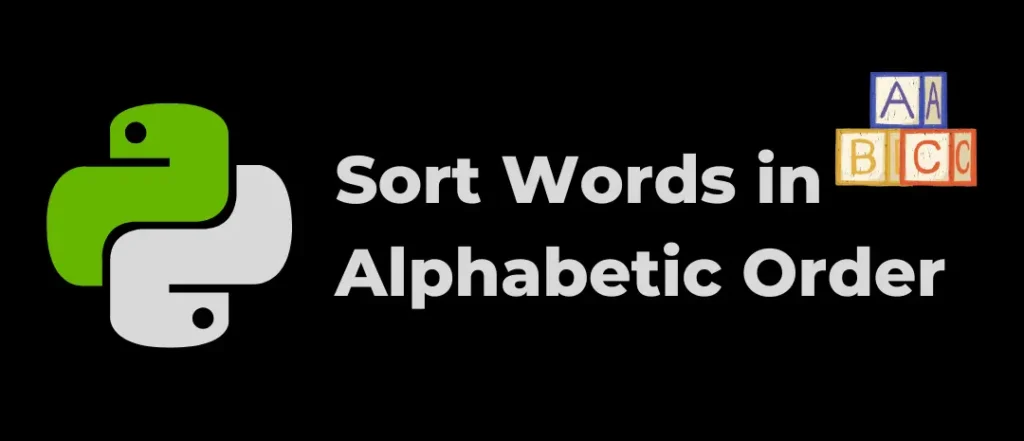
Algorithm
- Ask the user to enter their sentence and store it in a variable called
words
. - Transform
words
into lowercase using the.lower()
method. - In the 3rd step split the
words
into a Python list using the.split()
method and store the list in the variable calledwords_list
. - After splitting words into a Python list, sort the list using the
.sort()
method. - Join the
words_list
into a single string using the.join()
method and store it in a variable calledsorted_words
. - At the end print the
sorted_words
.
From the above algorithm, now we know how to write a Python program to sort words in alphabetical order, but before we jump into the programming part, there are a few programming concepts you have to know:
- How to take input from the user
- Python string methods (
.lower()
,.split()
&.join()
).
Source Code
# User input
words = input("Enter your sentence: ")
# Transform it into lowercase
words = words.lower()
# Split words into Python list
words_list = words.split()
# sort words_list
words_list.sort()
# join sorted_list into single string
sorted_words = " ".join(words_list)
print("Sorted words:")
print(sorted_words)
Output
Enter your sentence: This is Python learning site
Sorted words:
is learning python site this
We can also modify this program and write it using Python functions, Let’s see how.
Sort Words in Alphabetic Order in Python Using Function
# define a function
def sorted_words(w):
return " ".join(sorted(w.lower().split())) # Here we use sorted() function because .sort() method do not return anything.
# User input
words = input("Enter your sentence: ")
# call a function & print it
print("Sorted words:")
print(sorted_words(words))
Output:
Enter your sentence: This is my sentence
Sorted words:
is my sentence this