In this post, we will learn how to write a program to check for leap year but before writing a program first we should know what is a leap year.
What is Leap year?
A year in which 29 days in the month of February is called a leap year (that means there are 366 days in this year).

We should write this program in three different ways
- using if-else (In a simple way by taking user input)
- using function
- except exception, if the user inputs the wrong data (using try-except)
Now let’s understand how we implement the leap year program with the help of a flowchart.
Flowchart for Leap year
The flowchart helps us to write a program more efficiently and effectively. it is recommended that before writing a program create a flowchart for your program (it helps you a lot).
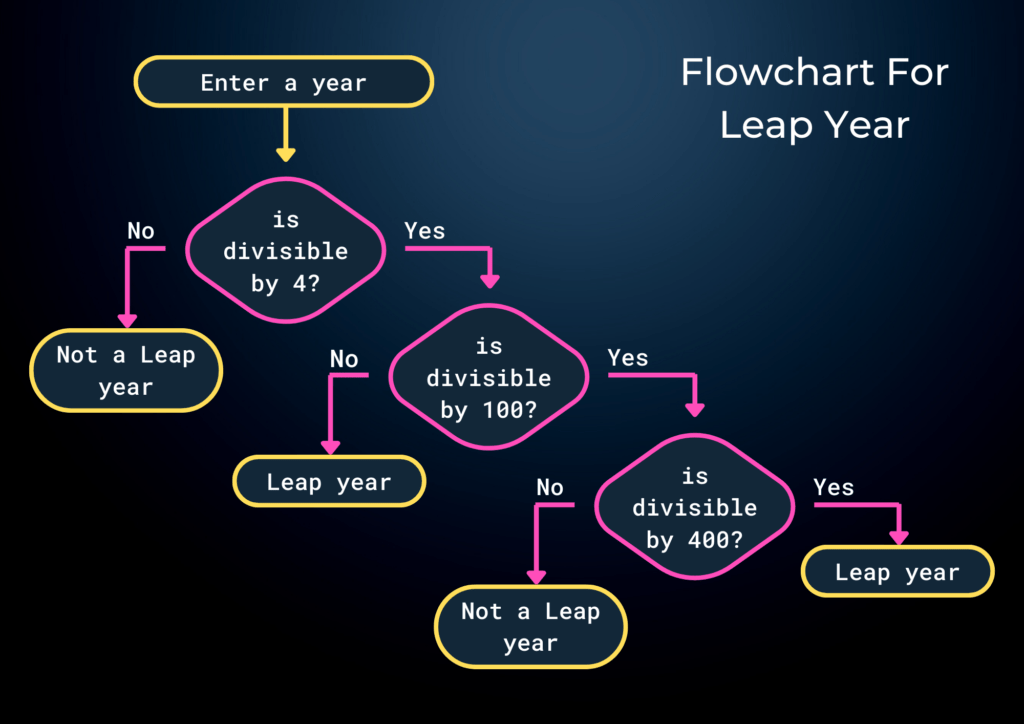
Algorithm
- if the year is divisible by 4 then go to step number 2 otherwise year is not a leap year.
- if the year is divisible by 100 then go to step number 3 otherwise year is leap year.
- if the year is divisible by 400 then the year is a leap year otherwise it is not a leap year.
Now we understand how to write a program to check for leap year with the help of the above flowchart and algorithm so let’s start writing the program.
1. using if-else (In simple way by taking user input)
Write a program to check for leap year by taking user input.
Before writing this program you should know about:
Source code:
num = int(input('Enter a year: '))
if num%4 == 0:
if num % 100 == 0:
if num%400 == 0:
print(f'{num} is leap year')
else:
print(f'{num} is not leap year')
else:
print(f'{num} is leap year')
else:
print(f'{num} is not leap year ')
Output-1:
Enter a year: 2012
2012 is leap year
Output-2:
Enter a year: 2013
2013 is not leap year
2. Using function
Write A program to check for leap year by using the function.
for understanding this program you should know about:
Source code:
def check_leap_year(year):
if year % 4 == 0:
if year % 100 == 0:
if year % 400 == 0:
print(f"{year} is a leap year")
else:
print(f"{year} is not a leap year")
else:
print(f"{year} is a leap year")
else:
print(f"{year} is not a leap year")
year = int(input("Enter a year to check for leap year :"))
check_leap_year(year)
Output-1:
Enter a year to check for leap year :2015
2015 is not a leap year
Output-2:
Enter a year to check for leap year :2020
2020 is a leap year
3. except exceptions if user input wrong data
Write a program to check for leap year and also except exception if the user input wrong data with the help of the try-except block.
Before writing this program you should know about:
Source code:
def check_leap_year(year):
if year % 4 == 0:
if year % 100 == 0:
if year % 400 == 0:
print(f"{year} is a leap year")
else:
print(f"{year} is not a leap year")
else:
print(f"{year} is a leap year")
else:
print(f"{year} is not a leap year")
while True:
try:
year = int(input("Enter a year to check for leap year :"))
break
except Exception:
print("pls enter numberic value only! and try again")
check_leap_year(year)
Output:
Enter a year to check for leap year :two thousand twenty-seven
pls enter numberic value only! and try again
Enter a year to check for leap year :2027
2027 is not a leap year
Hope this post adds some value to your life -thank you