In this post, you will learn how to write a python program to check for a palindrome string using recursion with a detailed explanation but before writing a program you should know about what is palindrome string.
What is a Palindrome string?
If a string is the same as its reverse then that string is known as a palindrome string. for example, REFER.
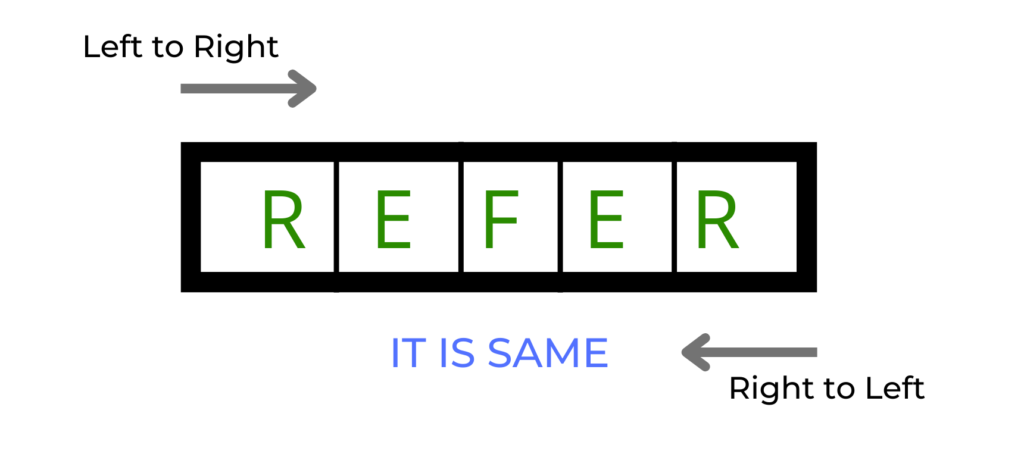
Algorithm
- Take two pointers L & R.
- L points to the first character of the string and R points to the last character of the String.
- if
L >= R
then returns True (which means it is a palindrome) if S[L] != S[R]
then returns False (which means it is not palindrome).- otherwise return recursive function
is_palindrome(L+1, R-1,S)
.
Now we understood how to implement the palindrome program using recursion with help of an algorithm.
But before writing a program you should now about:
- if-else
- function in python &
- How recursion works.
Source code
def is_palindrome(l,r,S):
if l>=r:
return True
if S[l] != S[r]:
return False
return is_palindrome(l+1,r-1,S)
my_string = input('Enter your string: ')
l = 0
r = len(my_string)-1
check = is_palindrome(l,r,my_string)
if check:
print(f"{my_string} is palindrome")
else:
print(f"{my_string} is not palindrome")
Output
Enter your string: refer
refer is palindrome
Explanation
Let’s understand how the palindrome program works with the help of an example.
NOTE: Here in this program we use two pointer approach.
Initially pointer L point to the first character of the string and pointer R point to the last character of the string.
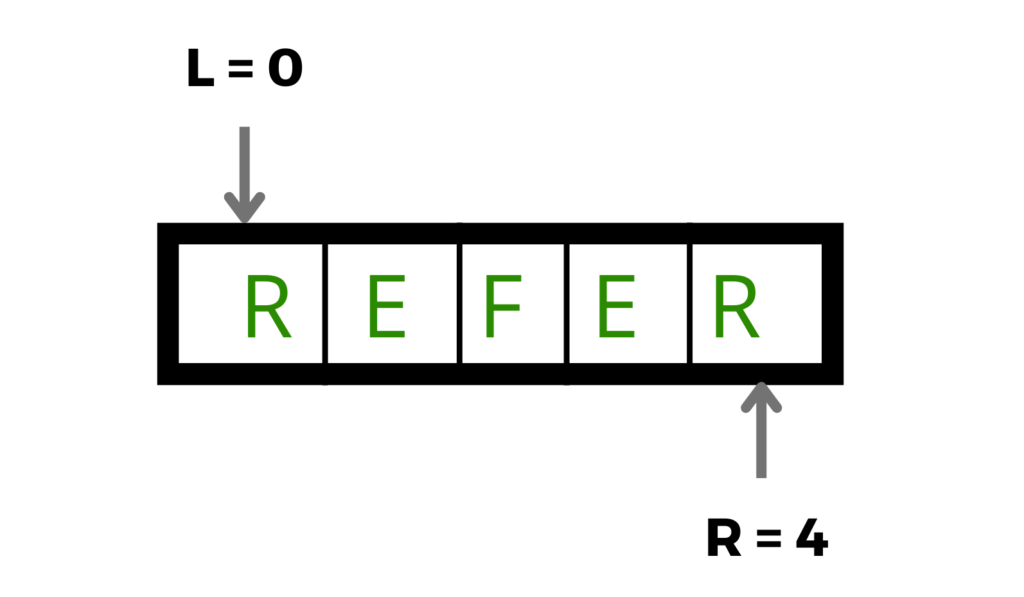
Check If L and R both are the same (if S[L] == S[R]
) then increment L by 1 (L+1
) and decrement R by 1 (R-1
).
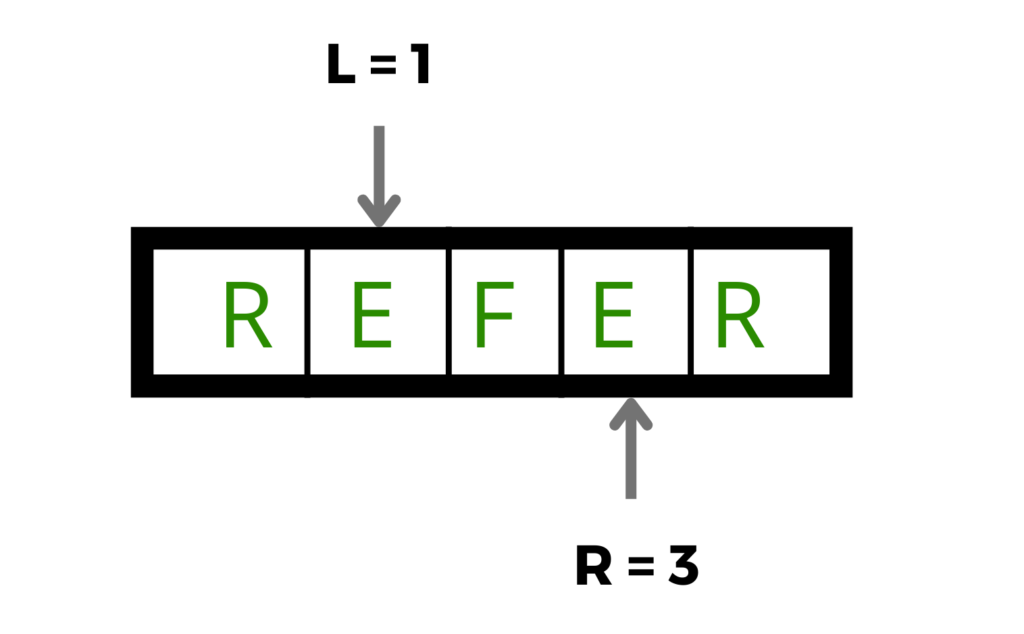
Check again if L & R (if S[L] == S[R]
) both are the same then perform the same task again increment L (L+1
) and decrement R (R-1
).
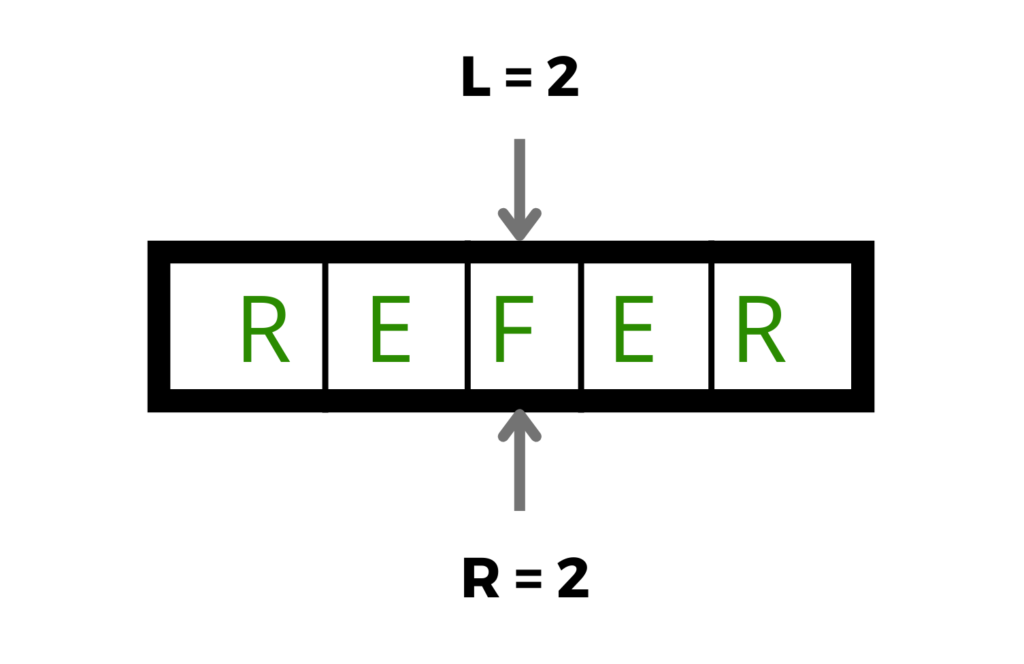
In this case L = R this means the given string is a palindrome.
This is how the palindrome program works in python.
you can also check it by taking a non-palindrome string for your understanding.