In this post, you will learn what is linear search in python with a detailed explanation and source code.
Linear Search
Linear Search is a searching algorithm in which we sequentially search for a presence of a particular element inside a list or array.
Example:
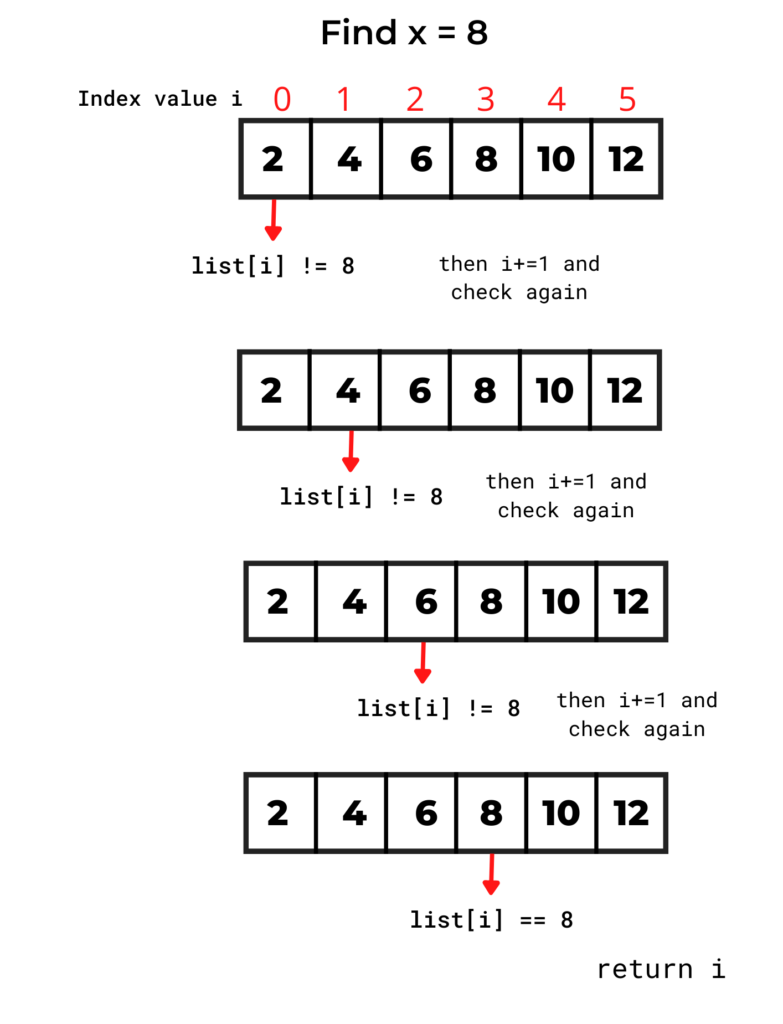
Algorithm for Linear Search
Suppose we have a list of even numbers such as L1 = [2,4,6,8,10,12]
and user want to find element x = 8.
1. | apply while loop on list L1 –> while i < len(l1): |
2. | Inside while-loop check if L1[i] == x then print i and break a loop(because you found x at i th position) |
3. | Otherwise outside of the while-loop checkif i >= len(L1) then print( 'element is not present inside a list) |
Source Code
l1 = [2,4,6,8,10,12]
x = 8 # element that we want to be found
i = 0 # pointer
while i < len(l1):
if l1[i] == x:
print(f'element {x} present at {i}th position')
break
i+=1
if i >= len(l1):
print('Element is not present')
Output
element 8 present at 3th position
Linear Search in python using function
Question: Write a Linear Search program in python using Function
Few concepts of python you should know before writing a program:
Algorithm for Linear Search using function
1. | Define a function –>def Linear_search(L1,x): |
2. | apply for-loop on list L1 –> for i in range(len(L1)): |
3. | Inside for-loop check if L1[i] == x then return i . |
4. | Otherwise outside of the for-loop return -1 . |
Source code
def LinearSearch(l1, element):
for i in range(len(l1)):
if l1[i] == element:
return i #return index value
return -1 # if element is not found then it return -1
list1 = [2,4,6,8,10,12]
x = 8
print(LinearSearch(list1,x))
Output
3
Linear Search in python using using recursion
Question: Write a Linear Search program in python using recursion.
Few concepts of python you should know before writing program:
- Function in python
- recursive function in python &
- for-loop in python.
Algorithm for Linear Search using recursion
1. | Define a function –>def Linear_search(L1,element,pointer): Here pointer initially points to the first element of the list. |
2. | if pointer >= len(L1) then return -1 |
3. | if L1[pointer] == element then return pointer . |
4. | Otherwisereturn Linear_search(L1,element,pointer+1). |
Source Code
def Linear_search(l1,element,pointer):
if pointer >= len(l1):
return -1
if l1[pointer] == element:
return pointer
return Linear_search(l1,element,pointer+1)
list1 = [2,4,6,8,10,12]
x = 8
i = 0 #pointer
print(Linear_search(list1,x,i))
Output
3