In this post, we will learn how to reverse a list in Python using a for-loop with a very simple explanation and example.
As we know, there are various built-in methods in Python to reverse a list. Even you can use slicing to reverse a list but here we do not use those methods instead we use only for-loop to reverse a list and trust me it is a very simple approach.
So without any further delay, let’s see the steps to write a Python program to reverse a list using for loop.
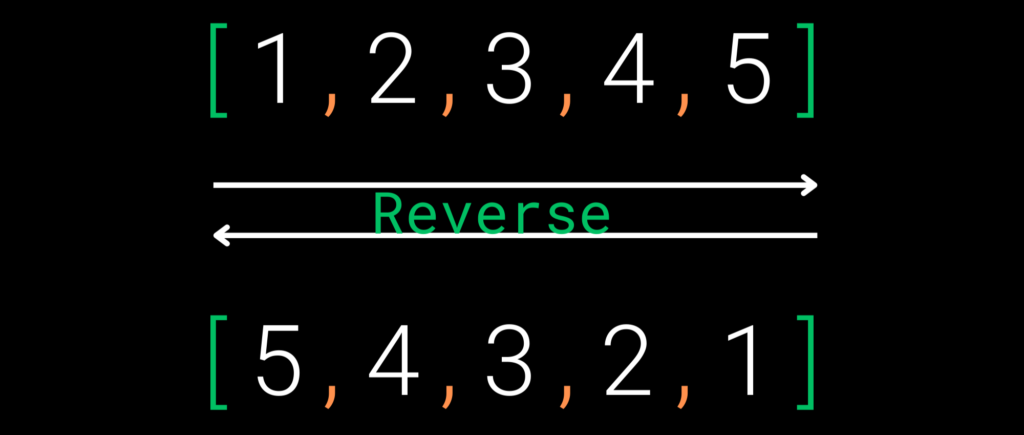
Steps to Reverse a List Using for-loop
- Take your list (
my_list = [1,2,3,4,5]
). - Create an empty list to store elements in reversed order
(r_list = []
). - Using for loop iterate in the list in reversed order
(for i in range(len(my_list)-1,-1,-1)
). - Inside the loop append ith element in the empty list
(r_list.append(my_list[i])
). - print reversed list (
print(r_list)
).
NOTE: Here you can change the variable name according to you.
Before writing this program few programming concepts you have to know about:
Source Code
my_list = [1,2,3,4,5]
r_list = []
for i in range(len(my_list)-1,-1,-1):
r_list.append(my_list[i])
print(f"Original List: {my_list}")
print(f"Reversed List: {r_list}")
Output
Original List: [1, 2, 3, 4, 5]
Reversed List: [5, 4, 3, 2, 1]
Now let’s modify this program and write it by taking input from the user.
Take input from the user and reverse a list using for loop
Here, we just modify our above program and make it static to dynamic. To make it you should know how to take input from the user.
Source Code
size = int(input("Enter size of the list: "))
my_list = [int(input(f"Enter {i+1} value: ")) for i in range(size)]
r_list = []
for i in range(len(my_list)-1,-1,-1):
r_list.append(my_list[i])
print(f"Original List: {my_list}")
print(f"Reversed List: {r_list}")
Output
Enter size of the list: 5
Enter 1 value: 34
Enter 2 value: 78
Enter 3 value: 12
Enter 4 value: 3
Enter 5 value: 12
Original List: [34, 78, 12, 3, 12]
Reversed List: [12, 3, 12, 78, 34]