In this post, we will learn how to find duplicates from a list in Python with a very simple explanation and algorithms.
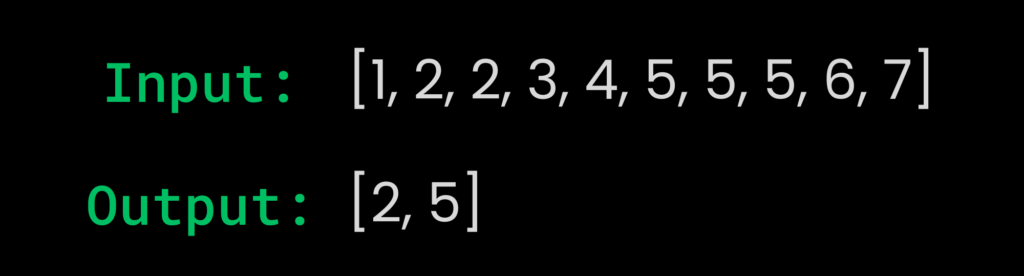
It is a very simple task to find duplicates from the list. Basically, we use the count()
method of the list to count the occurrence of the elements. If the occurrence of the element is greater than one that means it is a duplicate element.
This is how we extract duplicate elements from the list. Let’s see the algorithm first and then write code according to it.
Algorithm to find duplicates from a list
- Create an empty set with the name
store_d
to store duplicate elements of the list. - Using loop iterate in the list (name of this is
my_list
). - Inside the loop check
if my_list.count(i) > 1
then add that element in the empty set (store_d.add(i)
). - Convert the set into the list (
store_d = list(store_d)
). - At the end print the list (
print(store_d)
).
From the algorithm logic and implementation are clear but before writing this program few programming concepts you have to know:
Source Code
my_list = [1,2,2,3,4,5,5,5,6,7]
store_d = set() # instead of list we use set because we want to store every duplicate value only once
for i in my_list:
if my_list.count(i) > 1:
store_d.add(i)
store_d = list(store_d) # convert set into the list
print(f"List : {my_list}")
print(f"Duplicate elements of the list: {store_d}")
Output
List : [1, 2, 2, 3, 4, 5, 5, 5, 6, 7]
Duplicate elements of the list: [2, 5]
We will also write this program in very few lines of code using list comprehension.
Find duplicates from a list in Python Using list comprehension
Few programming concepts you have to know before writing this program:
my_list = [-1,1,-1,3,4,7,4,9,1,8]
duplicate_l = list(set([i for i in my_list if my_list.count(i)>1]))
print(f"List : {my_list}")
print(f"Duplicate elements of the list: {duplicate_l}")
Output:
List : [-1, 1, -1, 3, 4, 7, 4, 9, 1, 8]
Duplicate elements of the list: [1, 4, -1]