In this post, you will learn how to create a simple quiz game program in python with a proper explanation and algorithm.
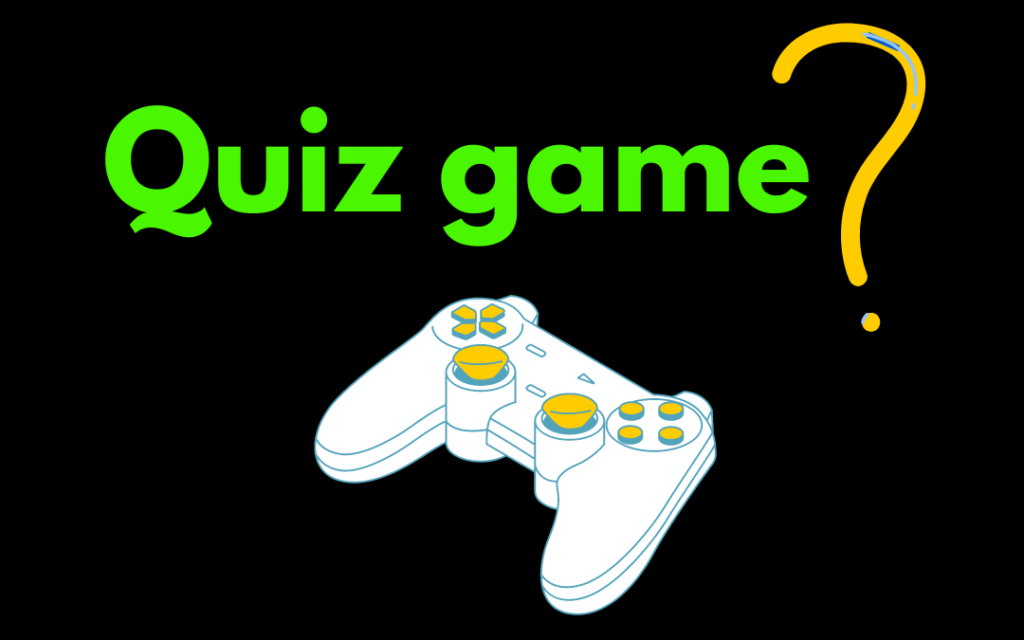
this python program is considered as a mini-project for beginners and also helps them to boost their knowledge and confidence (this project gives you the confidence to learn more and more things) but before writing the program let’s understand the algorithm for a python quiz game.
Algorithm for a Simple python quiz game
- Create one variable for counting score e.g
score
. - Ask questions to a user (using the
input()
function) - if a user gives a correct answer then increase a
score
by 1 andprint(correct!)
- if given answer of a user is incorrect then
print(incorrect!)
- and at last print
score
of the user.
Now you understand how to implement a simple quiz game in python through an above algorithm.
Few programming concepts you have to know for writing this program such as
Source code
question ask like: write a python program to create a quiz game.
print("Wellcome to quiz game !!")
print('NOTE: if your spelling is incorrect then it is considered as wrong answer')
score = 0
question_no = 0
playing = input('Do you want to play ? ').lower()
if playing == 'yes':
question_no += 1
ques = input(f'\n{question_no}. what does CPU stand for? ').lower()
if ques == 'central processing unit':
score +=1
print('correct! you got 1 point')
else:
print('Incorrect!')
print(f'current answer is --> central processing unit')
# ------1
question_no += 1
ques = input(f'\n{question_no}. what does GPU stand for? ').lower()
if ques == 'graphics processing unit':
score +=1
print('correct! you got 1 point')
else:
print('Incorrect!')
print(f'current answer is --> graphics processing unit')
# -----2
question_no += 1
ques = input(f'\n{question_no}. what does RAM stand for? ').lower()
if ques == 'random access memory':
score +=1
print('correct! you got 1 point')
else:
print('Incorrect!')
print(f'current answer is --> random access memory')
# -----3
question_no += 1
ques = input(f'\n{question_no}. what does PSU stand for? ').lower()
if ques == 'power supply unit':
score +=1
print('correct! you got 1 point')
else:
print('Incorrect!')
print(f'current answer is --> power supply unit')
# -----4
question_no += 1
ques = input(f'\n{question_no}. what does ROM stand for? ').lower()
if ques == 'read only memory':
score +=1
print('correct! you got 1 point')
else:
print('Incorrect!')
print(f'current answer is --> read only memory')
# ------5
else:
print('thankyou you are out of a game.')
quit()
print(f'\nnumber of question is {question_no}')
print(f'your score is {score}')
try:
percentage = (score *100)/question_no
except ZeroDivisionError:
print('0% quetions are correct')
print(f'{percentage}% questions are correct.')
Output
Wellcome to quiz game !!
NOTE: if your spelling is incorrect then it is considered as wrong answer
Do you want to play ? yes
1. what does CPU stand for? central processing unit
correct! you got 1 point
2. what does GPU stand for? graphics processing unit
correct! you got 1 point
3. what does RAM stand for? RAM
Incorrect!
current answer is --> random access memory
4. what does PSU stand for? power supplyy unit
Incorrect!
current answer is --> power supply unit
5. what does ROM stand for? read only memory
correct! you got 1 point
number of question is 5
your score is 3
60.0% questions are correct.
Hope this post adds some value to your life – thank you.
Another projects: