In this post, we will write a code to make a hangman game in python but before writing a program let’s understand what is hangman game and how to play it.
What is Hangman game?
Hangman game is a word guessing game where players have to guess a missing word by one letter at a time.
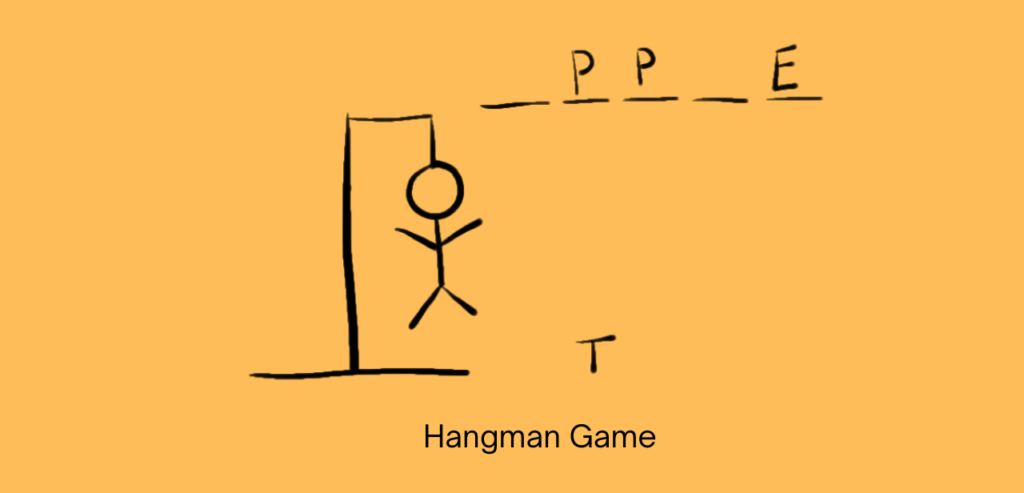
Now we know what is hangman game but before writing code, we should know about programming concepts such as.
- random module
- if-else
- for loop
- while loop
- list or list comprehension
now let’s write a program to create a hangman game in python.
Source code
question make ask like: write a python program to make simple hangman game.
import random
stages = ['''
+---+
| |
|
|
|
|
=========''', '''
+---+
| |
O |
|
|
|
=========''', '''
+---+
| |
O |
| |
|
|
=========''', '''
+---+
| |
O |
/| |
|
|
=========''', '''
+---+
| |
O |
/|\ |
|
|
=========''', '''
+---+
| |
O |
/|\ |
/ |
|
=========''', '''
+---+
| |
O |
/|\ |
/ \ |
|
=========''']
#Word bank of animals
hangman_game = ('ant baboon badger bat bear beaver camel cat clam cobra cougar '
'coyote crow deer dog donkey duck eagle ferret fox frog goat '
'goose hawk lion lizard llama mole monkey moose mouse mule newt '
'otter owl panda parrot pigeon python rabbit ram rat raven '
'rhino salmon seal shark sheep skunk sloth snake spider '
'stork swan tiger toad trout turkey turtle weasel whale wolf '
'wombat zebra ').split()
rendom_choice = random.choice(hangman_game)
blank = ['_' for i in range(len(rendom_choice))]
print(' '.join(blank))
end_game = False
lives = 6
stages = stages[::-1]
while not end_game:
user_choice = input('\nEnter your choice : ')
if len(user_choice) == 0:
print("You are not enter anything pls try again")
else:
if user_choice in blank:
print(f'\nyou already guessed {user_choice}')
for j in range(len(rendom_choice)):
if user_choice in rendom_choice[j]:
blank[j] = user_choice
print(f'\n{" ".join(blank) }\n')
if user_choice not in rendom_choice:
lives-=1
if lives == 0 :
print('You lose.')
print(f'Word is: {rendom_choice}')
end_game = True
print(stages[lives])
if '_' not in blank:
end_game = True
print('You win.')
Output
_ _ _ _
ENter your choice : a
_ a _ _
+---+
| |
|
|
|
|
=========
ENter your choice : e
_ a _ _
+---+
| |
O |
|
|
|
=========
ENter your choice : r
_ a _ _
+---+
| |
O |
| |
|
|
=========
ENter your choice : e
_ a _ _
+---+
| |
O |
/| |
|
|
=========
ENter your choice : t
_ a _ _
+---+
| |
O |
/|\ |
|
|
=========
ENter your choice : s
_ a _ _
+---+
| |
O |
/|\ |
/ |
|
=========
ENter your choice : t
_ a _ _
You lose.
Word is: hawk
+---+
| |
O |
/|\ |
/ \ |
|
=========
Hope this post helped you to create a simple hangman game in python -thank you.