In this post we create number guessing game using python and this is considered as mini project for beginner who just start learning python.
Number guessing game is one of the coolest game and easy to make that’s why every python programmer create this game as there first project in python.
Now it’s time to create our own number-guessing game in Python but before writing a program let us understand how the number-guessing game works.
Number guessing game
The user has to guess a computer-generated random number (this number is not visible to the user) if the user’s guess is correct in a given attempt then the user win otherwise user loses a game.
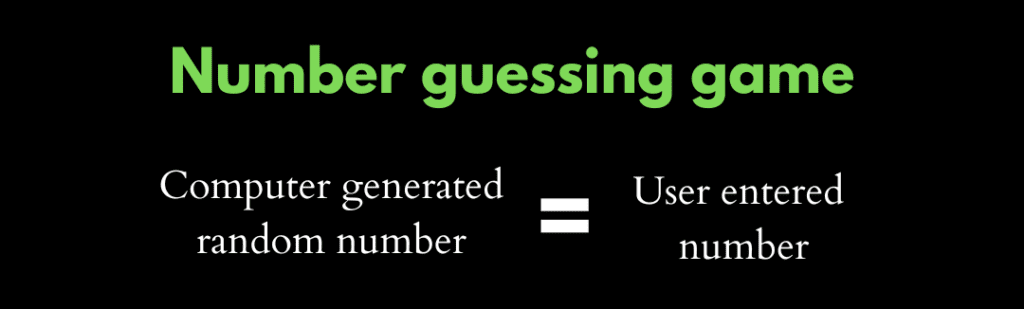
Algorithm for number guessing game
- Computer generate one random number using random module (this number is not visible to user or player).
- user have to guess one number.
- if user guess is equal to computer generated random number than user win.
- if user guess is greater than computer generated random number than you have to print message too High.
- if user guess is less than computer generated random number than you have to print message too low.
- create a counter that count number of attempt.
- repeat step-4 and step-5 until user win.
This 7 steps are compulsory to implements in your code to make number guessing game.
futher you can add levels in this game like hard levels or easy levels in which hard level has 5 attempts to win and easy level has 10 attempts to win.
Here in this game we are add easy and hard levels but before writing code you should know about..
- if-else.
- if-elif-else.
- while loop.
- function.
- random module.
Code for number guessing game
Source code:
import random
def number_gussing_game(user_number,level):
if level == 'Hard':
attemp = 5
else:
attemp = 10
count = 0
computer_guess = random.randint(1,100)
# print(computer_guess)
game_end = False
while not game_end:
if user_number == computer_guess:
print(f"you win in {count+1} attemp")
game_end = True
return True
elif computer_guess>user_number:
print('Too low')
print(f"you have {attemp-1} attemp remain")
attemp-=1
count+=1
elif computer_guess<user_number:
print("too high")
print(f"you have {attemp-1} attemp remain")
attemp-=1
count+=1
if attemp == 0:
print(f"you have {attemp} attemp you lose and guessing number is {computer_guess}")
game_end = True
return False
user_number = int(input('Guess again: '))
print('Wellcome to number guessing game !')
level = input("select defculty level type 'Hard' or 'Easy' : " )
if level == 'Hard':
print('You have only 5 attemp to guess a number \n')
else:
print('You have 10 attemp to guess a number \n')
user_guess = int(input('Guess the number between 1 to 100 :'))
number_gussing_game(user_number=user_guess,level=level)
Output-1:
Wellcome to number guessing game !
select defculty level type 'Hard' or 'Easy' : Hard
You have only 5 attemp to guess a number
Guess the number between 1 to 100 :45
too high
you have 4 attemp remain
Guess again: 35
too high
you have 3 attemp remain
Guess again: 25
too high
you have 2 attemp remain
Guess again: 15
too high
you have 1 attemp remain
Guess again: 5
too high
you have 0 attemp remain
you have 0 attemp you lose and guessing number is 1
Output-2:
Wellcome to number guessing game !
select defculty level type 'Hard' or 'Easy' : Easy
You have 10 attemp to guess a number
Guess the number between 1 to 100 :25
Too low
you have 9 attemp remain
Guess again: 67
too high
you have 8 attemp remain
Guess again: 50
too high
you have 7 attemp remain
Guess again: 35
Too low
you have 6 attemp remain
Guess again: 45
too high
you have 5 attemp remain
Guess again: 37
too high
you have 4 attemp remain
Guess again: 36
you win in 7 attemp
Hope you enjoyed to make this game and learn something new from here -thankyou.