Find the area of square is a basic problem that helps beginners to understand concepts of Python and it also helps to improve their logic.
In this post, we will learn how to find the area of a square in Python by taking input from the user and also writing it using the function but before writing a program let’s see the formula to find area of a square.
Formula for Area of Square
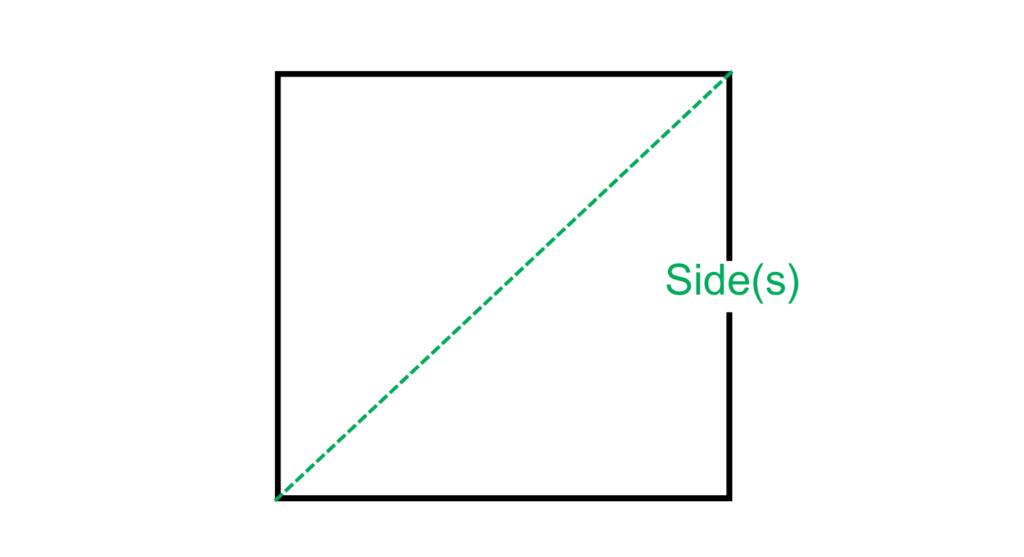
Formula: A = s2
Where A = Area of a Square.
s = length of the side
Algorithm for Area of Square
- Ask the user to enter the length of the side (
side
) - Create one variable called
Area
and perform the calculationArea = side**2
. - At the end print the result (
Area
).
From the above algorithm, we can easily write a Python program to find the area of a square but before writing a program few programming concepts you have to know:
Source Code
side = int(input('Enter Length of the Side: '))
Area = side**2
print(f'Area of a Square is {Area}')
Output
Enter Length of the Side: 5
Area of a Square is 25
Now let’s write this program using Python Function.
Find the Area of square in Python Using Function
Before writing this program you should know about:
Source Code
def Area_of_square(s):
return s**2
side = int(input('Enter Length of the Side: '))
print(f'Area of a Square is {Area_of_square(side)}')
Output
Enter Length of the Side: 7
Area of a Square is 49