In this post, we will learn about the Python zip()
function in detail with an example. after reading this post you will know everything about the Python zip()
function. So let’s start learning from the very basic what is zip()
function.
zip()
Function
zip()
is a built-in function that will take two or more iterables as input and returns an iterator of tuples. each tuple contains corresponding elements from the input iterables.
Syntax: zip(iterable1, iterable2, ...)
For Example:
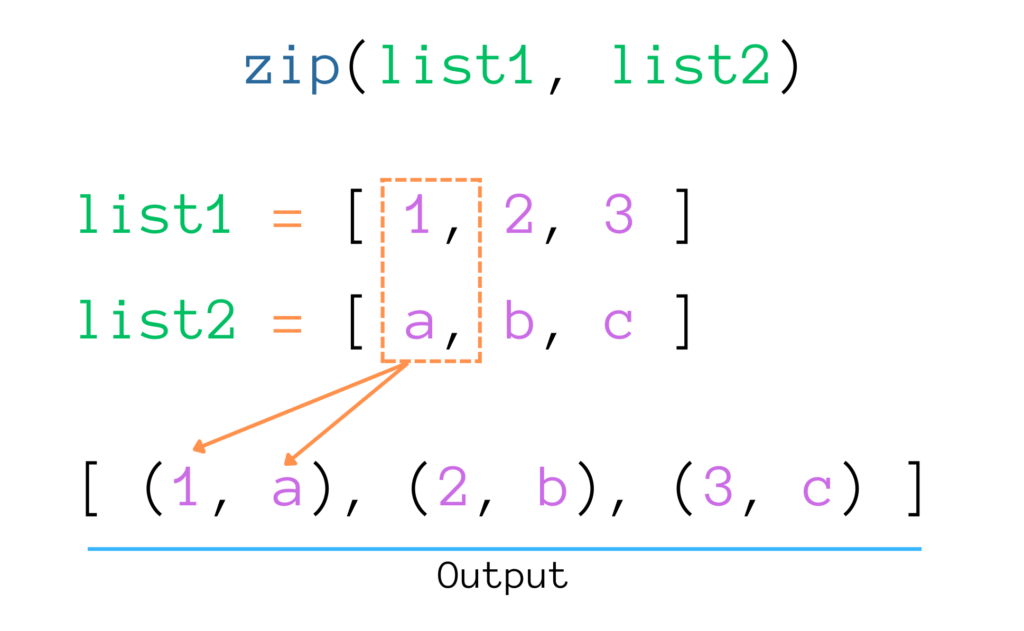
Let’s understand it in a better way by doing it practically.
list1 = [1,2,3]
list2 = ["a","b","c"]
z = list(zip(list1,list2))
print(z)
Output:
[(1, 'a'), (2, 'b'), (3, 'c')]
Let’s pass three iterables to the zip() function and see the output.
list1 = [1,2,3]
list2 = ["a","b","c"]
list3 = ["$","%","@"]
zipped = list(zip(list1,list2,list3))
print(zipped)
Output:
[(1, 'a', '$'), (2, 'b', '%'), (3, 'c', '@')]
Example for Practice
We can easily map the data or manipulate the data using the zip()
function. Here few practical examples that will help you understand how zip()
works.
Example-1: map the countries and their capitals using the zip() function.
contries = ["India", "USA", "UK","Russia"]
capitals = ["New Delhi", "Washington, D.C.", "London", "Moscow"]
mapping = list(zip(contries,capitals))
print(mapping)
Output:
[('India', 'New Delhi'), ('USA', 'Washington, D.C.'), ('UK', 'London'), ('Russia', 'Moscow')]
Example-2: Transpose of a matrix using the zip() function.
matrix_A = [
[1,2,3],
[4,5,6],
[7,8,9]
]
transpose = []
for rows in zip(*matrix_A):
transpose.append(list(rows))
print(transpose)
Output:
[[1, 4, 7], [2, 5, 8], [3, 6, 9]]
zip()
function with tuple
num = (1,2,3,4,5)
square = (1,4,9,16,25)
mapped = tuple(zip(num,square))
print(mapped)
Output:
((1, 1), (2, 4), (3, 9), (4, 16), (5, 25))
Unzipping Using zip()
function
contries = ["India", "USA", "UK","Russia"]
capitals = ["New Delhi", "Washington, D.C.", "London", "Moscow"]
# zip
result = list(zip(contries,capitals))
# unzipping (we use '*' opertor to unzip the iterables)
con, cap = zip(*result)
print(f"List of Countries: {con}")
print(f"List of capitals: {cap}")
Output:
List of Countries: ('India', 'USA', 'UK', 'Russia')
List of capitals: ('New Delhi', 'Washington, D.C.', 'London', 'Moscow')