In this post, we will learn how to remove background from images in Python with a very simple explanation and algorithm.
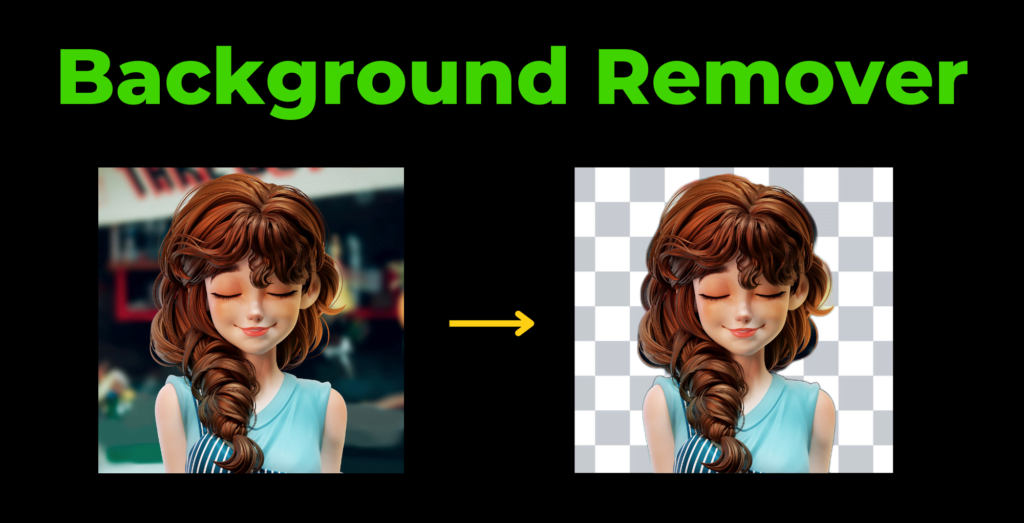
You can consider this Python program as your mini-project. Trust me, it is a super cool project where you can make your own tool to remove background from images.
To create background remover using Python you need to know and install three external libraries of Python and they are as follows.
1. Pillow Library: This external Python library help us to do image manipulation. With the help of this library, you can perform various image operations such as opening, resizing, adding sharpness, adjusting brightness and contrast, and more.
To install this library, open your command prompt and run this command:
pip install pillow
2. Rembg Library: This external Python library helps to remove the background from the images.
This is the main library that we are using for this project. To install this library open your command prompt and run this command:
pip install rembg
3. Easygui Library: This external Python library provides GUI (Graphical User Interface).
To install this library open your command prompt and run this command:
pip install easygui
After installing all the libraries you need to follow the algorithm (steps) to create background remover in Python.
Algorithm to Remove Background From Images
- Import
remove()
function fromrembg
library.
(from rembg import remove
) - Import
Image
from pillow library.
(from PIL import Image
) - Import Easygui library (
import easygui
) - Using the
easygui
library’s function calledfileopenbox()
, create a pop-up window to select an image from a specific file or folder and store it in a variable called ‘input_path
‘. - Similarly, using the
easygui
library’s function calledfilesavebox()
, create a
pop-up window to specify where you want to save your image in .png format and store that address in a variable called ‘Output_path
‘. - After that pass
input_path
toImage.open()
function and store it in a variable called ‘my_img
‘. - Using the
remove()
function remove the background from the image and store it in a variable called ‘rem
‘. (rem = remove(my_img)
) - At the end save your background removed image.
(save = rem.save(Output_path)
)
Source Code
from rembg import remove
from PIL import Image # pillow
import easygui
print("Please Wait...")
input_path = easygui.fileopenbox("Select your Image")
Output_path = easygui.filesavebox("Where you want to save your Image")
my_img = Image.open(input_path)
print("Please Wait...")
rem = remove(my_img)
save = rem.save(Output_path)
print("Successfully Done, check your Folder")
Output
After executing the above source code in your code editor, a pop-up window will appear, allowing you to select the image you want to remove the background from.
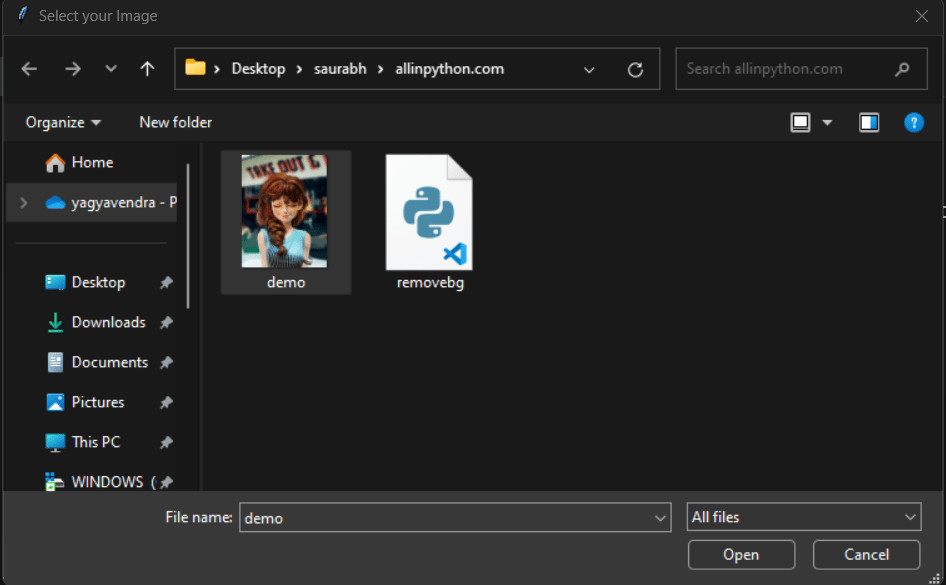
After selecting an image from your file or folder you will get another pop-up to specify the location, where you want to save the new image in .png format.
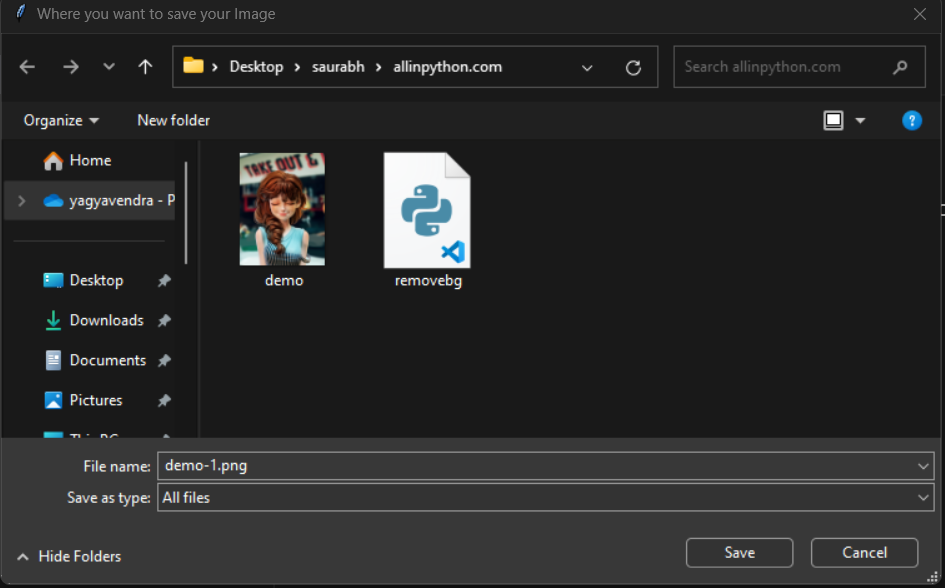
After that, you will get a successful message in your console and the background is remove from your image (you will see the output in your specified folder).
Please Wait...
Please Wait...
Successfully Done, check your Folder
For more Python projects Click Here