In this post, you will learn a Python Program to Check Even or Odd Numbers with detailed explanation and algorithm but before we dive into the coding part, let us first understand what is an even and odd number.
What is an Even and Odd number?
A number that is divisible by 2 is known as an Even number whereas a number that is not divisible by 2 is known as an Odd number.
For Example: 2, 4, 6, 8, 10 are even numbers, while 1, 3, 5, 7, 9 are odd numbers.
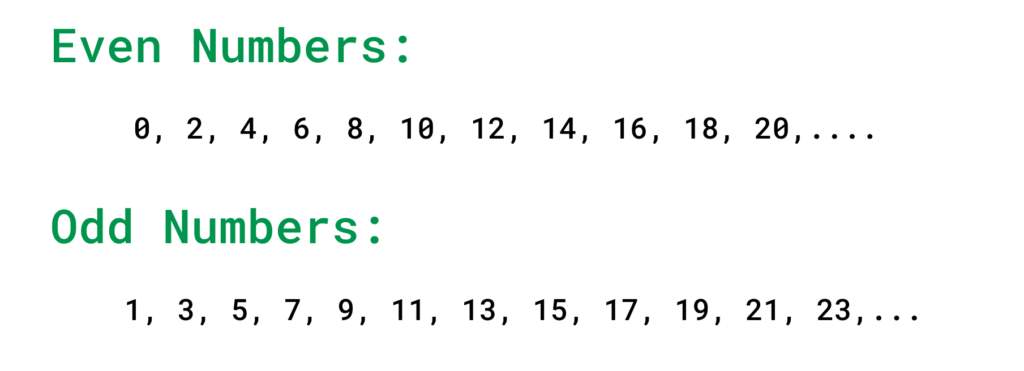
Algorithm to Check Even or Odd Number
- Take the input from the User (
num
) - check
if num % 2 == 0
then printnum
is Even. else
printnum
is Odd.
These three basic steps you have to follow to write a python program to check even or odd numbers.
But before writing this program few programming concepts you have to know:
Source Code
num = int(input("Enter a number to check Odd and Even: "))
if num % 2 == 0:
print(f"{num} is Even Number")
else:
print(f"{num} is Odd Number")
Output-1
Enter a number to check Odd and Even: 9 9 is Odd Number |
Output-2
Enter a number to check Odd and Even: 8 8 is Even Number |
Let us modify the above program and write it using the function.
Python Program to Check Even or Odd Number Using the function
Before writing this program few programming concepts you have to know:
Source Code
def isEven(num):
if num %2 == 0:
return f"{num} is Even number"
else:
return f"{num} is Odd number"
number = int(input("Enter a number to check Odd and Even: "))
print(isEven(number))
Output-1
Enter a number to check Odd and Even: 15 15 is Odd number |
Output-2
Enter a number to check Odd and Even: 12 12 is Even number |