In this post, we will learn number system conversion in Python using built-in functions with detailed explanations and examples.
There are many built-in functions in Python that help us convert decimal numbers to binary, octal, and hexadecimal numbers, or vice-versa. Let’s learn each of these functions with the help of examples.
bin() Function
The bin()
function is used to convert decimal integers into their respective binary representation.
num = int(input("Enter a number: "))
binary = bin(num)
print(f"Binary of {num} is : {binary}")
Output:
Enter a number: 10
Binary of 10 is : 0b1010
The first two characters 0b
represent that it is a binary number and the remaining character 1010
is the actual binary representation of the given number.
oct() function
The oct()
function is used to convert decimal integers into their respective octal representation.
num = int(input("Enter a number: "))
octal = oct(num)
print(f"Octal of {num} is : {octal}")
Output:
Enter a number: 10
Octal of 10 is : 0o12
As we already discussed, the first two characters 0o
represent that it is an octal number, and the remaining 12
is the actual octal representation of given number.
hex() function
The hex()
function is used to convert decimal integers into their respective hexadecimal representations.
num = int(input("Enter a number: "))
hexadeciaml = hex(num)
print(f"Hexadeciaml of {num} is : {hexadeciaml}")
Output:
Enter a number: 10
Hexadeciaml of 10 is : 0xa
The first two characters 0x
represent that it is a hexadecimal number and the remaining character a
is the actual hexadecimal representation of the given number.
Now let’s discuss how we can convert any binary, octal, and hexadecimal number into their respective decimal numbers.
Binary to Decimal Number
We use the int()
function to convert any binary number into its respective decimal number. There is one attribute of the int()
function called base
that helps us convert any number system into its respective decimal number.
If you want to convert a binary number into a decimal number, you can achieve this by simply changing the base=2
in the int()
function by default base
is set to 10. But remember one thing You have to pass a binary number or another number system such as an octal or hexadecimal number in the form of a string otherwise you will get an error.
binary = "10"
decimal = int(binary,base=2)
print(f"Decimal of binary number {binary} is: {decimal}")
Output:
Decimal of binary number 10 is: 2
Octal to Decimal Number
To convert an octal number to its respective decimal representation, pass the octal number as a string to the int()
function and set the base
to 8.
octal = "12"
decimal = int(octal,base=8)
print(f"Decimal of octal number {octal} is: {decimal}")
Output:
Decimal of octal number 12 is: 10
Hexadecimal to Decimal Number
To convert a hexadecimal number to its respective decimal representation, pass the hexadecimal number as a string to the int()
function and set the base
to 16.
hexadecimal = "a"
decimal = int(hexadecimal,base=16)
print(f"Decimal of hexadecimal number {hexadecimal} is: {decimal}")
Output:
Decimal of hexadecimal number a is: 10
Summary
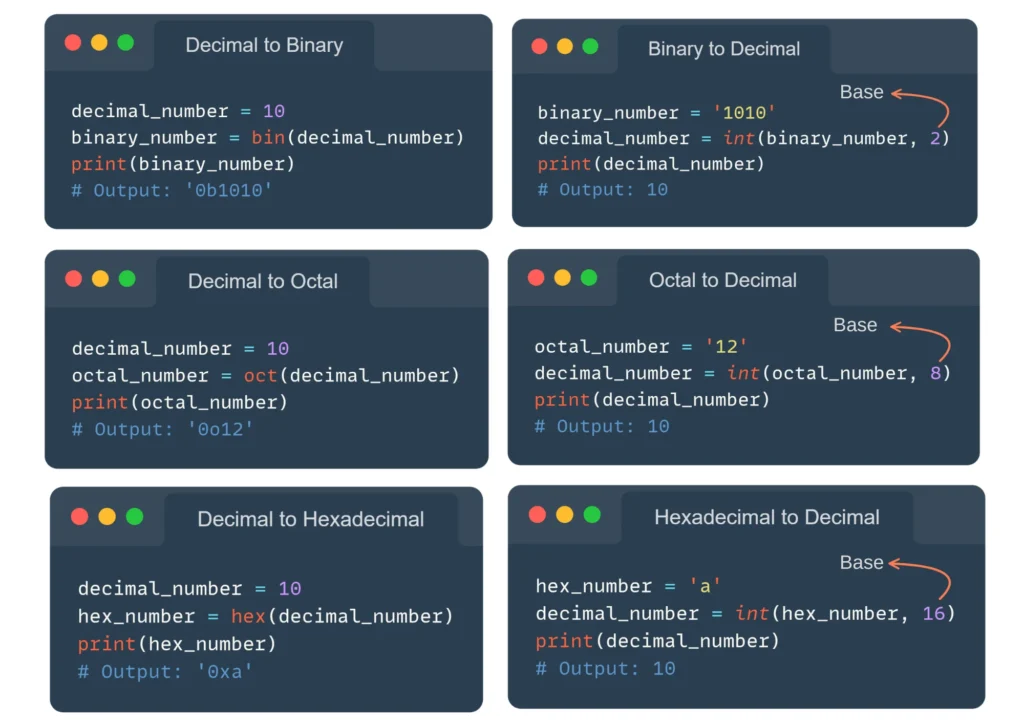
For more Python programs click here 👉 60+ Python programs with an explanation