In this post, we learn how to write a python program to check for Armstrong numbers with a detailed explanation but before writing the program let’s understand what is Armstrong number.
What is Armstrong number?
Armstrong number is a number in which the sum of each digit powered to the total number of digits is same as the given number.
For example: 153
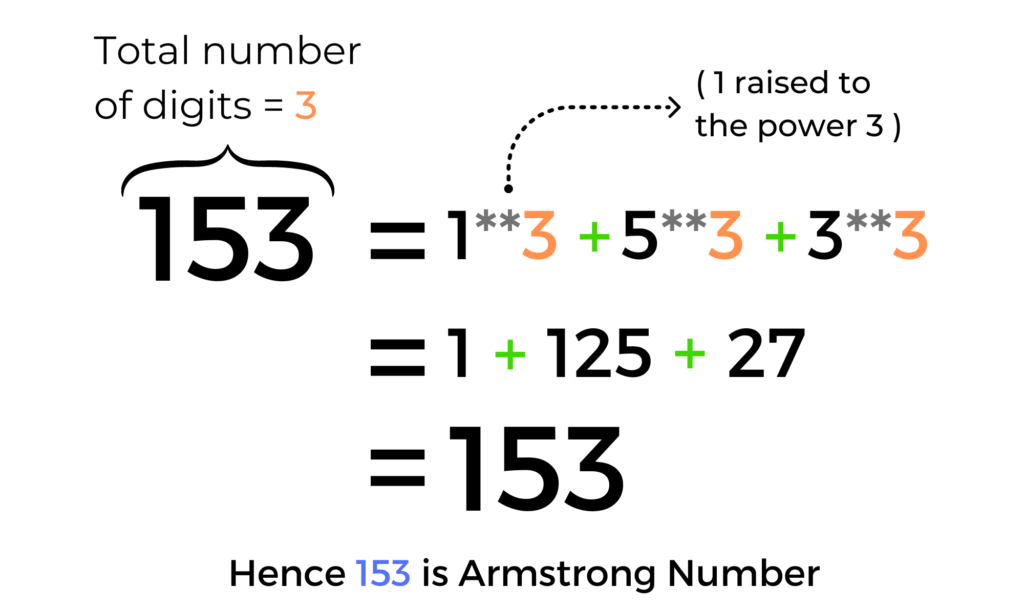
Another example: 1634
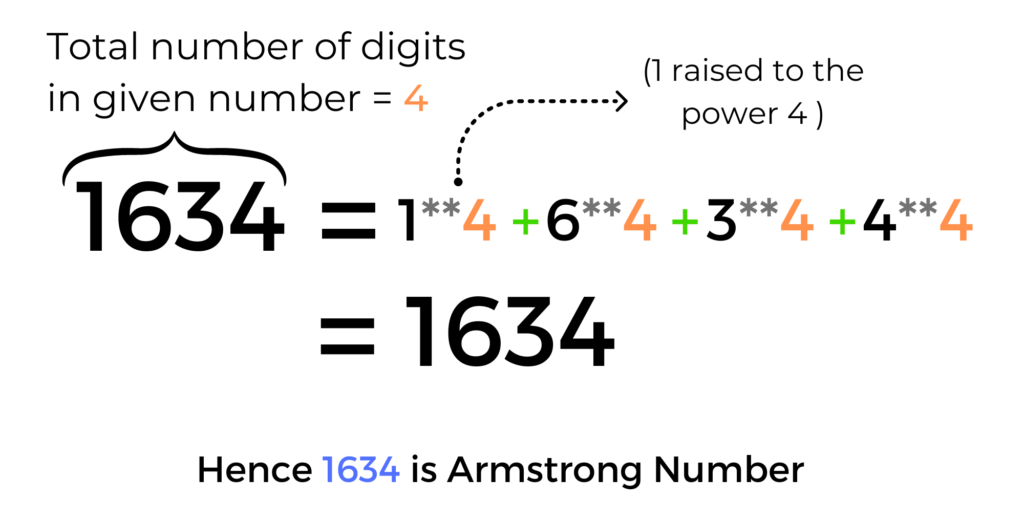
Armstrong number program in python
We are writing this program in three different ways
- Simple by taking user input
- By using function
- using try-except block (Except the exception, if the user inputs wrong data)
Simple by taking user input
Questions may be asked like: Write a python program to check for Armstrong number by taking user input.
But before writing this program you should know about:
Source code
my_num = int(input('ENter a number: '))
power_of_my_num = len(str(my_num))
total = 0
i = my_num
while i > 0:
remainder = i%10
total += remainder**power_of_my_num
i = i // 10
if total == my_num:
print(f'{my_num} is Armstrong number')
else:
print(f'{my_num} is not Armstrong number')
Output-1
ENter a number: 153
153 is Armstrong number
Output-2
ENter a number: 1635
1635 is not Armstrong number
By using function
Questions may be asked like: Write a python program to check for Armstrong number by using a user-defined function.
But before we jump into the program few things you should know about:
Source code
def Armstrong_checker(num):
power_of_num = len(str(num))
sum = 0
i = num
while i>0:
remainder = i%10
sum += remainder**power_of_num
i = i//10
if sum == num:
return True
else:
return False
my_number = int(input('ENter a number: '))
if Armstrong_checker(my_number):
print(f'{my_number} is Armstrong number')
else:
print(f'{my_number} is not Armstrong number')
Output-1:
ENter a number: 1634
1634 is Armstrong number
Output-2:
ENter a number: 145
145 is not Armstrong number
Using try-except block (Except the exception, if the user inputs wrong data)
Questions may be asked like: Write a python program to check for Armstrong number and except the exception, if the user inputs wrong data.
But before we jump into the program few things you should know about:
Source code
def Armstrong_checker(num):
power_of_num = len(str(num))
sum = 0
i = num
while i>0:
remainder = i%10
sum += remainder**power_of_num
i = i//10
if sum == num:
return True
else:
return False
while True:
try:
my_number = int(input('ENter a number: '))
break
except Exception:
print('only numbers are allowed pls enter again')
if Armstrong_checker(my_number):
print(f'{my_number} is Armstrong number')
else:
print(f'{my_number} is not Armstrong number')
Output-1:
ENter a number: one
only numbers are allowed pls enter again
ENter a number: 1
1 is Armstrong number
Output-2:
ENter a number: 1634
1634 is Armstrong number