Type Casting is one of the important features of Python and you should definitely know about it. So in this article, we will learn what is Type Casting in Python and the types of Type Casting in Python with detailed explanations and examples.
So let’s start learning from the very basics…
What is Type Casting in Python
Type Casting is the process to convert one data type into another data type. For example, convert the int
data type into a float
by using the float()
function.
This means you can type cast int
into a float
.
my_int = 15 # int
my_float = float(my_int) # coverte int to float
print(my_float)
#Output: 15.0
Various built-in functions in Python that are used to convert one data type into another data type, here is a list of a few built-in functions.
int() | float() | str() |
list() | tuple() | dict() |
set() |
NOTE: List, tuple, dictionary, set, etc are built-in data structures in Python which means you can also convert or type cast one data structure into another data structure using the appropriate built-in functions.
Also remember, you can not convert int
to list
or float
to list
because int
and float
are not iterable.
Types of type Casting in Python
There are two types of type casting in Python.
- Implicit Type Casting
- Explicit Type Casting
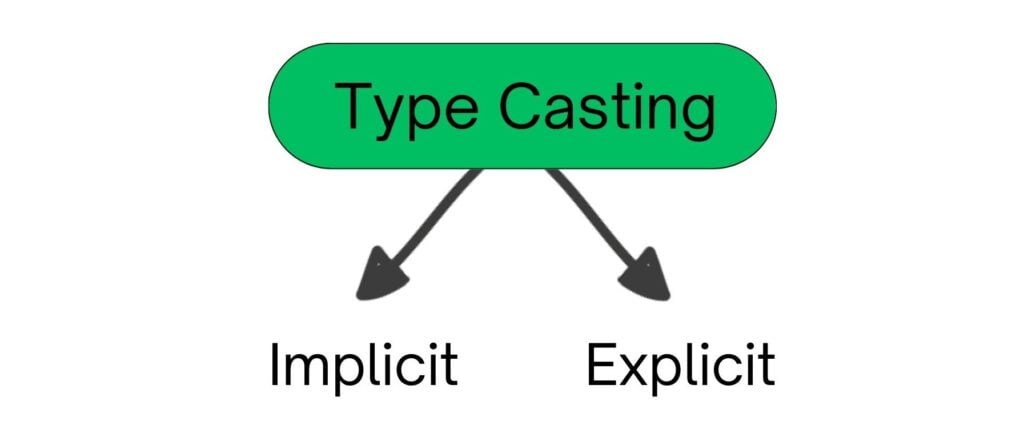
Implicit Type Casting
In implicit type casting Python automatically converts one data type into another data type whenever it is required.
For example, when we perform the addition of int
with a float
number, Python automatically promotes int
data type into a float
to get the correct output.
a = 15 # int
b = 17.5 # float
c = a+b # result is also in float
print(c)
Output:
32.5
Explicit Type Casting
In explicit type casting, the user (programmer) intentionally converts one data type into another data type according to their requirements.
One of the best examples of explicit type casting is when we take integer input from the user. Since the input()
function receives input as a string, we need to use the int()
function to explicitly convert the string to an integer data type. This way, we can use the integer value in our code.
num = input("Enter a number: ")
print(type(num))
# Explicit Type Casting
num = int(num)
print(type(num))
Output:
<class 'str'>
<class 'int'>
Type Casting int to float
We can use the float()
function to convert or typecast an integer to a floating-point number in Python.
num = 24 # integer
print(num)
print(type(num))
# typecast int to float
fnum = float(num)
print(fnum)
print(type(fnum))
# # ************** OR ******************
# # you can also typecast float to int using int() function
# a = 5.7
# b = int(a)
# print(b)
# print(type(b))
Output:
24
<class 'int'>
24.0
<class 'float'>
Type Casting str to int
We can use the int()
function to convert or typecast a string to an integer number in Python.
my_str = "15" # string
print(my_str)
print(type(my_str))
# typecast str to int
my_num = int(my_str)
print(my_num)
print(type(my_num))
# # ************** OR ******************
# # you can also typecast int to str using str() function
# a = 5
# b = str(a)
# print(b)
# print(type(b))
Output:
15
<class 'str'>
15
<class 'int'>
Similarly, you can also convert or typecast any Python data structure (such as list, tuple, set, etc.) into another Python data structure using the appropriate built-in function.