In this post, we will learn what is string formatting in python, why we use it, and how we can format our strings, with a detailed explanation and examples. So, let’s start with the very basics.
What is String Formatting?
String formatting is a way to combine your string and variable together to make a sentence that effectively describes your message to the user.
For better understanding, let’s take an example: Suppose you have two variables, name = "Joy"
and age = 22
, now you want to display the message “My name is Joy and I am 22 years old
“. there is one way you can use string concatenation to display this message.
name = "Joy"
age = 22
print("My name is " + name + " and I am " + str(age) +" years old")
Output:
My name is Joy and I am 22 years old
As you can see, it is a very tedious job to combine your custom string and variable together to create a sentence using string concatenation. You have to concatenate multiple strings and also typecast variables into strings if they contain numeric data. To avoid all such problems, there is one string method called the .format()
method that helps us format our string more easily compared to string concatenation.
So let’s see how the .format()
method works.
format() method
.format()
is a string method that helps us format our string Using curly braces ({}
) as a placeholder. Let’s understand its syntax with the help of an example.
Syntax:
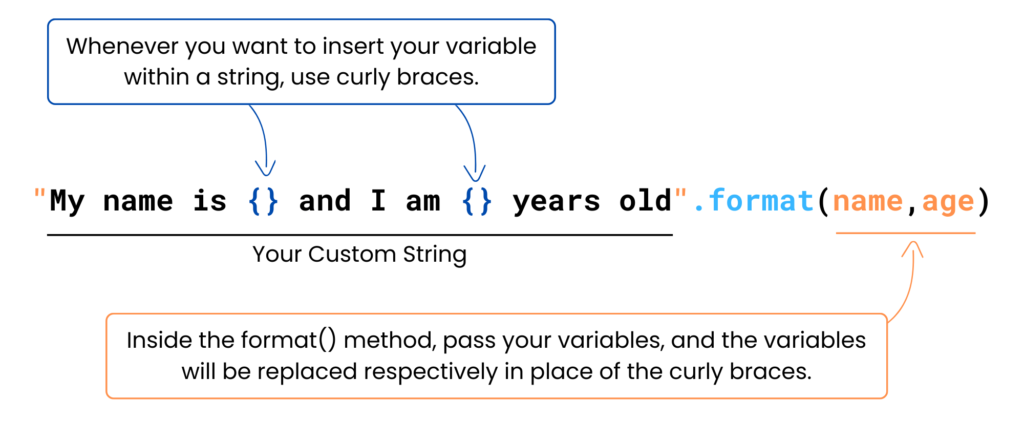
Example:
name = "Joy"
age = 22
print("My name is {} and I am {} years old".format(name,age))
Output:
My name is Joy and I am 22 years old
The .format()
method is very helpful for formatting a string. However, it has one drawback—it is not very readable. That’s why in Python 3.6, a new way to format strings was introduced, known as f-strings.
Nowadays, f-strings are commonly used for formatting strings instead of the .format()
method. So let’s see how we can use f-string to format our strings.
Use f-string for formatting a string
Syntax:
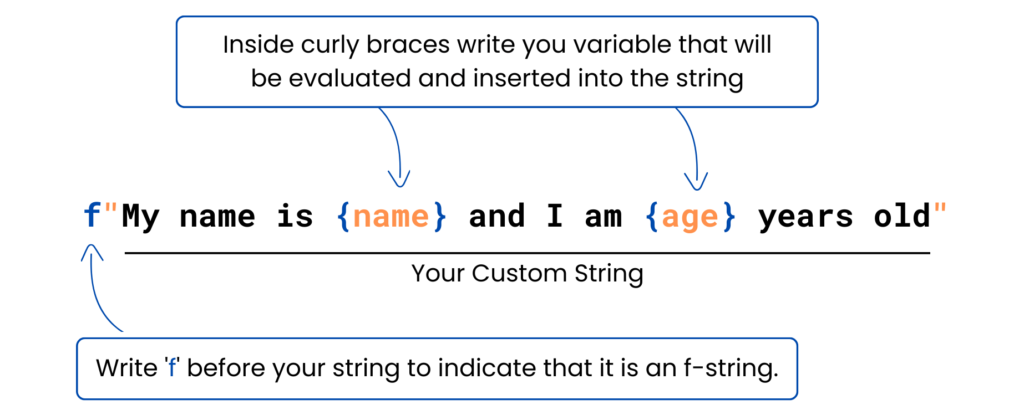
Example:
name = "Joy"
age = 22
print(f"My name is {name} and I am {age} years old")
Output:
My name is Joy and I am 22 years old
It is the recommended way to use f-strings for formatting a string because they offer better readability, a simple syntax, and ease of use.
I hope you understood what string formatting is and why we use it. I explained all the important things related to string formatting. So if you find this article helpful, then share it with your friends.
👉You can also check out: 60+ Python Practicals for your practice