In this post, you will learn a python program to rotate a matrix by 90 degrees in clockwise directions with a very simple explanation and algorithm.
So let us start learning…
Rotate a Matrix by 90 Degrees (Clockwise)
Rotating a matrix by 90 degrees in the clockwise direction using python is a very simple task. You just have to do two simple steps, the first step is to transpose the given matrix and the second step is to reverse the rows of the transpose matrix.
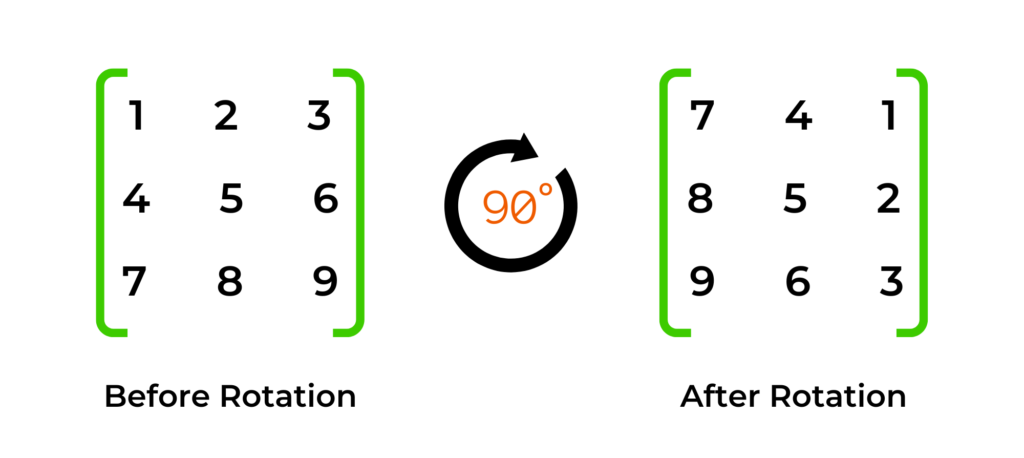
Now let’s see the algorithm for the above steps
Algorithm
1. | Define a function rotate_matrix(matrix) that takes a matrix as an input. |
2. | Transpose the matrix using the zip function:-> Create an empty list called transposed .-> Iterate over the rows of the matrix (using zip(*matrix) ).-> For each row, append a list version of the row to transposed . |
3. | Reverse the rows of the transposed matrix: -> Create an empty list called rotated .-> Iterate over the rows of the transposed matrix.-> For each row, append the reversed row to rotated . |
4. | Return the rotated matrix. |
From the above algorithm, we understood how to implement the python program to rotate a matrix by 90 degrees in a clockwise direction.
But before writing a program few programming concepts you have to know:
Source Code
def rotate_matrix(matrix):
transposed = []
for row in zip(*matrix):
transposed.append(list(row))
rotated = []
for row in transposed:
rotated.append(row[::-1])
return rotated
# User input
rows = int(input('Enter number of rows: '))
cols = int(input('Enter number of column: '))
matrix = [[int(input(f"column {j+1} -> ENter {i+1} element:")) for j in range(cols)] for i in range(rows) ]
print() # for new line
print('Given matrix :')
# print matrix
for row in matrix:
print(row)
# calling a function
rotate_90 = rotate_matrix(matrix)
print() # for new line
print('Matrix after rotated by 90 degree')
# print rotated matrix
for row in rotate_90:
print(row)
Output-1
Enter number of rows: 3 Enter number of column: 3 column 1 -> ENter 1 element:1 column 2 -> ENter 1 element:2 column 3 -> ENter 1 element:3 column 1 -> ENter 2 element:4 column 2 -> ENter 2 element:5 column 3 -> ENter 2 element:6 column 1 -> ENter 3 element:7 column 2 -> ENter 3 element:8 column 3 -> ENter 3 element:9 Given matrix : [1, 2, 3] [4, 5, 6] [7, 8, 9] Matrix after rotated by 90 degree [7, 4, 1] [8, 5, 2] [9, 6, 3] |
Output-2
Enter number of rows: 2 Enter number of column: 2 column 1 -> ENter 1 element:1 column 2 -> ENter 1 element:2 column 1 -> ENter 2 element:3 column 2 -> ENter 2 element:4 Given matrix : [1, 2] [3, 4] Matrix after rotated by 90 degree [3, 1] [4, 2] |