In this post, you will learn how to write a python program to remove duplicates from the list with an Explanation.
There are many ways to remove duplicates from the list but in this post, we will learn the three best and easy ways to do it.
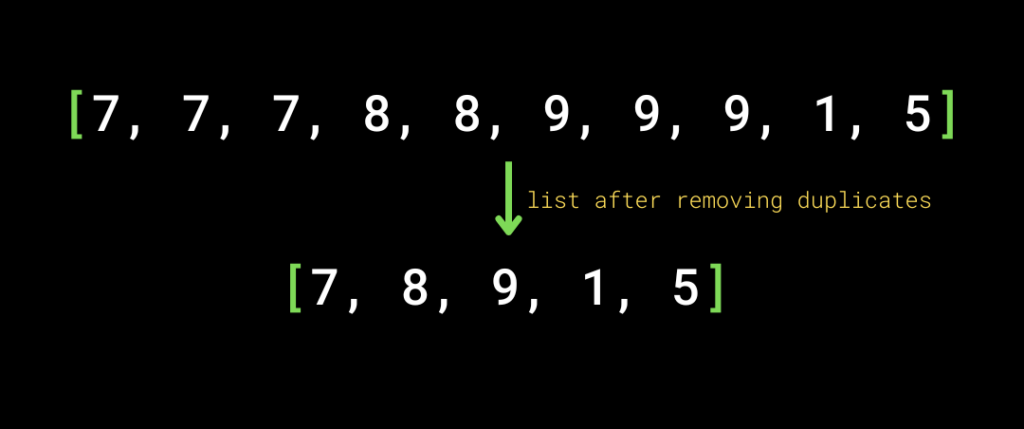
Three ways to remove duplicates from the list and those are:
Python Program to remove duplicates from the list using for loop
Few programming concepts you have to know before writing this program such as
- for loop &
- python list
- list comprehension (if you want to take input from the user)
Source code
size_of_list = int(input('ENter size of the list: '))
# create a list taking input from user
my_list = [int(input(f'ENter {i+1}th element of the list: ')) for i in range(size_of_list)]
new_list = [] #store unique elements
# main logic
for i in my_list:
if i not in new_list:
new_list.append(i)
print(f'your list {my_list}')
print(f'list after removing duplicates {new_list}')
Output
ENter size of the list: 5
ENter 1th element of the list: 4
ENter 2th element of the list: 5
ENter 3th element of the list: 45
ENter 4th element of the list: 4
ENter 5th element of the list: 5
your list [4, 5, 45, 4, 5]
list after removing duplicates [4, 5, 45]
Explanation
The main logic of the above program is that create a new empty list (new_list
) and check if the ith element of your list (my_list
) is not present inside the empty list then append the ith element in the empty list (new_list
).
This is how we filter unique elements from the list using for loop.
Python Program to remove duplicates from the list using a set() function
Few programming concepts you have to know before writing this program such as
- set() function
- list comprehension (if you want to take input from the user)
Source code
my_list = [1,1,1,2,2,2,3,3,3,5]
print(f'your list {my_list}')
# steps to remove dupicates from the list using set() function
# 1-> converts given list into set
# 2-> after that coverts into list again
my_list = list(set(my_list))
print(f'list after removing duplicates {my_list}')
Output
your list [1, 1, 1, 2, 2, 2, 3, 3, 3, 5]
list after removing duplicates [1, 2, 3, 5]
Explanation
As you know the set is an unordered collection of unique items, So when you convert the list into the set then automatically duplicate elements are removed and after removing duplicates we convert it back into the list.
This is how we filter unique elements from the list using the set() function.
Python Program to remove duplicates from the list using a list comprehension
Few programming concepts you have to know before writing this program such as
Source code
my_list = [7,7,7,8,8,9,9,9,1,5]
print(f'your list {my_list}')
new_list = [my_list[i] for i in range(len(my_list)) if i == my_list.index(my_list[i])]
print(f'list after removing duplicates {new_list}')
Output
your list [7, 7, 7, 8, 8, 9, 9, 9, 1, 5]
list after removing duplicates [7, 8, 9, 1, 5]
Explanation
The main logic of the above program is to find the index of the current value and check if it is equal to the ith value (if i == my_list.index(my_list[i])
) then append it (my_list[i]
)into the list.