In this post, we will learn how to write a Python program to print hollow square patterns using for-loop, while-loop, using a function, etc.
So let’s start writing a program to print hollow square patterns in python…
1. Python Program to Print Hollow Square Pattern Using for-loop
Before writing a program few program concepts you have to know:
Source Code:
size = int(input('Enter Size of the hollow Square: '))
for i in range(size):
for j in range(size):
if i == 0 or i == size-1 or j == 0 or j == size-1:
print("*", end=" ")
else:
print(" ", end=" ")
print("\r")
Output:
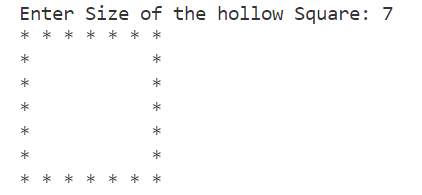
2. Python Program to Print Hollow Square Pattern Using while-loop
Before writing this program you should know about:
Source Code:
size = int(input('Enter Size of the hollow Square: '))
i = 0
while i<size:
j = 0
while j<size:
if i == 0 or i == size-1 or j == 0 or j == size-1:
print("*", end=" ")
else:
print(" ", end=" ")
j+=1
print("\r")
i+=1
Output:
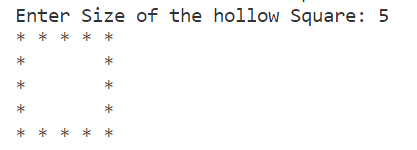
3. Python Program to Print Hollow Square Pattern Using function
Before writing this program you should know about:
Source Code:
def hollow_square(size,shape):
for i in range(size):
for j in range(size):
if i == 0 or i == size-1 or j == 0 or j == size-1:
print(shape, end=" ")
else:
print(" ", end=" ")
print("\r")
size = int(input('Enter Size of the hollow Square: '))
shape = input('Enter character: ')
hollow_square(size,shape)
Output-1:
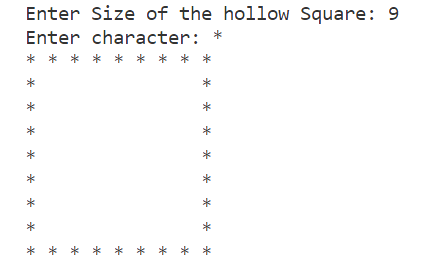
Output-2:
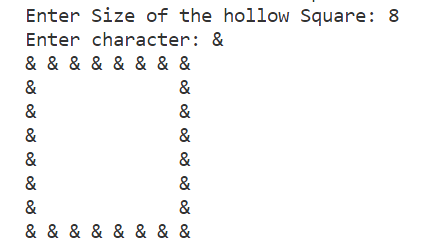