In this post, we will write a Python program to display calendar along with a detailed explanation and example.
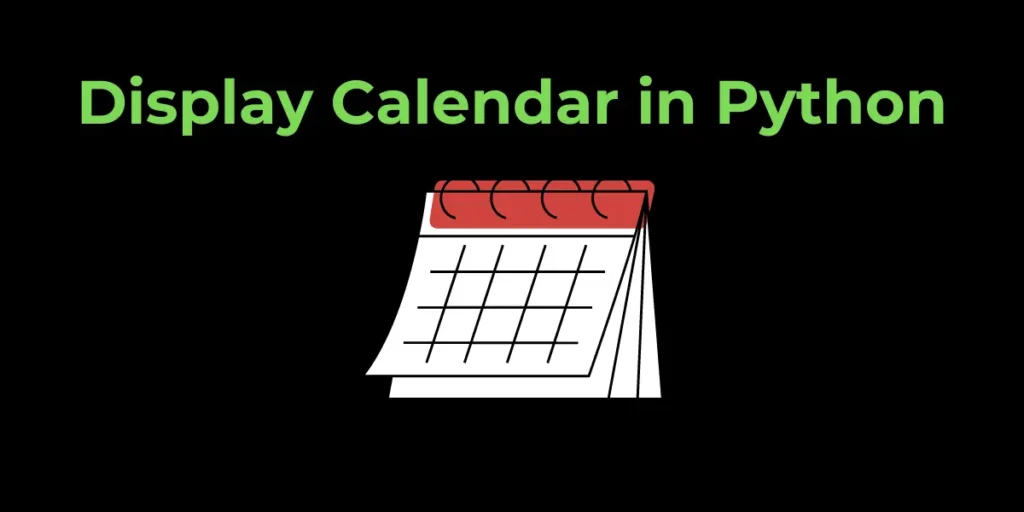
Python provides a built-in module named a calendar
. By using this module we will easily print the calendar of the whole year or a specific month. So let’s see the steps on how we can print a calendar using the calendar
module.
Steps to Display Calendar in Python
- Import
calendar
module. - Ask the user to input a
year
in integer format. - Using
calendar.calendar()
function print specified year.print(calendar.calendar(year))
. - End the program.
From the above algorithm, now we know how we will display a calendar in Python but before we jump into the programming part few programming concepts you have to know:
- Basic of Python modules
- How to take input from the user
Source Code
import calendar
year = int(input("Enter a year: "))
# display the calendar
print(calendar.calendar(year))
Output
Enter a year: 2024
2024
January February March
Mo Tu We Th Fr Sa Su Mo Tu We Th Fr Sa Su Mo Tu We Th Fr Sa Su
1 2 3 4 5 6 7 1 2 3 4 1 2 3
8 9 10 11 12 13 14 5 6 7 8 9 10 11 4 5 6 7 8 9 10
15 16 17 18 19 20 21 12 13 14 15 16 17 18 11 12 13 14 15 16 17
22 23 24 25 26 27 28 19 20 21 22 23 24 25 18 19 20 21 22 23 24
29 30 31 26 27 28 29 25 26 27 28 29 30 31
April May June
Mo Tu We Th Fr Sa Su Mo Tu We Th Fr Sa Su Mo Tu We Th Fr Sa Su
1 2 3 4 5 6 7 1 2 3 4 5 1 2
8 9 10 11 12 13 14 6 7 8 9 10 11 12 3 4 5 6 7 8 9
15 16 17 18 19 20 21 13 14 15 16 17 18 19 10 11 12 13 14 15 16
22 23 24 25 26 27 28 20 21 22 23 24 25 26 17 18 19 20 21 22 23
29 30 27 28 29 30 31 24 25 26 27 28 29 30
July August September
Mo Tu We Th Fr Sa Su Mo Tu We Th Fr Sa Su Mo Tu We Th Fr Sa Su
1 2 3 4 5 6 7 1 2 3 4 1
8 9 10 11 12 13 14 5 6 7 8 9 10 11 2 3 4 5 6 7 8
15 16 17 18 19 20 21 12 13 14 15 16 17 18 9 10 11 12 13 14 15
22 23 24 25 26 27 28 19 20 21 22 23 24 25 16 17 18 19 20 21 22
29 30 31 26 27 28 29 30 31 23 24 25 26 27 28 29
30
October November December
Mo Tu We Th Fr Sa Su Mo Tu We Th Fr Sa Su Mo Tu We Th Fr Sa Su
1 2 3 4 5 6 1 2 3 1
7 8 9 10 11 12 13 4 5 6 7 8 9 10 2 3 4 5 6 7 8
14 15 16 17 18 19 20 11 12 13 14 15 16 17 9 10 11 12 13 14 15
21 22 23 24 25 26 27 18 19 20 21 22 23 24 16 17 18 19 20 21 22
28 29 30 31 25 26 27 28 29 30 23 24 25 26 27 28 29
30 31
If you want to display a specific month from the year then you can do that using a calendar.month()
function.
Display Specific Month Calendar in Python
import calendar
year = int(input("Enter a year: "))
month = int(input("Enter a month: "))
# Display Specific Month Calendar
print(calendar.month(year, month))
Output
Enter a year: 2024
Enter a month: 2
February 2024
Mo Tu We Th Fr Sa Su
1 2 3 4
5 6 7 8 9 10 11
12 13 14 15 16 17 18
19 20 21 22 23 24 25
26 27 28 29
This is all about how we can display specific month or whole calendar using Python. I hope this post adds some value to your life – thank you for reading.