In this post, we will learn about the Python math
module in detail, with an explanation and examples. So let’s start learning from the very basics.
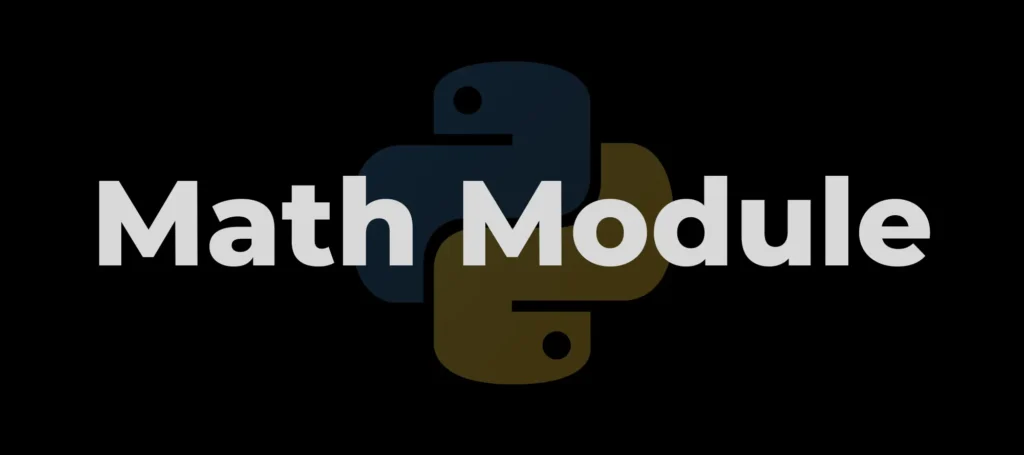
Introduction to math
module
Python math module is a built-in module that provides various functions and constants to perform the mathematical operations more accurately.
To use the math
module you need to import it:
import math
After importing the math
module into your program you have access to various functions and constants of the math
module let’s see all of those one by one with examples.
math.sqrt(x)
This function returns the square root of the input number x
.
import math
number = 16
square_root = math.sqrt(number)
print(f"The square root of {number} is {square_root}")
Output:
The square root of 16 is 4.0
math.sin(x), math.cos(x), and math.tan(x)
These functions return the sine, cosine, and tangent of the input angle x
(in radians), respectively.
import math
angle_in_degrees = 30
angle_in_radians = math.radians(angle_in_degrees)
sin_value = math.sin(angle_in_radians)
cos_value = math.cos(angle_in_radians)
tan_value = math.tan(angle_in_radians)
print("For angle", angle_in_degrees, "degrees:")
print("Sin:", sin_value)
print("Cos:", cos_value)
print("Tan:", tan_value)
Output:
For angle 30 degrees:
Sin: 0.49999999999999994
Cos: 0.8660254037844387
Tan: 0.5773502691896257
math.pi and math.e
These are mathematical constants representing π (pi) and Euler’s number (e) respectively.
import math
print("The value of π (pi) is:", math.pi)
print("The value of Euler's number (e) is:", math.e)
Output:
The value of π (pi) is: 3.141592653589793
The value of Euler's number (e) is: 2.718281828459045
math.factorial(x)
This function returns the factorial of the input integer x
.
import math
number = 5
factorial_result = math.factorial(number)
print(f"Factorial of {number} is {factorial_result}")
Output:
Factorial of 5 is 120
math.pow(x, y)
This function returns x
raised to the power y
.
import math
base = 2
exponent = 3
result = math.pow(base, exponent)
print(f"{base} raised to the power {exponent} is {result}")
Output:
2 raised to the power 3 is 8.0
math.gcd()
This function returns greatest common divisor (GCD) of two or more integers.
import math
n1 = 10
n2 = 25
print(f"GCD of {n1} & {n2} is {math.gcd(n1,n2)}")
Output:
GCD of 10 & 25 is 5
math.ceil(x)
This function returns the smallest integer greater than or equal to x
.
import math
number = 4.2
ceiled_value = math.ceil(number)
print(f"The ceil of {number} is {ceiled_value}")
Output:
The ceil of 4.2 is 5
math.floor(x)
This function returns the largest integer less than or equal to x
.
import math
number = 4.8
floored_value = math.floor(number)
print(f"The floor of {number} is {floored_value}")
Output:
The floor of 4.8 is 4
math.fabs(x)
This function returns the absolute value (magnitude) of the input number x
.
import math
number = -10.5
absolute_value = math.fabs(number)
print(f"The absolute value of {number} is {absolute_value}")
Output:
The absolute value of -10.5 is 10.5
math.exp(x)
This function returns the exponential value of x
, i.e., e raised to the power x
.
import math
x = 2
exp_value = math.exp(x)
print(f"The exponential value of {x} is {exp_value}")
Output:
The exponential value of 2 is 7.38905609893065
math.log(x, base)
This function returns the natural logarithm of x
to the specified base (default base is e
).
import math
x = 10
log_value = math.log(x, 2) # Logarithm base 2
print(f"The logarithm (base 2) of {x} is {log_value}")
Output:
The logarithm (base 2) of 10 is 3.3219280948873626
math.degrees(x) and math.radians(x)
import math
angle_in_degrees = 45
angle_in_radians = math.radians(angle_in_degrees)
print(f"{angle_in_degrees} degrees is equal to {angle_in_radians} radians")
# Convert back to degrees
angle_in_degrees = math.degrees(angle_in_radians)
print(f"{angle_in_radians} radians is equal to {angle_in_degrees} degrees")
Output:
45 degrees is equal to 0.7853981633974483 radians
0.7853981633974483 radians is equal to 45.0 degrees
math.isclose(a, b)
This function checks whether two floating-point numbers a
and b
are close to each other, considering relative and absolute tolerances.
import math
a = 0.1 + 0.1 + 0.1
b = 0.3
is_close = math.isclose(a, b)
print(f"Are {a} and {b} close? {is_close}")
Output:
Are 0.30000000000000004 and 0.3 close? True
This is all about the Python math
module. If you want to learn more about Python, then Click Here