In this post, you will learn python data types with detailed explanations and examples so let us start learning from the very basics.
What is Data Types
A variable is used to store different types of data and those types of data are known as data types.
Python is a dynamic programming language that’s why there is no need to specify data types before a variable name. at runtime python interpreter automatically binds value with its data types.
Example:
num = 9
print(num)
Output:
9 |
Here num
is an integer data type.
How to Check data types in python
With the help of the type()
Function in python, you can check the data types of any variable.
my_num = 25
print(type(my_num)) # Integer
my_num2 = 35.5
print(type(my_num2)) # FLoat
my_list = [1,2,3,4]
print(type(my_list)) #list
complex_value = 3+2j # complex
print(type(complex_value))
Output:
<class ‘int’> <class ‘float’> <class ‘list’> <class ‘complex’> |
Data Types in Python
Generally, there are five data types in python and they are as follows
- Numeric
- Sequence types
- Dictionary
- Set
- Boolean
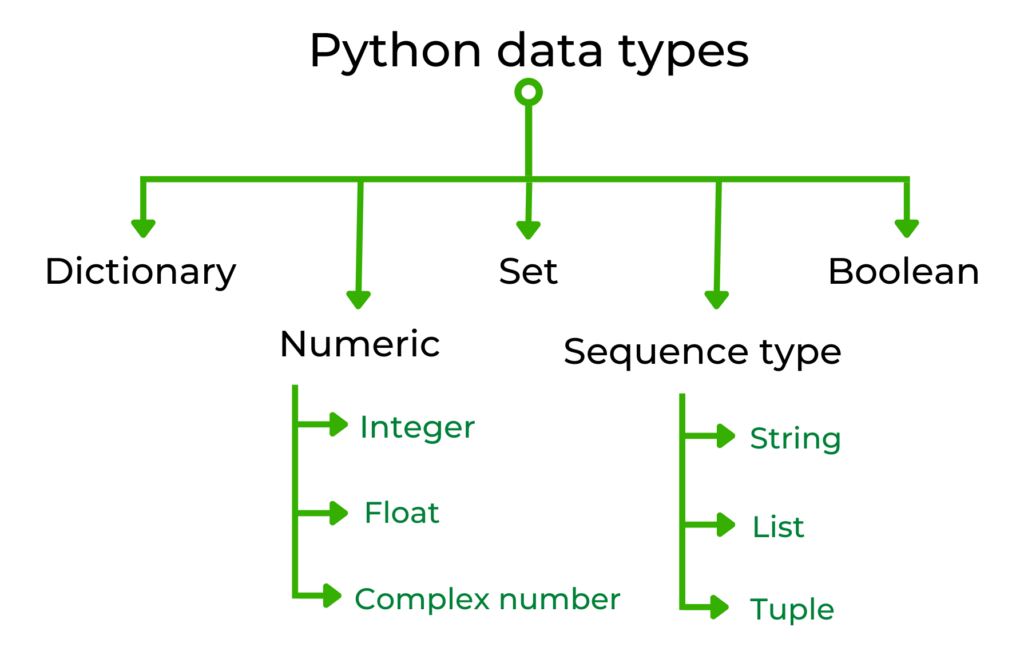
Numeric Data Types
There are three subtypes of Numeric data types
- Integer
- Float
- Complex number
Integer Data Types
Integer data types are used to store integer values such as …-3, -2, -1, 0, 1, 2, 3, 4… etc. you can store integer values of any length in python.
num = 35000
num2 = -72
print(num)
print(num2)
print(type(num))
print(type(num2))
Output:
35000 -72 <class ‘int’> <class ‘int’> |
Float Data Types
Float data types are used to store floating values such as -3.2, 1.1, 0.0, 5.4, etc.
num = 35.7
num2 = -7.3
print(num)
print(num2)
print(type(num))
print(type(num2))
Output:
35.7 -7.3 <class ‘float’> <class ‘float’> |
Complex Data Types
Complex data types are used to store complex numbers such as (3+7j), (5+9j), etc.
comp = 5+3j
print(comp)
print(type(comp))
Output:
(5+3j) <class ‘complex’> |
Sequence Data Types
There are three subtypes of Sequence data types
- String
- List
- Tuple
String
String is a sequence of characters but in python, you can say that anything inside single or double quotes ( ‘ ___’ or “___” ) is considered a string.
my_string = 'allinpython.com'
print(my_string)
print(type(my_string))
Output:
allinpython.com <class ‘str’> |
List
List is an ordered collection of items in python.
my_list = [1,4,6,8,9.1,'allinpython']
print(my_list)
print(type(my_list))
Output:
[1, 4, 6, 8, 9.1, ‘allinpython’] <class ‘list’> |
Tuple
Tuple is used to store a collection of items like integer, float, string, list, dictionary, set, etc in one variable.
A tuple is an immutable data structure in python.
t1 = (1,3,5,7,'allinpython',5.3) # create a tuple
print(t1)
print(type(t1))
Output:
(1, 3, 5, 7, ‘allinpython’, 5.3) <class ‘tuple’> |
Dictionary
dictionary is an unordered collection of data in a key: value pair.
name = {'name':'allinpython','age':19,'collage':'unknown'}
print(name)
print(type(name))
Output:
{‘name’: ‘allinpython’, ‘age’: 19, ‘collage’: ‘unknown’} <class ‘dict’> |
Sets
Set is an unordered collection of unique items in python
You can not store built-in data structures (like list, dictionary, set, etc.) inside a set except tuple.
set1 = {1,2,3,4,'five',(1,3,5,7)}
print(set1)
print(type(set1))
Output:
{1, 2, 3, 4, ‘five’, (1, 3, 5, 7)} <class ‘set’> |
Boolean
The boolean data type has two built-in values that are True & False.
game_start = True
game_over = False
print(game_start)
print(game_over)
print(type(game_over))
Output:
True False <class ‘bool’> |