In this post, we will discuss Python data structure interview questions with their answers. all those questions that we will discuss are frequently asked in the interview so without wasting any time let’s go and discuss all the questions with their solutions.
The very first question that is asked in any Data Structure Interview is “What is a data structure?”, to answer this question you have to explain the basics of data structure and their types. let’s see how would you explain it.
Q.1 What is Data Structure?
Data structures are formats for organizing, storing, retrieving, and manipulating data in an efficient way in memory.
Types of data Structures in Python
There are two types of data structures in Python:
- Built-in Data Structures
- User-define Data Structures
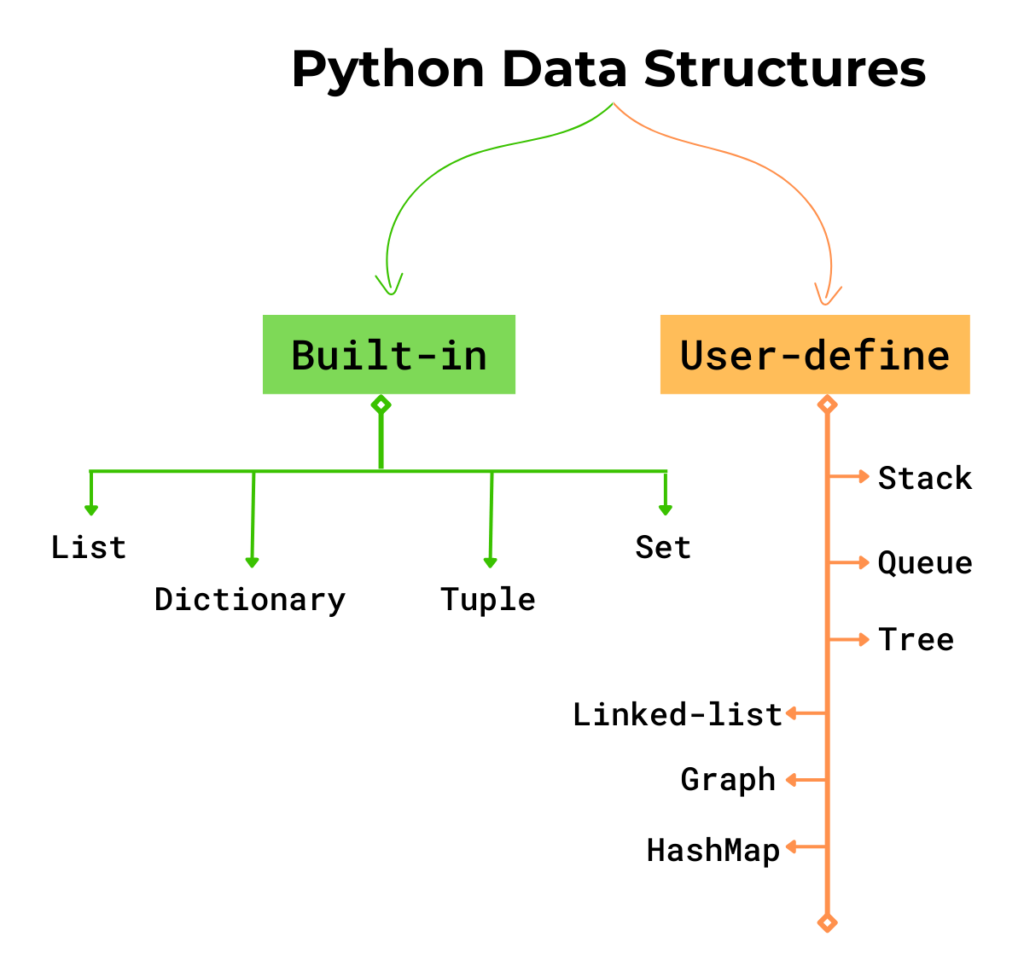
Q.2 what is list in python?
The List is a built-in data structure in Python and it will allow you to store multiple data items into one variable and all the data items are arranged in a specified order (you can access all the data inside the list using indexing).
# Create a List
my_list = [1,2,3]
print(my_list) # Output: [1, 2, 3]
# Access elements of the list using indexing
print(my_list[0]) # Output: 1
# add data into the list
my_list.append(4)
print(my_list) # Output: [1,2,3,4]
# modify data of the list
my_list[-1] = 5
print(my_list) # Output: [1, 2, 3, 5]
#delete data of the list
my_list.pop()
print(my_list) # Output: [1, 2, 3]
# mixed list
mixed_list = ["string",1,1.5,True,["allinpython",True,1.5]]
print(mixed_list) # Output: ['string', 1, 1.5, True, ['allinpython', True, 1.5]]
Q.3 How the list is different From an Array?
A traditional Array stores similar data types of data and it has a fixed size while the list is dynamic (not fixed size) and inside the list you can store any data types of data.
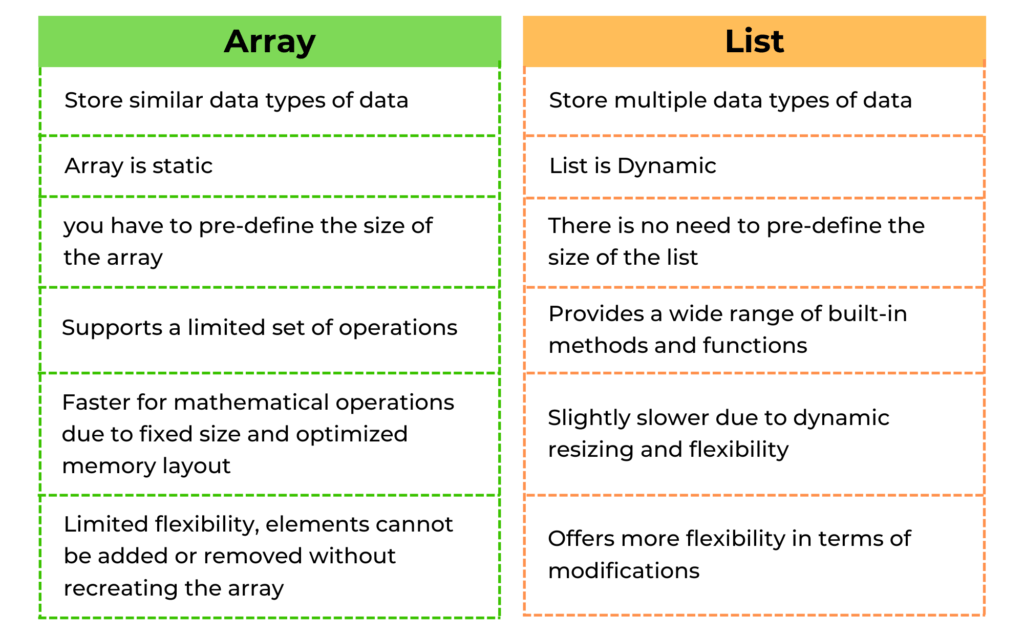
Q.4 What is tuple in Python?
Tuple is an immutable order data structure in Python, you can store multiple data types of data inside the tuple but once the tuple is created you can not modify it.
# Create tuple
my_tuple = (1,4,9,16,25)
print(my_tuple) # Output: (1, 4, 9, 16, 25)
# Access elements of the tuple
print(my_tuple[1]) # Output: 4
# Create a tuple with single element
t = (1,) # Output: (1,)
print(t)
Q.5 Why do we use tuple if we have list which is more flexible than tuple?
We use a tuple because it is much faster as compared to the list. Sometimes we need to store static data in our program, at that time tuple is best to store static data instead of a list. also, tuples take less memory as compared to lists.
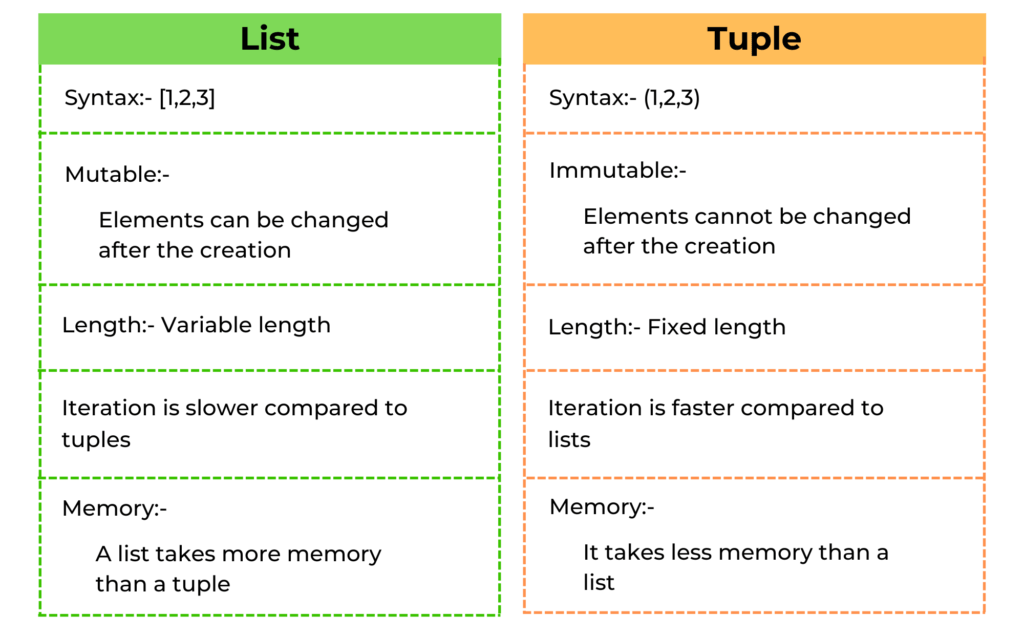
Q.6 What is a dictionary and why do we use it?
A dictionary is an unordered collection of data in a key: value
pair. One of the best reasons to use a dictionary is, it is a more efficient data structure (as compared to the list) to store real data items.
Student_info1 = {
"name": "joy",
"roll no": 13,
"marks": 93,
"result": "Pass",
"grade":"A+"
}
print(Student_info1) # Output: {'name': 'joy', 'roll no': 13, 'marks': 93, 'result': 'Pass', 'grade': 'A+'}
Q.7 Difference between list and a dictionary.
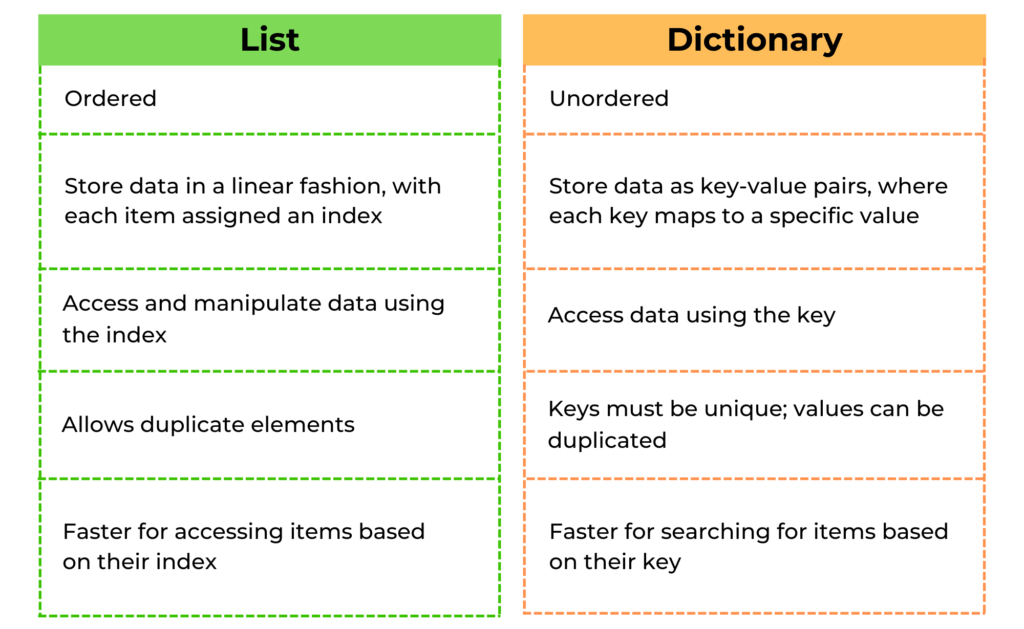
Q.8 How to add Data items in the List
There are different methods of the list that are used to add data items to the list and they are as follows:
- .append()
- .insert()
- .extend()
.append()
method is used to add data items at the end of the list.
list1 = [1,2,3,4]
list1.append(5) #add 5
print(list1) # Output: [1, 2, 3, 4, 5]
.insert()
method is used to add data items in a specified index position of the list.
list2 = [1,3,7]
list2.insert(2,5)
print(list2) # Output: [1, 3, 5, 7]
.extend()
method is used to add the whole data of the list into another list.
list1 = [1,2,3]
list2 = [4,5,6]
list1.extend(list2) #add data of list2 into list1
print(list1)
Q.9 Why do we use set if we have a list, tuple, dictionary, etc
A Set is an unordered collection of unique data items and there are many uses of a set few are listed below:
- One of the most important uses of a set is to remove duplicate data or items from a list.
- It is also used to perform union and intersection operations between two sets.
Q.10 How to add and access data inside a dictionary?
To add data inside the dictionary we use .update()
method and to access data inside a dictionary we use the.get()
method (also uses the keys of the dictionary).
# .update() method
emp_detail = {
"name": "Yagyavendra"
}
emp_detail.update({"salary":93000})
print(emp_detail) # Output: {'name': 'Yagyavendra', 'salary': 93000}
# another way to add data inside dictionary
emp_detail["address"] = "India"
print(emp_detail) # Output: {'name': 'Yagyavendra', 'salary': 93000, 'address': 'India'}
# .get() method
print(emp_detail["salary"]) # Output: 93000
# another ways to access data inside dictionary (using keys)
print(emp_detail["name"]) # Output: Yagyavendra
Q.11 How many different ways to define an empty set in Python?
There is only one way to define an empty set in Python, and that is using the set()
function.
# Create an empty set
my_set = set()
print(my_set) # Output: set()
# add data inside a set
my_set.add(1)
my_set.add(2)
my_set.add(3)
print(my_set) # Output: {1, 2, 3}
# delete data from the set
my_set.remove(2)
print(my_set) # Output: {1, 3}
Q.12 How to create a tuple with a single element?
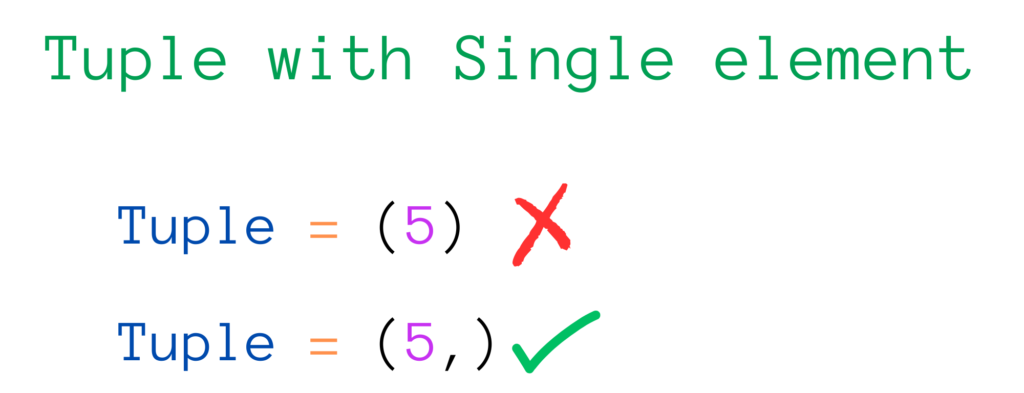
Q.13 Difference between stack and queue.
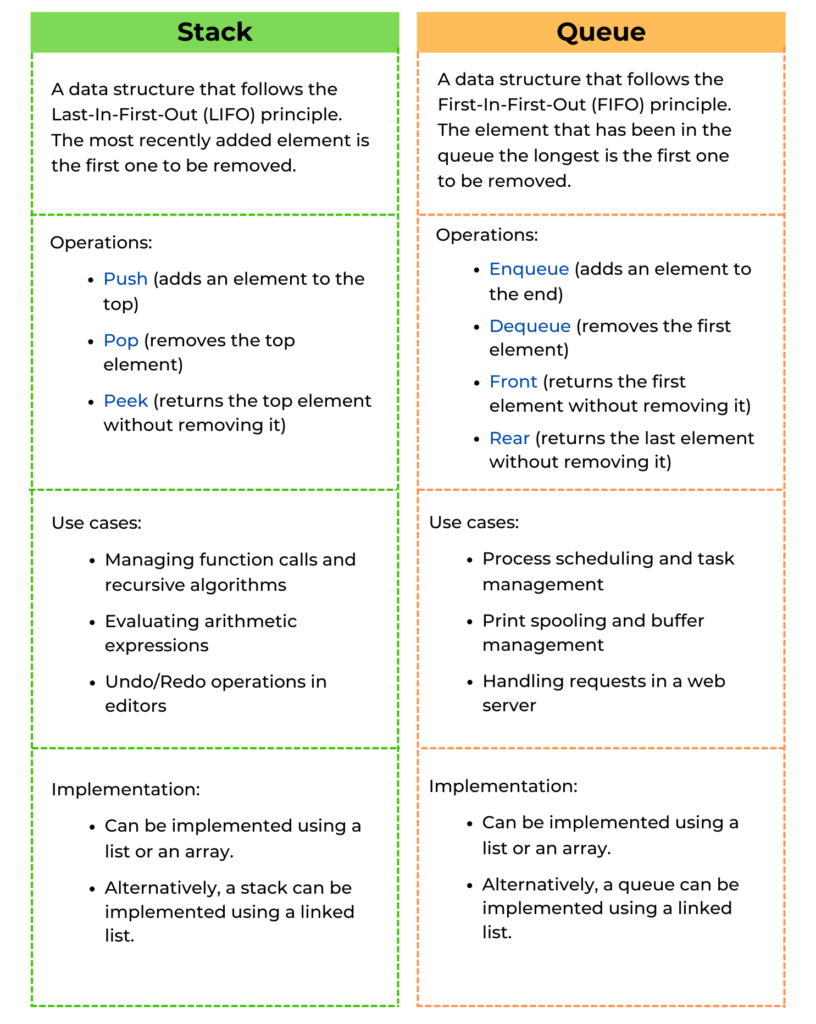