In this post, we will learn how to print prime numbers in python from 1 to 100, 1 to n, and in a given interval but before we jump into a program let’s understand what is a prime number?
What is a prime number?
A natural number that is only divisible by 1 and itself is called a prime number.
For example: 2,3,5,7,11,13,17,19….
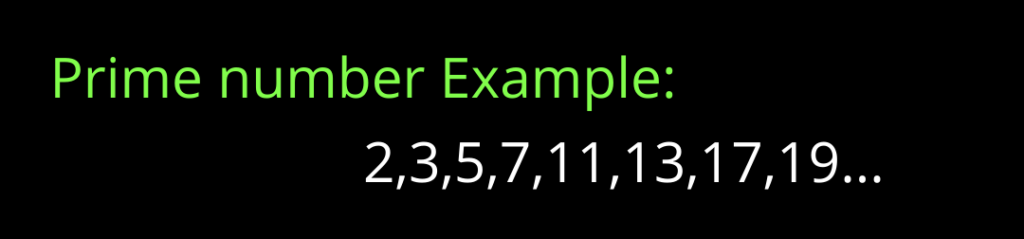
Algorithm to Print Prime Numbers from 1 to 100
Step-1: iterate a for loop in range 2 to100 –> for i in range(2,101)
Step-2: inside the first loop create another for loop in the range 2 to 100 –> for j in range(2,101)
Step-3: check if i%j == 0
then break a loop (Because a number is not prime)
Step-4: step-3 implies that if a number i
is not divisible by j
then the program never enters in if-block, this means at the end of the second for-loop i == j
Step-5: if i == j
then print i
( Because i
is your prime number)
Now we understand what is prime number and how to implement a prime number program in python so now it’s time to do it practically.
1. Write a python program to print prime numbers from 1 to 100 using a for loop
Few concepts you know before writing this program such as
Source code:
for i in range(2,101): # The range() function does not include the last number (the ending number). Only numbers from 2 to 100 are counted.
for j in range(2,101):
if i%j == 0:
break
if i == j:
print(i,end=",")
Output:
2,3,5,7,11,13,17,19,23,29,31,37,41,43,47,53,59,61,67,71,73,79,83,89,97,
2. Write a python program to print prime numbers from 1 to 100 using a while loop
In the case of a while loop Above algorithm is used only syntax is changed.
Few concepts you know before writing this program such as
- while loop
- nested while loop
- if-else
Source code:
i = 2
while i <= 100:
j = 2
while j < 100:
if i%j == 0:
break
j += 1
if i == j:
print(i,end=',')
i += 1
Output:
2,3,5,7,11,13,17,19,23,29,31,37,41,43,47,53,59,61,67,71,73,79,83,89,97,
3. Write a python program to print prime numbers from 1 to N using a for loop
NOTE: In this program, we will change only the user input num
with the static value 101
of the above program.
Few concepts you know before writing this program such as
- how to take user input
- for loop
- nested for loop
- if-else
Source code:
num = int(input("Enter a number: "))
for i in range(2,num+1):
for j in range(2,num+1):
if i%j == 0:
break
if i == j:
print(i,end=',')
Output:
Enter a number: 50
2,3,5,7,11,13,17,19,23,29,31,37,41,43,47,
4. Write a python program to print prime numbers from 1 to N using a while loop
NOTE: In this program, we will change only the user input num
with the static value 100
of the above program.
Few concepts you know before writing this program such as
- how to take user input
- while loop
- nested while loop
- if-else
Source code:
num = int(input("Enter a number: "))
i = 2
while i <= num:
j = 2
while j < num:
if i%j == 0:
break
j += 1
if i == j:
print(i,end=',')
i += 1
Output:
Enter a number: 70
2,3,5,7,11,13,17,19,23,29,31,37,41,43,47,53,59,61,67,
5. write a python program to print prime numbers in a given range using a for loop
NOTE: In this program, we will change only the upper limit and lower limit of the range()
function with user input.
Source code:
num1 = int(input("Enter a first number: ")) #lower limit
num2 = int(input("Enter a second number: ")) #upper limit
if num1<num2:
for i in range(num1,num2+1):
for j in range(2,num2+1):
if i%j == 0:
break
if i == j:
print(i,end=',')
else:
print('invalid number!')
Output:
Enter a first number: 4
Enter a second number: 7
5,7,
6. write a python program to print prime numbers in a given range using a while loop
NOTE: In this program, we will change only the upper limit and lower limit of the while-loop with user input.
num1 = int(input("Enter a first number: ")) #lower limit
num2 = int(input("Enter a second number: ")) #upper limit
if num1<num2:
while num1 <= num2:
j = 2
while j < num2:
if num1%j == 0:
break
j += 1
if num1 == j:
print(num1,end=',')
num1 += 1
else:
print('invalid input')
Output:
Enter a first number: 9
Enter a second number: 19
11,13,17,19,
From all the above 6 programs we understand how to print prime number sequences.
This is a bonus for the prime number program.
Write a python program to create a list of prime numbers from 1 to N
Few concepts you know before writing this program such as
- python list
- function in python
- for loop
- nested for loop
- if-else
Source code:
def prime_range(num):
prime = []
for i in range(2,num+1):
for j in range(2,num+1):
if i%j == 0:
break
if i == j:
prime.append(i)
return prime
number = int(input('Enter a number: '))
print(prime_range(number))
print('sum of prime numbers',sum(prime_range(num= number)))
Output:
Enter a number: 20
[2, 3, 5, 7, 11, 13, 17, 19]
sum of prime numbers 77
Hope this post adds some value to your life – thank you.