In this post, we will learn how to write a program to print odd numbers from 1 to 100 in Python using for-loop, while-loop, function, etc. with proper algorithm and explanation.
We will also see different variations of this program, such as printing odd numbers from 1 to N, printing odd numbers in a given range, etc.
So let’s start with the very basics: What is an odd number?
What is Odd Number?
An integer number that is not divisible by 2 is known as an odd number.
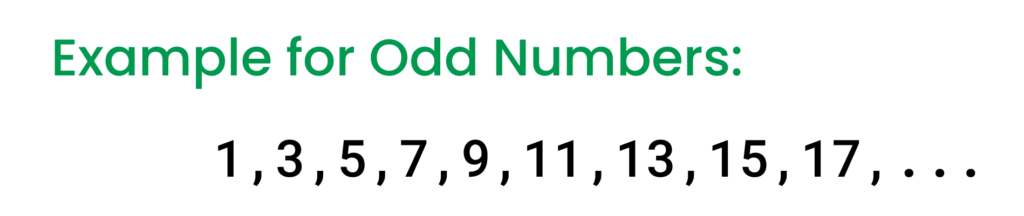
Now the basics are clear, So let’s jump into the programming part but few programming concepts you have to know before writing all this program and those are:
Print Odd Numbers from 1 to 100 in Python Using for-loop
Let’s discuss the algorithm first then we will write code for it.
Algorithm:
- Take a for-loop from 1 to 101 –> (
for i in range(1,101):
) - Inside for-loop check
if i % 2 != 0:
then do step-3 print(i)
- End program.
Source Code:
# The range function does not include the end value, so only numbers from 1 to 100 are considered.
for i in range(1, 101):
if i % 2 != 0:
print(i,end=",")
Output:
1,3,5,7,9,11,13,15,17,19,21,23,25,27,29,31,33,35,37,39,41,43,45,47,49,51,53,55,57,59,61,63,65,67,69,71,73,75,77,79,81,83,85,87,89,91,93,95,97,99,
There is an alternate approach using a for-loop where you can use the step argument of the range function. As we know range function takes three arguments (start: end: step
). step argument helps us to skip the iteration in the for-loop, so we will skip one iteration and print odd numbers.
Let’s see it with the help of an example:
Source Code:
for i in range(1, 101, 2):
print(i, end=",")
Output:
1,3,5,7,9,11,13,15,17,19,21,23,25,27,29,31,33,35,37,39,41,43,45,47,49,51,53,55,57,59,61,63,65,67,69,71,73,75,77,79,81,83,85,87,89,91,93,95,97,99,
Print Odd Numbers from 1 to 100 in Python Using while-loop
Algorithm:
- Take one variable called “num” initialize it with 0 (
num = 0
). - Iterate using while-loop as long as
num
is less or equal to 100 (while num <= 100:
) - Inside the while-loop check
if num % 2 != 0
then do step-4 print(num)
- Outside of if-block, increment
num
by 1 (num = num + 1
) - End program.
Source Code:
num = 0
while num <= 100:
if num % 2 != 0:
print(num, end=",")
num+=1
Output:
1,3,5,7,9,11,13,15,17,19,21,23,25,27,29,31,33,35,37,39,41,43,45,47,49,51,53,55,57,59,61,63,65,67,69,71,73,75,77,79,81,83,85,87,89,91,93,95,97,99,
Print Odd Numbers from 1 to 100 in Python Using Function
Algorithm:
- Define a function that will take one argument (
def print_odd(num)
) - Inside function body takes a for-loop from 1 to
num
(for i in range(1, num+1)
). - Inside the for-loop, check
if i % 2 != 0:
then do step-4 print(i)
- Outside of the function Take variable
n = 100
- Call a function and pass variable
n
(print_odd(n)
) - End program
Source Code:
def print_odd(num):
for i in range(1, num+1):
if i % 2 != 0:
print(i,end=",")
n = 100
print_odd(n) # calling a function
Output:
1,3,5,7,9,11,13,15,17,19,21,23,25,27,29,31,33,35,37,39,41,43,45,47,49,51,53,55,57,59,61,63,65,67,69,71,73,75,77,79,81,83,85,87,89,91,93,95,97,99,
Now let us modify all three above programs and write them by taking input from the user.
Print Odd Numbers from 1 to N in Python Using for-loop
Source Code:
num = int(input("Enter a number: "))
for i in range(1,num+1):
if i % 2 != 0:
print(i, end=',')
Output:
Enter a number: 10
1,3,5,7,9,
Print Odd Numbers from 1 to N in Python Using while-loop
Source Code:
num = int(input("Enter a number: "))
i = 0
while i<=num:
if i % 2 != 0:
print(i,end=',')
i+=1
Output:
Enter a number: 50
1,3,5,7,9,11,13,15,17,19,21,23,25,27,29,31,33,35,37,39,41,43,45,47,49,
Print Odd Numbers from 1 to N in Python Using Function
Source Code:
def print_odd(num):
for i in range(1, num+1):
if i % 2 != 0:
print(i,end=",")
n = int(input("Enter a number: "))
print_odd(n)
Output:
Enter a number: 20
1,3,5,7,9,11,13,15,17,19,
Print Odd Numbers in the given Range
Source Code:
def print_odd(num1,num2):
for i in range(num1, num2+1):
if i % 2 != 0:
print(i,end=",")
n1 = int(input("Enter a number-1: "))
n2 = int(input("Enter a number-2: "))
print_odd(n1,n2)
Output:
Enter a number-1: 10
Enter a number-2: 20
11,13,15,17,19,