print()
Function in python is used to print anything in the console or screen.
the print()
function is one of the most important and useful function in python.
In Python2 print
is a keyword but from Python3 print
is treated as a function. this is the basic difference between Python2 and Python3.
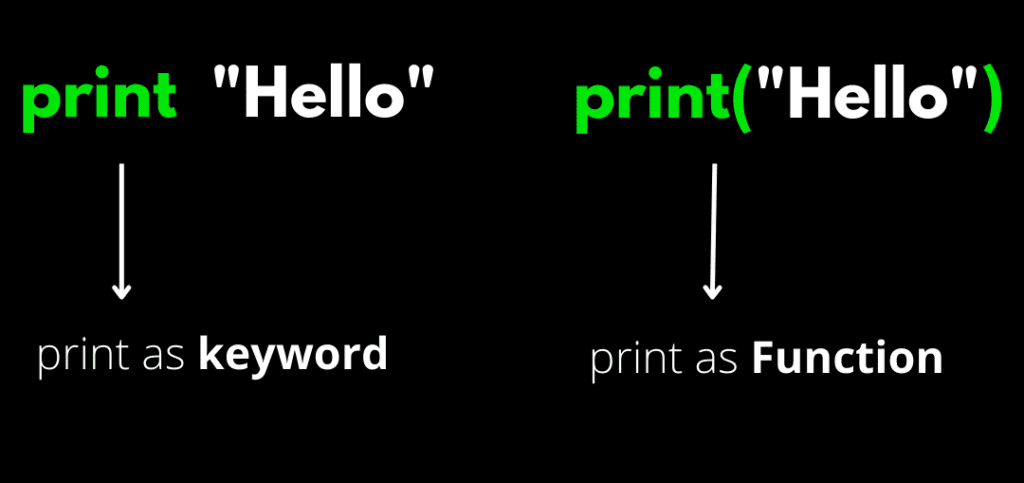
let’s write our first program using the print()
function in python
print("Hello world")
Output:
Hello world
NOTE: It is compulsory to write a string inside a single quote (‘…’) or double quote (“…”) otherwise it will give an error.
Perform arithmetic operation inside print() function
you can also perform arithmetic operations such as addition, subtraction, multiplication, division, etc. inside the print()
function.
print(2+3) #5
print(3+5+1) #9
print(5-7) #-2
print(7-1) #6
print(3*4) #12
print(10/5) #2.0
You can also concatenate two strings inside the print()
function.
first_name = "allin"
last_name = "python"
print(first_name + last_name)
Output:
allinpython
Print Python Escape Characters as a normal character
\n, \r, \ t all are python escape characters we are writing a program to print those escape characters as normal characters.
print("\\n")
print("\\r")
print("\\")
print("\\t")
Output:
\n
\r
\
\t
print() function without new line
Let’s understand how to print multiple print statements in a single line with the help of an example.
print("this is fist line ", end="-->")
print("this is second line")
Output:
this is fist line -->this is second line
the end
is an attribute of the print()
function that voids (or prevents) printing in the next line.
Instead of writing "-->"
, you can write any other characters for example (*, &, #, etc..,).
Without using the end
attribute output of the above program is:
print("this is fist line ")
print("this is second line")
Output:
this is fist line
this is second line
Hope this content adds some value to your life – thank you.
1 Comment
it is very easy to understand