In this post, we will learn map() and filter() Functions in Python with detailed explanation and examples.
So let us start learning map() and filter() function in python one by one.
map()
Function
The map() function is a high-order function in Python and it is used to apply a function to every element of an iterable, such as a list or tuple, and returns a new iterable object (which is an iterator) with the modified elements.
Syntax of map()
Function
syntax –> map(function, iterable)
- Here, the function is the name of the function that is applied to every element of the iterable. (instead of defining a function separately you can also use lambda expression)
- the iterable is the sequence of elements such as a List, tuple, dictionary, etc.
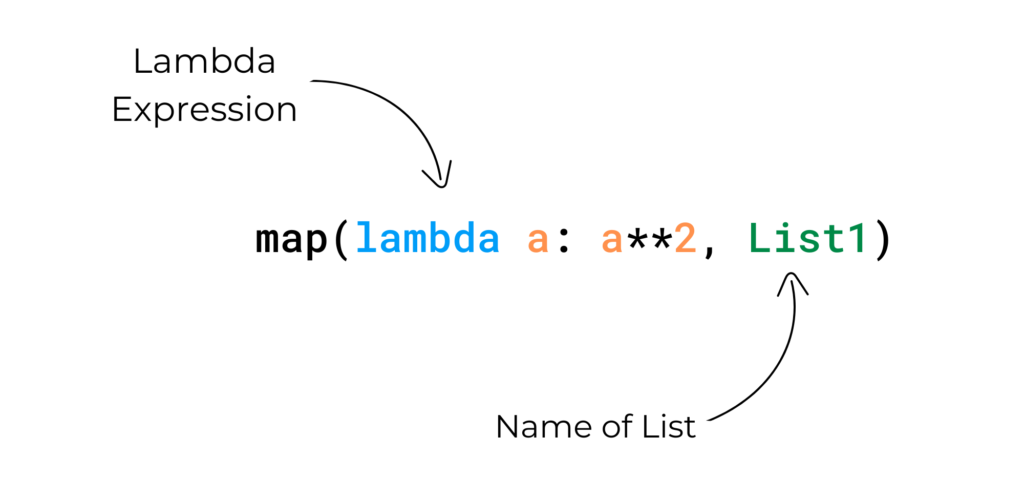
Let’s take a few examples to understand the map() function more efficiently.
Example-1
For Example, you have one list List1 = [2,3,5,7,9]
, and you want to create another list with a square of the elements in List1
.
There are many ways to achieve the desired output but the more efficient way is using the map() function.
def square(a):
return a**2
List1 = [2,3,5,7,9]
square_list1 = map(square,List1)
print(square_list1)
Output
<map object at 0x000001A2E1FACFA0> |
As we discussed, the map() function returns a new iterable object (or map object) as shown in the above output.
To see the output of the map object you can convert it into the list or you can use a for-loop.
So let’s convert the above map object into the list using the list()
function and see the output.
def square(a):
return a**2
List1 = [2,3,5,7,9]
square_list1 = list(map(square,List1))
print(square_list1)
Output
[4, 9, 25, 49, 81] |
In the above example, we define a function (square
) separately; however, we can also use a lambda function to make our code more readable.
# map() function with lambda expression
List1 = [2,3,5,7,9]
square_list1 = list(map(lambda a: a**2,List1))
print(square_list1)
Output
[4, 9, 25, 49, 81] |
Example-2
For example, let’s say we have a list of strings, and we want to create a new list that contains each string converted to uppercase. We can do this using map()
and the built-in str.upper()
method:
strings = ["Hello", "world", "allinpython"]
uppercase_strings = list(map(str.upper, strings))
print(uppercase_strings)
Output
[‘HELLO’, ‘WORLD’, ‘ALLINPYTHON’] |
map()
with multiple iterable objects
map()
function can also be used with multiple iterable objects. In this case, the function must take the same number of arguments as the number of iterable objects passed to the map()
.
For example, let’s say we have two lists, and we want to create a new list that contains the sum of each pair of corresponding elements from the two lists. We can do this using map()
and a simple lambda function:
list1 = [1, 2, 3, 4, 5]
list2 = [10, 20, 30, 40, 50]
sums = list(map(lambda x, y: x + y, list1, list2))
print(sums)
Output
[11, 22, 33, 44, 55] |
filter()
Function
The filter() function is a high-order function in Python and it is used to filter out elements from an iterable (such as a list or tuple) based on a specific condition (or Function).
The filter() function takes two arguments: a function and an iterable. The function takes an element from the iterable and returns True or False. The filter() function then returns an iterator that contains only the elements for which the function returned True.
Syntax of filter()
Function
Syntax –> filter(function, iterable)
- Here, the function is the name of the function that is used to filter the elements from the iterable. (instead of defining a function separately you can also use lambda expression)
- the iterable is the collection of elements such as a List, tuple, dictionary, etc. that need to be filtered.
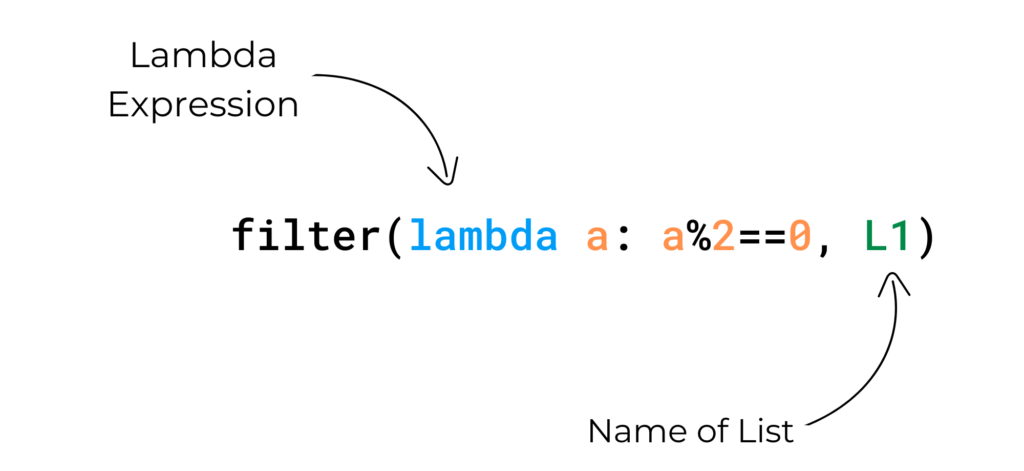
Let’s take a few examples to understand filter function more efficiently:
Example-1
Suppose you have one list L1 = [1,2,3,4,5,6,7,8,9,10]
and you want to filter out only even numbers from the list (L1
). We can use the filter() function to achieve this as follows:
L1 = [1,2,3,4,5,6,7,8,9,10]
even_l1 = list(filter(lambda a: a%2 == 0,L1))
print(even_l1)
Output
[2, 4, 6, 8, 10] |
Here in the above example, we are converting the filter object to the list.
Example-2
Another example, suppose you have one mixed list (mixed_list = ["hello", "allinpython", 23.5, 12, "python", 7, 2.1, 1]
) and you want to filter out only integer and float numbers. We can use the filter() function to achieve this as follows:
mixed_list = ["hello", "allinpython", 23.5, 12, "python", 7, 2.1, 1]
num_list = list(filter(lambda a: type(a) == int or type(a) == float, mixed_list))
print(num_list)
Output
[23.5, 12, 7, 2.1, 1] |
Benefits of map()
and filter()
function
The filter()
and map()
functions in Python have several benefits, including:
- Readability: Both functions allow you to write clean and concise code that is easy to read and understand.
- Improved performance: Using these functions instead of writing a loop can result in better performance because the functions are implemented in C and are optimized for speed.
- Flexibility: The functions can be used with a wide variety of data types, including lists, tuples, and dictionaries.
- Easy to use: The functions have a simple syntax and are easy to use.