In this article, we will take a detailed look at the differences between lists and tuples in Python, and provide examples to illustrate how they are used in real-world scenarios.
Both lists and tuples are used to store a collection of items. However, there are some key differences between the two that are important to understand.
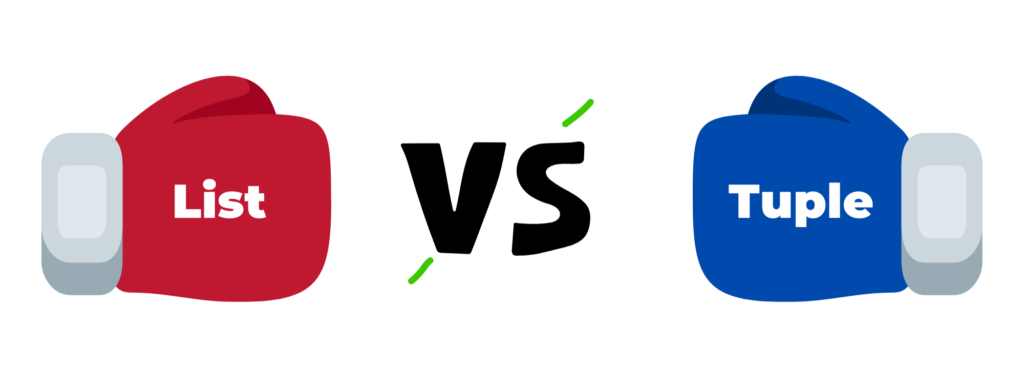
Mutability
The main difference between lists and tuples is their mutability. Lists are mutable, which means that you can add, remove, or modify elements in a list after it has been created.
For example, you can use the append()
method to add elements to a list, the remove()
method to remove elements from a list, and the indexing operator []
to change the value of a specific element in a list.
On the other hand, tuples are immutable, which means that once a tuple is created, you cannot add, remove, or modify elements within it. If you try to do so, you will get a TypeError.
This makes tuples useful for situations where you need to store a collection of items that will not change, such as fixed configuration settings or the elements of a math equation.
Syntax
Another difference between lists and tuples is their syntax. Lists use square brackets ([]), while tuples use parentheses (()).
For example:
#Creating a list
my_list = [1, 2, 3]
#Changing values in list
my_list[1] = 4
print(my_list) # [1, 4, 3]
#Creating a tuple
my_tuple = (1, 2, 3)
#trying to change values in tuple
my_tuple[1] = 4
#throws an error
Additionally, lists have several built-in methods that allow you to manipulate the data, like append()
, extend()
, insert()
, remove()
, pop()
, sort()
, etc. On the other hand, tuples do not have many built-in methods, and the ones available are mainly used to return a specific element or slice of the tuple.
Memory and Performance
Another important difference between lists and tuples is related to memory and performance.
Lists are more flexible and powerful than tuples, but they also use more memory and are slower. This is because lists use a dynamic array to store the elements, which means that the size of the list can change as elements are added or removed. This requires more memory and processing power.
On the other hand, tuples use a static array to store the elements, which means that the size of the tuple is fixed. This makes them more memory-efficient and faster than lists, but also less flexible.
List vs Tuple (Summary)
Here’s a table that summarizes the main differences between lists and tuples:
Feature | Lists | Tuples |
Definition | A list is an ordered collection of items, enclosed in square brackets. | A tuple is an ordered collection of items, enclosed in round brackets. |
Mutability | Lists are mutable, which means that their elements can be modified after they are created. | Tuples are immutable, which means that their elements cannot be modified after they are created. |
Syntax | list_name = [item1, item2, item3] | tuple_name = (item1, item2, item3) |
Memory | lists use more memory as compared to tuple | tuple uses less memory as compared to the list |
Performance | list is slower | tuple is faster than the list. |