In this post, we will learn about python keywords and their uses with examples.
So let’s start from the very basics..
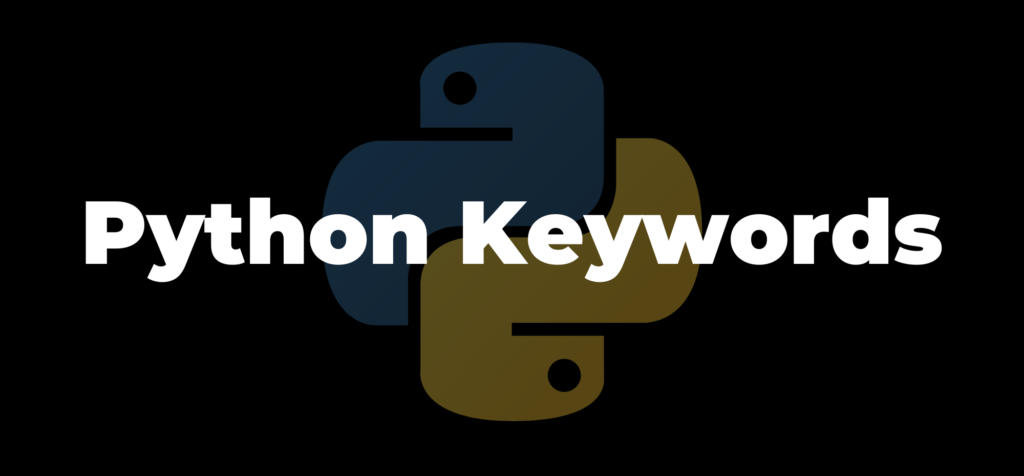
What are Keywords in python
In Python, a keyword is a word that has a special meaning in the Python language. Keywords are used to define the syntax and structure of the Python language, and they cannot be used as variables or identifiers in your code.
List of Python Keywords
There are a total of 35 keywords in Python. Here is a list of all the Python keywords:
Keyword | Description |
---|---|
and | Used to combine two Boolean expressions. Returns True if both expressions are True , and False otherwise. |
as | Used to import a module or package and give it an alias. |
assert | Used to test a condition and raise an exception if the condition is False . |
async | Used to define an asynchronous function. |
await | Used to wait for the completion of an asynchronous function. |
break | Used to exit a loop prematurely. |
class | Used to define a new class. |
continue | Used to skip the rest of the current iteration of a loop and move on to the next iteration. |
def | Used to define a new function. |
del | Used to delete an object or an element from a list. |
elif | Used in an if statement to specify additional conditions to be tested if the previous conditions are False . |
else | Used in an if statement to specify a block of code to be executed if all the previous conditions are False . |
except | Used in a try statement to specify a block of code to be executed if an exception is raised. |
False | Represents the Boolean value False . |
finally | Used in a try statement to specify a block of code that will always be executed, regardless of whether an exception is raised. |
for | Used to create a loop that iterates over a sequence of elements. |
from | Used to import specific modules or symbols from a package or module. |
global | Used to declare a variable as global. |
if | Used to specify a block of code to be executed if a condition is True . |
import | Used to import a module or package. |
in | Used to test if an element is contained in a sequence. |
is | Used to test if two objects are equal. |
lambda | Used to create a small anonymous function. |
None | Represents the null value. |
nonlocal | Used to declare a variable as nonlocal. |
not | Used to negate a Boolean expression. |
or | Used to combine two Boolean expressions. Returns True if either expression is True , and False otherwise. |
pass | Used as a placeholder for a block of code that does nothing. |
raise | Used to raise an exception. |
return | Used to exit a function and return a value. |
True | Represents the Boolean value True . |
try | Used to specify a block of code to be tested for exceptions. |
while | Used to create a loop that continues as long as a condition is True . |
with | Used to create a context manager that manages the execution of a block of code. |
yield | Used in a function to return a value and pause the function’s execution. The function can be resumed later from where it left off. |
It is important to note that Python is a case-sensitive language so “True” and “true” are not the same keyword.
You should avoid using these keywords as variable names or function names in your code to avoid any syntax errors or unexpected behavior. Instead, you should use descriptive names for your variables and functions that accurately reflect their purpose in your code.
All the Python Keywords with Example
Here is a brief explanation of each Python keyword, along with an example of how it can be used in your code:
and
: Used to combine two Boolean expressions. Returns True
if both expressions are True
, otherwise returns False
.
#Example
x = 3
y = 5
if x > 0 and y > 0:
print("Both x and y are positive.")
as
: Used to import a module or package and give it an alias.
#Example
import numpy as np
assert
: Used to test a condition and raise an exception if the condition is False
.
#Example
x = -3
assert x > 0, "x must be positive."
async
: Used to define an asynchronous function.
#Example
async def fetch_data():
# Asynchronous code here
pass
await
: Used to wait for the completion of an asynchronous function.
async def main():
data = await fetch_data()
# Do something with the data
pass
break
: Used to exit a loop prematurely.
for i in range(10):
if i == 5:
break
print(i)
class
: Used to define a new class.
class Dog:
def __init__(self, name, breed):
self.name = name
self.breed = breed
continue
: Used to skip the rest of the current iteration of a loop and move on to the next iteration.
for i in range(10):
if i % 2 == 0:
continue
print(i)
def
: Used to define a new function.
def greet(name):
print("Hello, " + name + "!")
del
: Used to delete an object or an element from a list.
x = 10
del x
elif
: Used in an if
statement to specify additional conditions to be tested if the previous conditions are False
.
x = -3
if x > 0:
print("x is positive.")
elif x < 0:
print("x is negative.")
else:
print("x is zero.")
else
: Used in an if
statement to specify a block of code to be executed if all the previous conditions are False
.
x = -5
if x > 0:
print("x is positive.")
else:
print("x is not positive.")
except
: Used in a try
statement to specify a block of code to be executed if an exception is raised.
try:
# Code that might raise an exception
pass
except SomeException:
# Code to handle the exception
pass
False
: Represents the Boolean value False
.
x = False
if x == False:
print("x is False.")
finally
: Used in a try
statement to specify a block of code that will always be executed, regardless of whether an exception is raised.
try:
# Code that might raise an exception
pass
except SomeException:
# Code to handle the exception
pass
finally:
# Code that will always be executed
pass
for
: Used to create a loop that iterates over a sequence of elements.
for i in range(10):
print(i)
from
: Used to import specific modules or symbols from a package or module.
from math import pi
global
: Used to declare a variable as global.
x = 10
def increment():
global x
x += 1
print(X)
if
: Used to specify a block of code to be executed if a condition is True
.
x = 9
if x > 0:
print("x is positive.")
import
: Used to import a module or package.
import math
in
: Used to test if an element is contained in a sequence.
my_list = [1,2,3,4,5,6,7]
x = 5
if x in my_list:
print("x is in the list.")
is
: Used to test if two objects are equal.
x = None
if x is None:
print("x is None.")
lambda
: Used to create a small anonymous function.
double = lambda x: x * 2
None
: Represents the null value.
x = None
nonlocal
: Used to declare a variable as nonlocal.
def outer():
x = 10
def inner():
nonlocal x
x += 1
inner()
return x
not
: Used to negate a Boolean expression.
x = False
if not x:
print("x is False.")
or
: Used to combine two Boolean expressions. Returns True
if either expression is True
, and False
otherwise.
x = 3
y = -5
if x > 0 or y > 0:
print("Either x or y, or both, are positive.")
pass
: Used as a placeholder for a block of code that does nothing.
def do_nothing():
pass
raise
: Used to raise an exception.
raise ValueError("Invalid value.")
return
: Used to exit a function and return a value.
def double(x):
return x * 2
True
: Represents the Boolean value True
.
x = True
if x == True:
print("x is True.")
try
: Used to specify a block of code to be tested for exceptions.
try:
# Code that might raise an exception
pass
except SomeException:
# Code to handle the exception
pass
while
: Used to create a loop that continues as long as a condition is True
.
x = 10
while x > 0:
print(x)
x -= 1
with
: Used to create a context manager that manages the execution of a block of code.
with open("file.txt", "r") as f:
data = f.read()
yield
: Used in a function to return a value and pause the function’s execution. The function can be resumed later from where it left off.
def infinite_sequence():
i = 0
while True:
yield i
i += 1