In this post, we will learn a python program to check if a year is a leap year or not with a very simple explanation but before writing a program let’s understand what is a leap year.
What is Leap Year ?
In a Leap year, there are 29 days in the month of February (which means that there are 366 days in that year) and a Leap Year arrives in the gap of every four years.
Mathematically,
- If the year is divisible by 4, it is a leap year.
- However, if the year is divisible by 100, it is not a leap year, unless… the year is also divisible by 400, in that case it is a leap year.
For Example: 2000, 2004, 2008, 2012, 2016, 2020, … etc are Leap years.
Now let us understand the implementation part with the help of a flowchart.
Flowchart for Leap Year
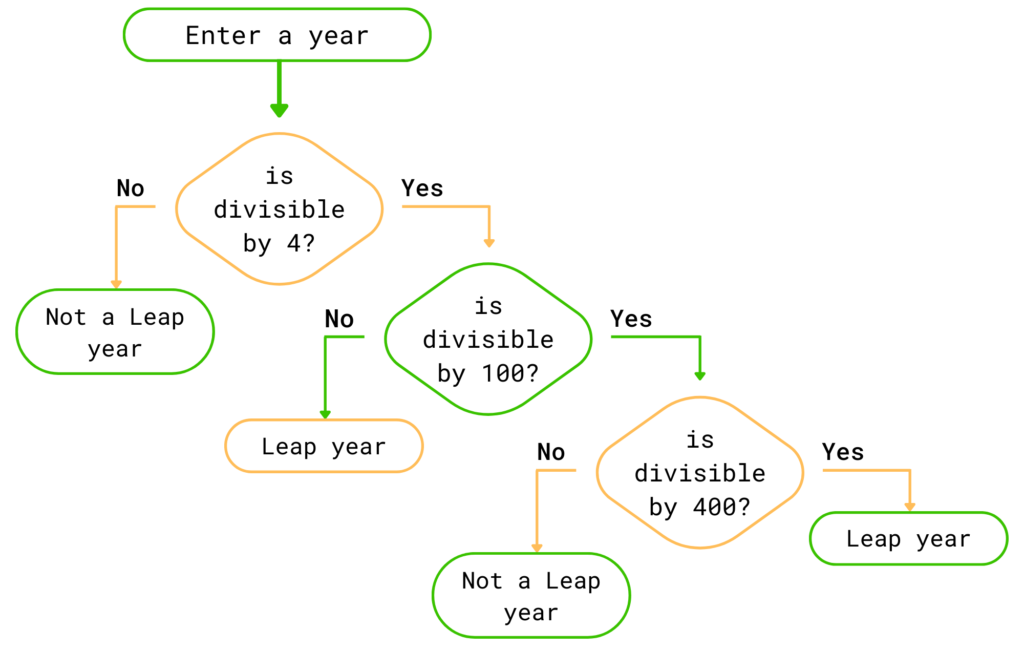
Now we know how to implement a python program to check if a year is a leap year or not but before writing a program few programming concepts you have to know:
Source Code
year = int(input('Enter your Year: '))
if year % 4 == 0:
if year % 100 == 0:
if year % 400 ==0:
print(f"{year} is a Leap year")
else:
print(f"{year} is Not a Leap year")
else:
print(f"{year} is a Leap year")
else:
print(f"{year} is Not a Leap year")
Output-1
Enter your Year: 2000 2000 is a Leap year |
Output-2
Enter your Year: 2010 2010 is Not a Leap year |
Let us modify the above program and write it using a Function.
Leap Year Program in Python Using a Function
A question may be asked Like: Write a Python program to check if a year is a leap year or not using a function.
Few programming concepts you have to know before writing this program:
Source Code
def check_leap_year(year):
if year % 4 == 0:
if year % 100 == 0:
if year % 400 ==0:
return f"{year} is a Leap year"
else:
return f"{year} is Not a Leap year"
else:
return f"{year} is a Leap year"
else:
return f"{year} is Not a Leap year"
my_year = int(input('Enter your Year: '))
print(check_leap_year(my_year))
Output-1
Enter your Year: 1992 1992 is a Leap year |
Output-2
Enter your Year: 2002 2002 is Not a Leap year |