In programming, anagrams are useful in various fields such as cryptography, natural language processing, and game development. Checking if two strings are anagram is a common problem in programming interviews and challenges. In this article, we will discuss how to check if two python strings are Anagrams or not with a detailed explanation and example.
Before we dive into the topic, let’s first define what is an anagram.
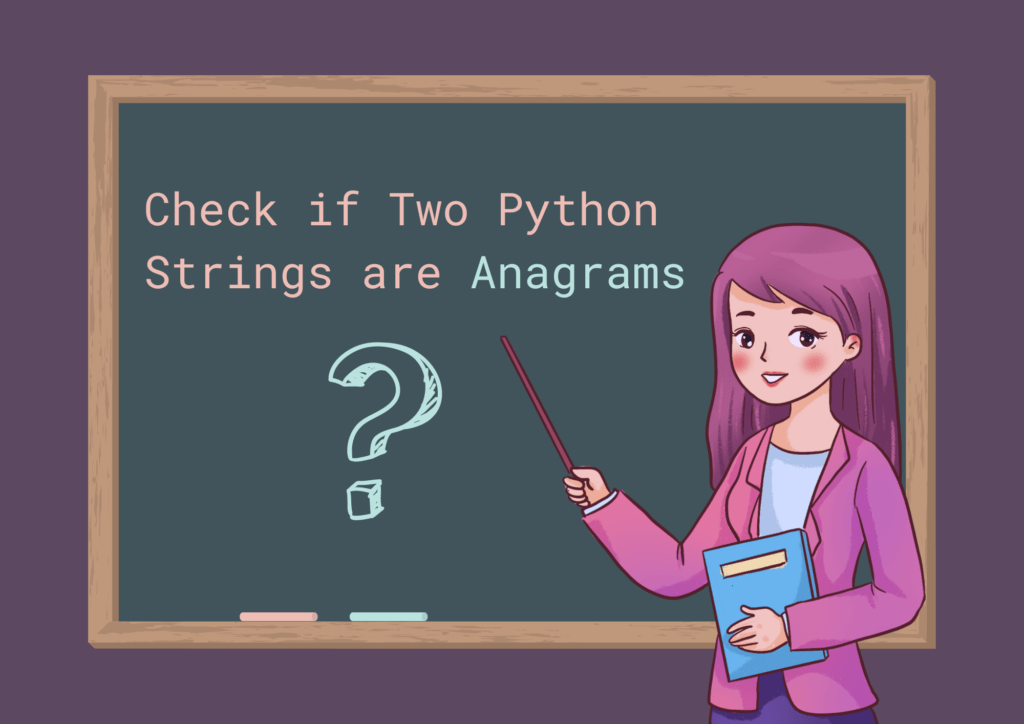
What is an Anagrams?
An anagram is a word or phrase formed by rearranging the letters of another word or phrase. For example, the word “listen” can be rearranged to form the word “silent.” Both words have the same letters but in a different order.
Similarly, when we talk about strings, two strings are said to be anagrams of each other if they have the same characters but in a different order. For example, the strings “restful” and “fluster” are anagrams of each other because they contain the same characters but in a different order.
Now we have a basic understanding of what an anagram is, let’s move on to the main topic of how to check whether two python strings are anagrams of each other or not.
Algorithm
- Get the input strings from the user and store them in two separate variables.
- Check if the length of both strings is the same. If not, they cannot be anagrams.
- Else convert both strings to lowercase (or uppercase) to ignore case sensitivity.
- Sort both strings using the
sorted()
function. - Compare the two sorted strings. If they are equal, the strings are anagrams.
- If the sorted strings are not equal then strings are not anagrams.
From the above algorithm, we can easily write a python program to check if two strings is an anagram or not but before writing a program few programming concepts you have to know:
- how to take input from the user
- if-else
sorted()
function in python
Source Code
# get input strings from user
string1 = input("Enter the first string: ")
string2 = input("Enter the second string: ")
# check if strings are of equal length
if len(string1) != len(string2):
print("The strings are not anagrams.")
else:
# convert strings to lowercase and sort them
sorted_string1 = sorted(string1.lower())
sorted_string2 = sorted(string2.lower())
# compare the two sorted strings
if sorted_string1 == sorted_string2:
print("The strings are anagrams.")
else:
print("The strings are not anagrams.")
Output
Enter the first string: listen Enter the second string: silent The strings are anagrams. |
Now let us modify the above program and write it using a user-defined function
Python Program to Check if Two strings are Anagrams Using Function
But before writing this program few programming concepts you have to know:
- how to take input from the user
- if-else
sorted()
function in python- Python Functions
Source Code
def is_anagram(str1, str2):
if len(str1) != len(str2):
return False
else:
return sorted(str1.lower()) == sorted(str2.lower())
string1 = input("Enter the 1st string: ")
string2 = input("Enter the 2nd string: ")
if is_anagram(string1,string2):
print("The strings are anagrams.")
else:
print("The strings are not anagrams.")
Output
Enter the 1st string: restful Enter the 2nd string: fluster The strings are anagrams. |
FAQs
Here are some frequently asked questions related to checking whether two strings are anagrams of each other:
Q. Can an empty string be an anagram?
Answer. No, an empty string cannot be an anagram because it does not contain any characters.
Q. Does the order of characters matter in anagrams?
Answer: No, the order of characters does not matter in anagrams. Two strings are said to be anagrams of each other if they contain the same characters but in a different order.