In this post, we will learn how to find the volume of a cylinder in Python with a detailed explanation and example. So let’s start with the very basics, that is, the formula. First, we will see the formula; after that, we will see the steps (algorithms) for how we can implement it in Python, and then we will finally jump to the coding part. This whole process is very beginner-friendly, so let’s get started.
Formula For Volume of Cylinder
Volume of cylinder (V) = πr2h
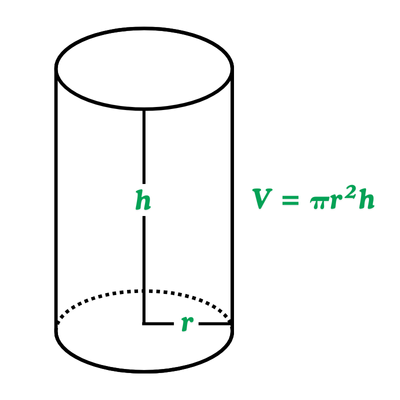
Where π (pie) is constent, and value of π is 3.14
r = Radius of the cylinder
h = Height of the cylinder
After knowing the formula, it is very simple to implement it in Python. You just need to know a few basic concepts of Python, such as:
By using these two simple Python concepts, we implement a dynamic Python program where the user enters the radius (r) and height of the cylinder, and our program calculates its volume. Here are the steps:
Algorithm
- Ask the user to enter the height (h) and radius (r) of the cylinder, and store them in variables called
height
andradius
, respectively. - Create a variable called
volume
and do:volume = 3.14 * (radius * radius) * height
- At the end print the
volume
.
These three simple steps create a Python program that calculates the volume of a cylinder by taking input from the user. Let’s see it practically.
Source Code
height = float(input("Enter Height of the Cylinder: "))
radius = float(input("Enter radius of the Cylinder: "))
volume = 3.14 * (radius*radius) * height
print(f"Volume of the Cylinder is: {volume:.2f}")
Output
Enter Height of the Cylinder: 5
Enter radius of the Cylinder: 2
Volume of the Cylinder is: 62.800
If you want to modify the above program and write it using a Python function, then we can also do that very easily. Let’s do it practically and see the output.
Find Volume of a Cylinder in Python Using a Function
To implement a Python program that calculates the volume of a cylinder using a function, you should be familiar with Python function concepts such as defining a function and how a function returns a result. For now, let’s see practically how we will use these concepts to write our program.
Source Code
def volume_of_cylinder(h, r):
return 3.14 * (r*r) * h
height = float(input("Enter Height of the Cylinder: "))
radius = float(input("Enter radius of the Cylinder: "))
print(f"Volume of the Cylinder is: {volume_of_cylinder(height,radius):.2f}")
Output
Enter Height of the Cylinder: 7
Enter radius of the Cylinder: 4
Volume of the Cylinder is: 351.68
This is all about how we can calculate the volume of a cylinder in Python. I hope this post helps you and adds some value to your programming journey. Thank you for reading, and see you in the next article.