In this post, we will learn about dictionary comprehension, including its syntax, and implementation of dictionary comprehension with if-else statements, and provide detailed explanations along with simple examples.
So let’s start with the very basic question ‘What is dictionary comprehension?’
What is Dictionary Comprehension?
Dictionary comprehension is a short-hand method to create a new dictionary from the existing iterables such as lists, tuples, etc.
It is very similar to list comprehension, with the only difference being that we pass key-value
pairs instead of individual elements in dictionary comprehension.
Syntax of Dictionary Comprehension
{key_expression: value_expression for i in iterable}
For Example:
Suppose you want to create a dictionary that includes numbers from 1 to 10 as keys and their respective squares as the corresponding values. You can do it in just one line using dictionary comprehension.
square = {num: num**2 for num in range(1,11)}
print(square)
Output:
{1: 1, 2: 4, 3: 9, 4: 16, 5: 25, 6: 36, 7: 49, 8: 64, 9: 81, 10: 100}
Explanation:
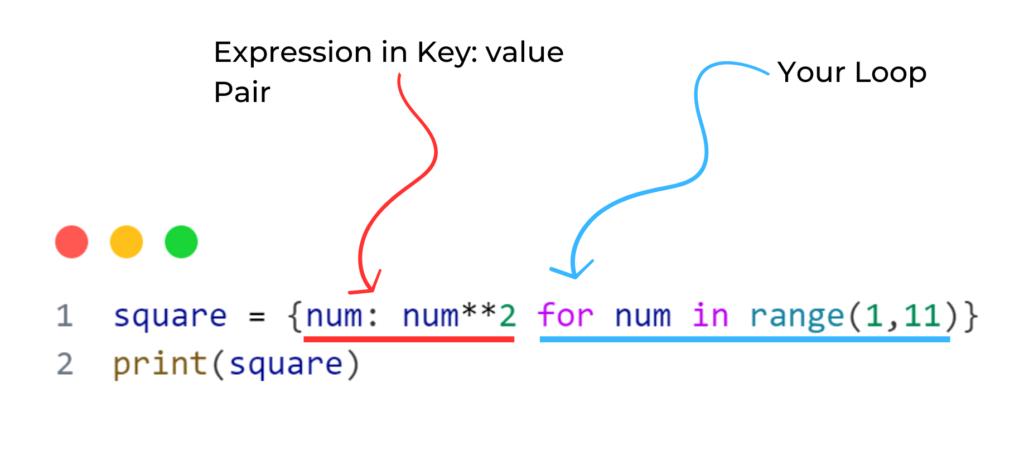
Dictionary Comprehension with If Statement
You can also include conditions while creating a dictionary using dictionary comprehension. Let’s understand this concept with the help of an example.
Syntax:
{key_expression: value_expression for i in iterable if Condition == True}
Example-1: Create a dictionary that stores even numbers from 1 to 10 as keys and their squares as the corresponding values.
d1 = {num: num**2 for num in range(1,11) if num%2 == 0}
print(d1)
Output:
{2: 4, 4: 16, 6: 36, 8: 64, 10: 100}
Explanation:
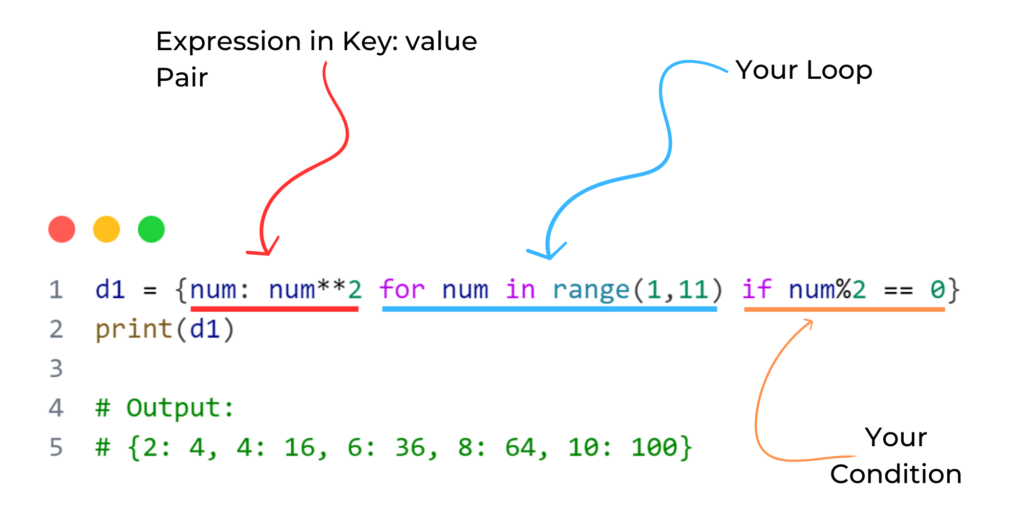
Example-2:
Suppose you have a list called my_list = [1, 2, 7, 89, 12, 45, 28, 90, 3, 32, 5]
, and you want to create a dictionary where the even numbers from the list serve as keys, and their corresponding values are labeled as ‘Even‘.
my_list = [1, 2, 7, 89, 12, 45, 28, 90, 3, 32, 5]
d1 = {num: 'Even' for num in my_list if num%2 == 0}
print(d1)
Output:
{2: 'Even', 12: 'Even', 28: 'Even', 90: 'Even', 32: 'Even'}
In this example, we iterate over each number num
in my_list
. We include the number in the dictionary only if it is even, which is checked using the condition num % 2 == 0
. For each even number, we set the key-value pair in the dictionary, where the number serves as the key, and the value is set as ‘Even‘.
Dictionary Comprehension with If-else Statement
Let’s understand it with the help of an example. Suppose you have a list called l1
with elements [1, 2, 3, 4, 5]
, and you want to assign a label to each element as either ‘odd‘ or ‘even‘.
l1 = [1,2,3,4,5]
my_dict = {num:("Even" if num%2 == 0 else "Odd") for num in l1}
print(my_dict)
Output:
{1: 'Odd', 2: 'Even', 3: 'Odd', 4: 'Even', 5: 'Odd'}
Explanation:
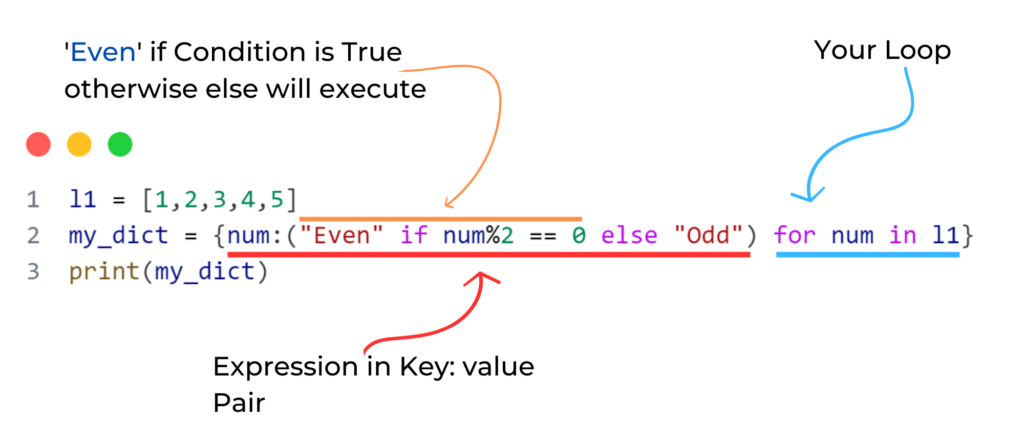
Example for Practice
Example 1: Count the length of strings present inside the list using dictionary comprehension.
fruits = ['apple', 'banana', 'cherry', 'date', 'elderberry']
fruit_lengths = {fruit: len(fruit) for fruit in fruits}
Output:
{'apple': 5, 'banana': 6, 'cherry': 6, 'date': 4, 'elderberry': 10}
Example-2:
Suppose we have a list of numbers called numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
, and we want to create a dictionary where the keys are the numbers, and the values are lists of their divisors.
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
divisor_dict = {num: [divisor for divisor in range(1, num+1) if num % divisor == 0] for num in numbers}
print(divisor_dict)
Output:
{1: [1], 2: [1, 2], 3: [1, 3], 4: [1, 2, 4], 5: [1, 5], 6: [1, 2, 3, 6], 7: [1, 7], 8: [1, 2, 4, 8], 9: [1, 3, 9], 10: [1, 2, 5, 10]}
In this example, we iterate over each number in the numbers
list. For each number, we use a list comprehension [divisor for divisor in range(1, num+1) if num % divisor == 0]
to find all the divisors of that number. The number is set as the key, and the list of divisors is set as the value in the dictionary comprehension.
Example-3: count characters of string Using dictionary comprehension.
my_string = "allinpython"
counting = {i : my_string.count(i) for i in my_string}
print(counting)
Output:
{'a': 1, 'l': 2, 'i': 1, 'n': 2, 'p': 1, 'y': 1, 't': 1, 'h': 1, 'o': 1}
Example-4:
Suppose we have a list of names called names = ['Alice', 'Bob', 'Charlie', 'David', 'Eve']
, and we want to create a dictionary where the keys are the names, and the values are the counts of vowels in each name.
names = ['Alice', 'Bob', 'Charlie', 'David', 'Eve']
vowel_counts = {name: sum([1 for letter in name if letter.lower() in 'aeiou']) for name in names}
print(vowel_counts)
Output:
{'Alice': 3, 'Bob': 1, 'Charlie': 3, 'David': 2, 'Eve': 2}
In this example, we iterate over each name in the names
list. For each name, we use a list comprehension [1 for letter in name if letter.lower() in 'aeiou']
to count the number of vowels in the name. The sum()
function is used to sum up the counts of vowels. Finally, we set the name as the key and the vowel count as the value in the dictionary comprehension.