In this post, we will learn how to write a python program to reverse a string using recursion with a detailed explanation and algorithm.
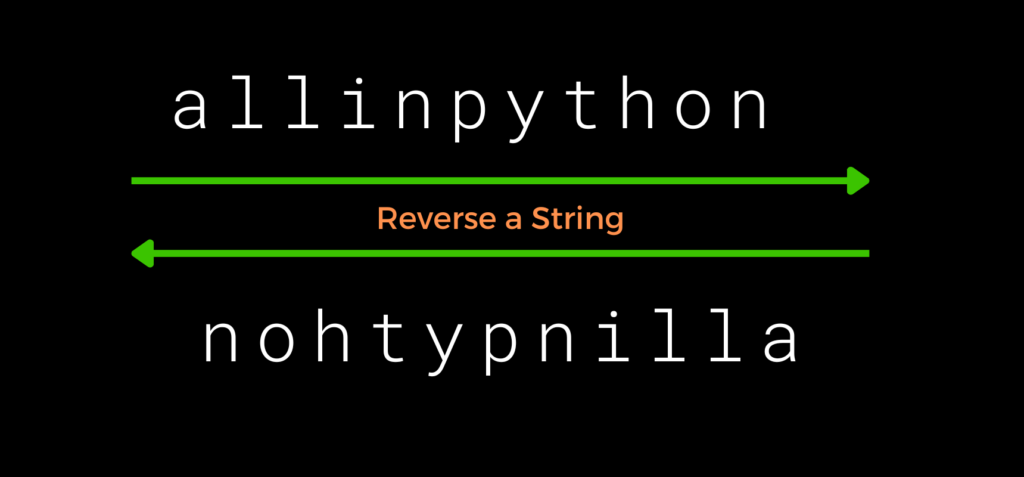
With the help of the recursive function and slicing operator, we can easily reverse a String in python but before we jump into writing a program few programming concepts you have to know and that is:
- How to take user-input
- Slicing in Python
- Python Function &
- how recursive function work
Table of Contents
hide
Algorithm to Reverse a String Using Recursion
- Define a function (
def reverse_str(my_str)
). - check
if len(my_str) == 0
returnmy_str
else
return a recursive functionreverse_str(my_str[1:]) + my_str[0]
These three steps help you to reverse a string using recursion.
Source Code
def reverse_str(my_str):
if len(my_str) == 0:
return my_str
else:
return reverse_str(my_str[1:]) + my_str[0]
my_string = input('Enter your string:')
print(f"Given String: {my_string}")
print(f"reversed String: {reverse_str(my_string)}")
Output
Given String: allinpython reversed String: nohtypnilla |
Explanation
- The code starts with defining a function
reverse_str
that takes a string as input calledmy_str
. - Inside the function, there is an if statement that checks if the length of the
my_str
string is equal to zero then it will returnmy_str
as it is. - else the function calls itself but with a modified string
my_str[1:]
. This slicing removes the first character of the string. - The result of the recursive call is then concatenated with the first character of the original
my_str
string (my_str[0]
), and the result is returned. - 2nd, 3rd and 4th steps are repeated until the length of the string is zero (
len(my_str) == 0
). - after that, we take input from the user and store it in the
my_string
variable. - Call the function
reversed_str(my_string)
and print the result.