In this post, we will learn how to read text files in Python with detailed explanations and examples.
Reading a text file is one of the most common and important tasks when working on any file-handling operations. Therefore, it is crucial to understand how to open a file and read its content. In this discussion, we cover all the necessary concepts along with examples to help you grasp the concepts easily.
Open the Text File in Python
Before reading a text file first, you need to open it in Python. there are two ways to open files in Python
- Using
open()
function - Using
with
block (or context manager).
We will see both ways to open a text file in Python. Suppose we have one .txt
file with the name ‘my_file1‘ in the same folder where we code. Now let’s see how we can open this txt file in Python.
Using open()
function
The open()
function is used to open files in Python with different modes.
Syntax: open(path, mode)
Here, the ‘path‘ refers to the full path of the text file, and ‘mode‘ indicates whether you want to open the file in reading (‘r’) or writing (‘w’) mode.
file = open("my_file1.txt", "r")
# After performing all the operations related to your file, you have to close it using the .close() method.
file.close()
Explanation:
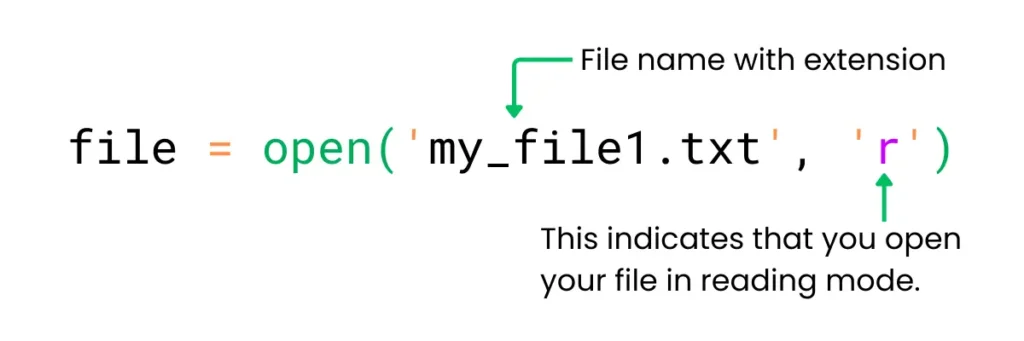
Note: In our case, the text file is in the same location as our coding file; that’s why we just specify the name of the file. However, if your file is located in any other location, you have to specify the full path (or Absolute Path).
Using with
Block
There is one advantage when we use the with
block to open our text file, and that is there is no need to close your file every time after reading it because when we open our text file in the with
block, it automatically closes the file.
Syntax: with open(path, mode) as f:
with open('my_file1.txt', "r") as f:
# here you can do all the operation related to your file.
Explanation:
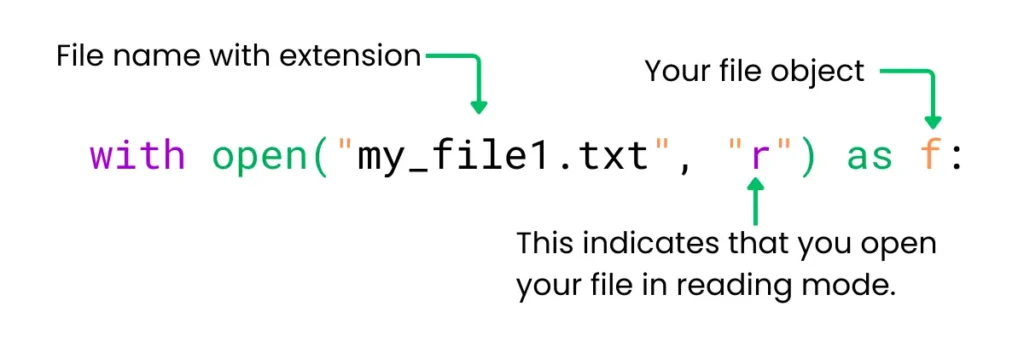
Read Text File in Python
Here is a list of methods used for reading text files in Python.
- read()
- readline() &
- readlines()
Let’s explore each of those methods one by one with examples. But before we jump into it, suppose our file ‘my_file1.txt‘ has the following content, and it is located in the same folder where we code.
my_file1.txt |
---|
Learn programming for Free with practical and project allinpython is a free learning platform. keep learning and sharing |
read() method
The read()
method is used to read a specified number of characters ( or bytes) from a file or the entire file content if no size is specified.
file = open("my_file1.txt", "r")
content = file.read() # read entire file content
print(content)
file.close() # close the file
Output:
Learn programming for Free with
practical and project
allinpython is a free learning
platform.
keep learning and sharing
Similarly, you can read a Txt file using with block, here is an example:
Read txt file using with block:
with open("my_file1.txt", "r") as f:
content = f.read()
print(content)
Output:
Learn programming for Free with
practical and project
allinpython is a free learning
platform.
keep learning and sharing
Note: For best practices, it is recommended that you open your file using the with
block.
In the read()
method, you can also specify the number of characters or bytes you want to read. For example, if you want to read only 10 characters, you can do so.
with open("my_file1.txt", "r") as f:
content = f.read(10)
print(content)
Output:
Learn prog
readline() method
The readline()
method is used to read a single line from a file at a time.
with open("my_file1.txt", "r") as f:
line1 = f.readline()
print(line1)
Output:
Learn programming for Free with
readlines() method
The readlines()
method is used to read all the lines from a file and return them as a list. Each element of the list corresponds to a line in the file.
with open("my_file1.txt", "r") as f:
content_l = f.readlines()
print(content_l)
Output:
['Learn programming for Free with \n', 'practical and project \n', 'allinpython is a free learning \n', 'platform.\n', 'keep learning and sharing']
There are a few more methods related to reading operations, such as the tell()
and seek()
methods. Let’s also explore these methods.
tell() method
The tell()
method returns the current position of the cursor in a text file. Initially, the cursor is at position 0.
In Python, the content of a file is read from the cursor’s position. If you are unfamiliar with the term ‘cursor,’ think of it as an index. Initially, it is set to 0, and as you read the file, it increases.
with open("my_file1.txt", "r") as f:
print(f.tell()) # 0
print(f.readline())
print(f.tell()) # position after reading first line
Output:
0
Learn programming for Free with
34
seek() method
The seek()
method is used to change the current position of the cursor in the file.
with open("my_file1.txt", "r") as f:
f.seek(34) # change the cursor position
print(f.tell()) # check the current position
print(f.readline()) # read single line from the current cursor position
Output:
34
practical and project
This concludes our discussion on how to read a text file in Python. I hope you now understand all the concepts related to reading operations. Thank you for reading this article!
Learn Python with lot’s of practicals and projects 👉 Click Here