In this post, we will learn about what is list comprehension, Syntax of list comprehension, list comprehension with an if statement, list comprehension with the if-else statement, nested list comprehension, and so on.
So let’s start learning from the very basics
What is List Comprehension?
List comprehension is short-hand to create a new list from an existing list or iterable.
Basically, List comprehension helps you to create a new list from an existing list in just one line.
NOTE: You can also create a completely new list using list comprehension.
Now let us create a list of squares from 1 to 10 without using list comprehension and with using list comprehension for your understanding.
Without using list comprehension:
sq_list = []
for i in range(1,11):
sq_list.append(i**2)
print(sq_list)
Output:
[1, 4, 9, 16, 25, 36, 49, 64, 81, 100]
With using list comprehension:
sq_list = [i**2 for i in range(1,11)]
print(sq_list)
Output:
[1, 4, 9, 16, 25, 36, 49, 64, 81, 100]
Creating a List of squares from 1 to 10 using list comprehension is much easier and simpler because we did it in just one line whereas we write 3-4 lines of code without using list comprehension.
Syntax of List Comprehension
my_list = [expression for i in iterable if condition == True] |
expression
means what you want to append
in the list.
For Example:
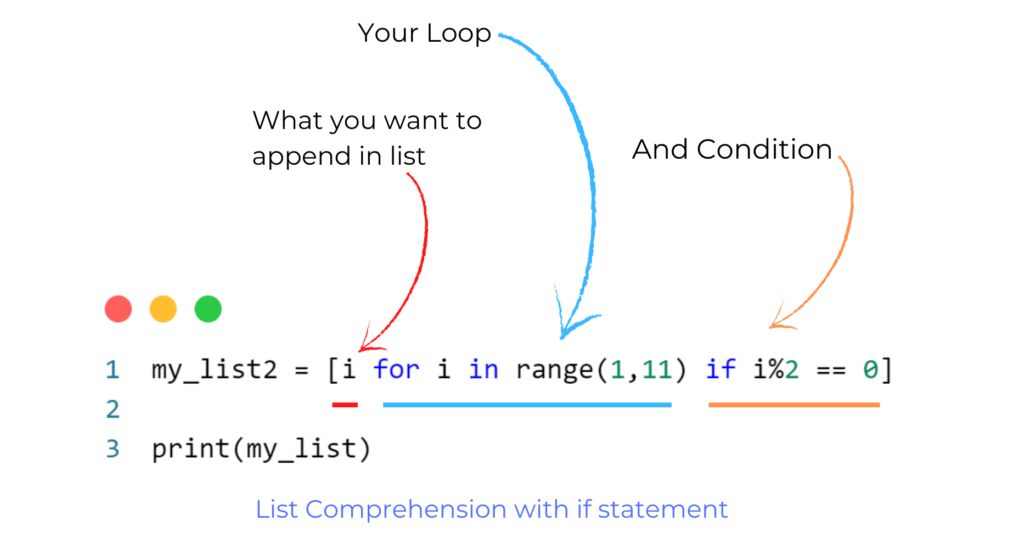
List Comprehension with if-statement
Create a list of even numbers from existing list my_list = [45,12,67,8,2,6,90,13,15]
my_list = [45,12,67,8,2,6,90,13,15]
my_list2 = [i for i in my_list if i%2 == 0]
print(my_list2)
Output:
[12, 8, 2, 6, 90]
Examples for practice
Filter integer or float numbers from the list
l1 = ['harsh',3,'roy','nil',1.1,25,2.1]
l2 = [i for i in l1 if (type(i) == int or type(i) == float)]
print(l2)
Output:
[3, 1.1, 25, 2.1]
Create a list that contains the first character of an existing list.
my_fruit = ['Apple','Banana','Orange','Mango']
my_fruit2 = [i[0] for i in my_fruit]
print(my_fruit2)
Output:
['A', 'B', 'O', 'M']
List Comprehension with if-else statement
Syntax of List comprehension with if-else statement
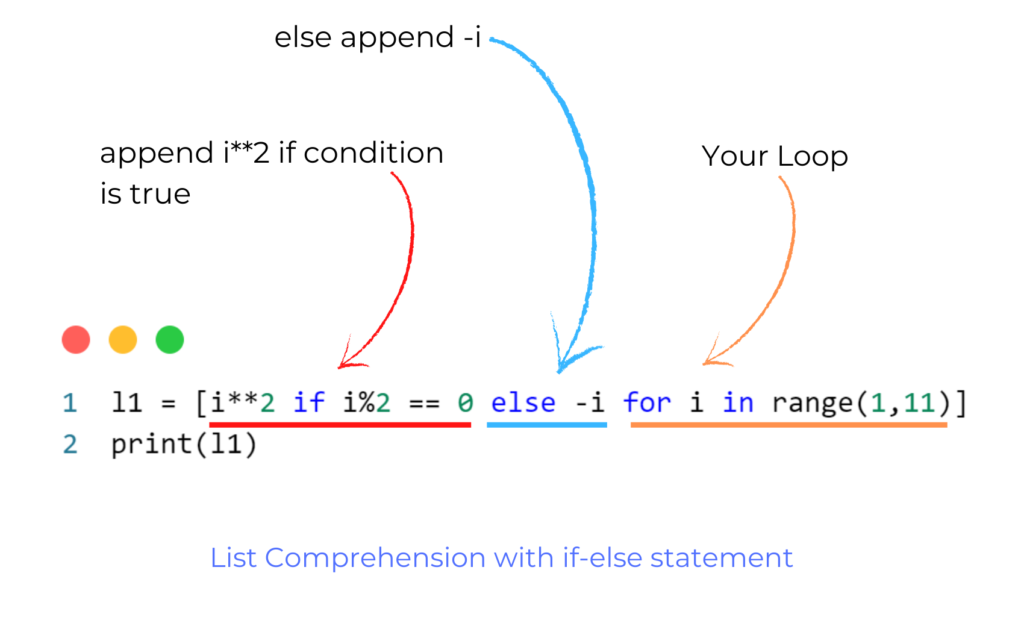
Example:
mixed_list = ['Apple','Banana',12,15,7,2,3,'Orange','Mango']
# if type is equal to int then append square of the number
# otherwise append first character of string
mixed_list2 = [i**2 if type(i) == int else i[0] for i in mixed_list]
print(mixed_list2)
Output:
['A', 'B', 144, 225, 49, 4, 9, 'O', 'M']
Nested List Comprehension
In nested List comprehension we append the whole list.
l2 = [[i for i in range(3)] for j in range(3)]
print(l2)
Output:
[[0, 1, 2], [0, 1, 2], [0, 1, 2]]