In this post, you will learn how to reverse a list using recursion in python with a detailed explanation and algorithm.
To reverse a list using recursion we use a two-pointer approach. In this approach, we take two pointers L & R initially L point to the first element of the list and R points to the last element of the list.
Let’s understand whole concepts with the help of an example.
Explanation
Take one list L1 = [3,1,5,4,7]
where initially L points to the first element of the list and R points to the last element of the list as shown below.
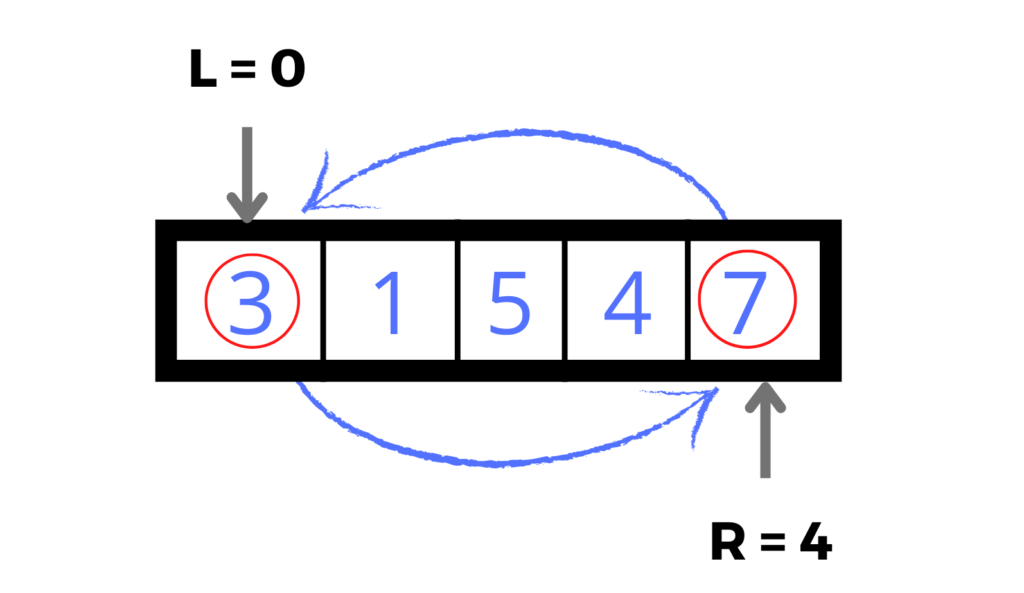
First swap the element at L with the element at R after that increment L (L += 1
) & decrement R (R -= 1
).
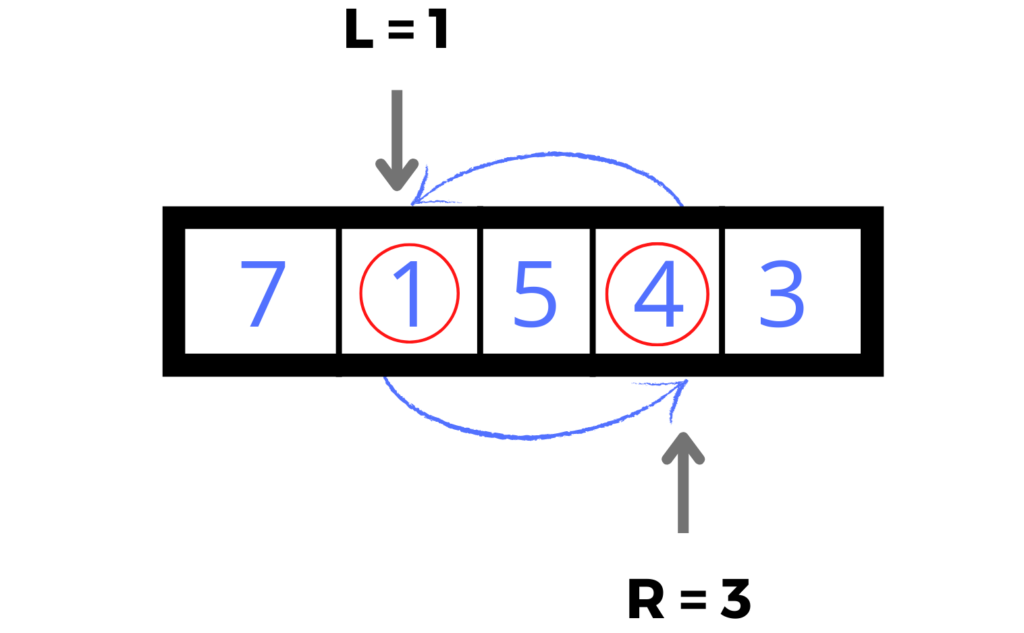
repeat the above step ( first swap the element at L with the element at R after that increment L & decrement R).
if L >= R
that means we have completed the task to reverse a list.
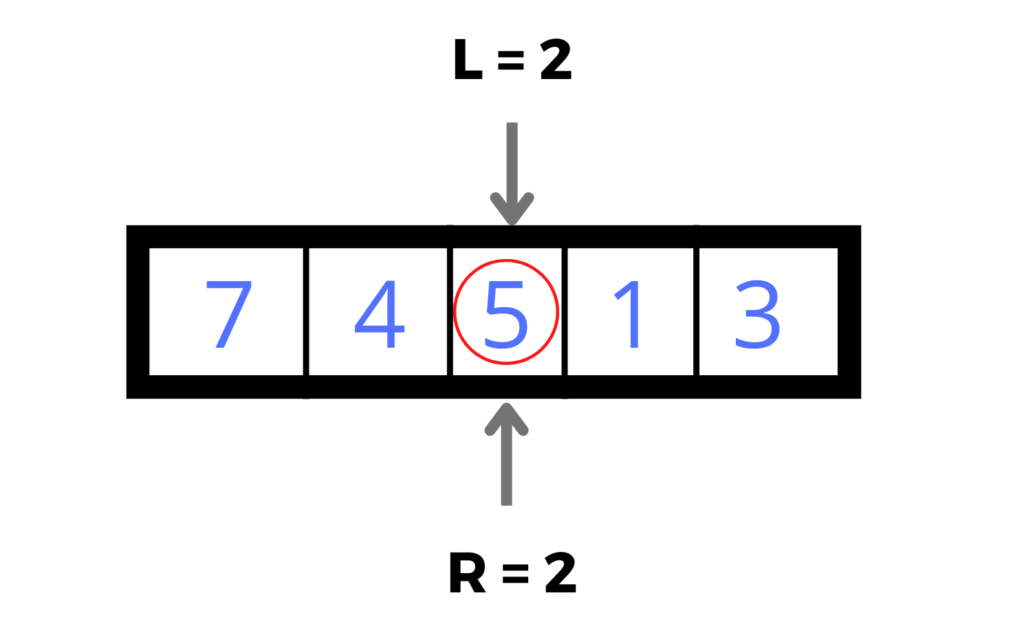
Here in this case L == R
means the list is reversed.
reverse of the list l1 = [7,4,5,1,3]
.
Algorithm to Reverse a list using recursion
- Take two-pointers L & R.
- L point to the first element of the list and R point to the last element of the list.
- if
L >= R
then returns your list (return my_list
). - otherwise, swap
my_list[L]
withmy_list[R]
- At the end return recursive function
reverse_list(my_list,L+1,R-1)
.
Now we have understood how to write a program to reverse a list using recursion through the above algorithm.
But before we write a program few concepts you have to know:
- if-else
- function in python &
- How recursive function work
Source code
def reverse_list(my_list, l, r):
if l >= r:
return my_list
#swap
tem = my_list[l]
my_list[l] = my_list[r]
my_list[r] = tem
return reverse_list(my_list,l+1,r-1)
my_l = [3,1,5,4,7]
L = 0
R = len(my_l)-1
print('my list:',my_l)
print('reverse list :',reverse_list(my_l,L,R))
Output
my list: [3, 1, 5, 4, 7]
reverse list : [7, 4, 5, 1, 3]
Fun Fact: In python, it is very simple to reverse a list, you just use slicing and step argument to reverse a list (list_name[::-1]
)
But for recursion, these are general steps you have to follow.