In this post, we will learn how to merge two dictionaries in Python with detailed explanations and examples.
If you are not familiar with Python dictionary, checkout our this article 👉 Explain Python Dictionary in detail
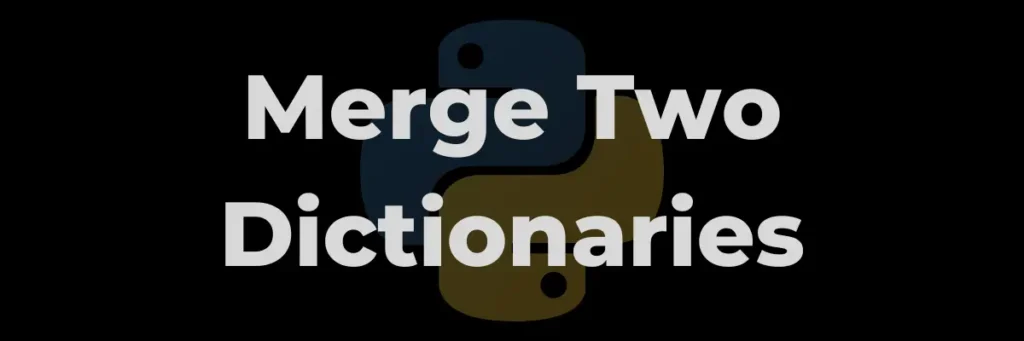
Merging or concatenating two Python dictionaries is a very common task that every programmer does in their daily life. Today we show different approaches to do this so that you are familiar with it and also learn the best suited ways for you to merge two Python dictionaries.
So let’s start learning…
Using update() Method
Using the update() method, we will merge or concatenate two Python dictionaries. Basically, the update method adds one dictionary into another dictionary.
dict1 = {'a': 1, 'b': 2}
dict2 = {'b': 3, 'c': 4}
dict1.update(dict2)
print(dict1)
Output:
{'a': 1, 'b': 3, 'c': 4}
NOTE: If a duplicate key is found in a dictionary during an update operation in Python, the value of the existing key will be replaced by the new value provided. In our case, the value associated with the key ‘b’ in dict1
, originally 2, is replaced with the value ‘3’ from dict2
.
Using the **kwargs unpacking operator
This is also a very easy and simple way to merge two dictionaries that many Python developers may not know.
dict1 = {'a': 1, 'b': 2}
dict2 = {'c': 3, 'd': 4}
dict3 = {**dict1, **dict2}
print(dict3)
Output:
{'a': 1, 'b': 2, 'c': 3, 'd': 4}
Using the dict() Constructor with **kwargs unpacking
dict1 = {'a': 1, 'b': 2}
dict2 = {'c': 3, 'd': 4}
concatenated_dict = dict(dict1, **dict2)
print(concatenated_dict)
Output:
{'a': 1, 'b': 2, 'c': 3, 'd': 4}
Using “|“ operator
In recent Python versions (Python 3.9 and later), the |
operator can be used to concatenate dictionaries. This operator performs a union operation on dictionaries, combining their key-value pairs.
dict1 = {'a': 1, 'b': 2}
dict2 = {'c': 3, 'd': 4}
dict3 = dict1 | dict2 # perfrom union operation on dictionaries
print(dict3)
Ouput:
{'a': 1, 'b': 2, 'c': 3, 'd': 4}
Using dict() constructor and union operator (|)
This is one of the less commonly used methods to merge or concatenate Python dictionaries, but it’s still important for you to know.
dict1 = {'a': 1, 'b': 2}
dict2 = {'c': 3, 'd': 4}
dict3 = dict(dict1.items() | dict2.items())
print(dict3)
Output:
{'a': 1, 'b': 2, 'c': 3, 'd': 4}
These are all the ways to merge or concatenate two Python dictionaries. I hope this post adds some value to your life. Thank you for reading this article. See you soon in the next one.