In this post, we will learn Python list in detail with very basic explanations and examples. So let’s begin with the very basic question ‘What is a list in Python’.
What is the List in Python?
The list is one type of built-in data structure in python and it is used to store an ordered collection of items in a single variable.
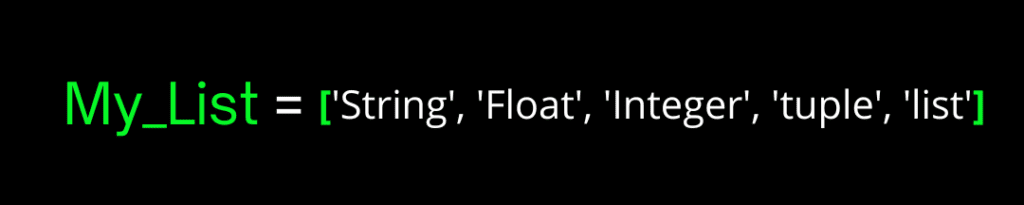
Create a list
To create a list in Python write comma-separated values inside a square bracket ([ ]) or you can use the list()
function to create a list in Python.
Example:
my_list = [1,4,6,8,9]
print(my_list)
Output:
[1, 4, 6, 8, 9]
Create a list using list() function
Example:
num_list = list(range(1,11))
print(num_list)
Output:
[1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
Mixed list
You can store anything in a list (like int, float, string, boolean, tuples, list, dictionary, etc..,)
Example:
mixed_list = ["first name",34.5,45,True]
print(mixed_list)
Output:
['first name', 34.5, 45, True]
Access elements of list
You can access any values inside the list using indexing in python.
Example:
My_string = ["saurabh","ibrahim","ram","syam"]
# indexing starts from 0
# "saurabh" ---> at 0 index
# "ibrahim" ---> at 1 index
# "ram" ---> at 2 index
# "syam" ---> at 3 index likewise
print(My_string[0])
print(My_string[3])
print(My_string[1])
# you can also use negative indexing.
# negative indexing starts from -1
# "syam" ---> at -1 index
# "ram" ---> at -2
# "ibrahim" ---> at -3
# "saurabh" ---> at -4 likewise
print(My_string[-1])
print(My_string[-3])
Output:
saurabh
syam
ibrahim
syam
ibrahim
Similarly, you can also use slicing and step-argument in the list.
Create empty list
There are two ways to create an empty list in python.
- using square bracket
- using
list()
function
Example:
# using square breaket
my_list1 = []
# using list function
my_list2 = list()
print(my_list1) # []
print(my_list2) # []
Output:
[]
[]
Add data in list
There are three methods used to add data to the list and they are.
- append()
- insert()
- extend()
append() method
append() method is used to add data at the end of the list.
Syntax –> list_name.append(data)
Example:
my_num1 = [1,2,3]
print(my_num1)
my_num1.append(4) # add 4
print(my_num1)
my_num1.append(45) #add 45
print(my_num1)
Output:
[1, 2, 3]
[1, 2, 3, 4]
[1, 2, 3, 4, 45]
insert() method
insert() method is used to add data at a specific index in the list.
Syntax –> list_name.insert(index_value, data)
Example:
my_data = ['harshit','rahul','raj']
print(my_data)
my_data.insert(1,'saurabh')
print(my_data)
my_data.insert(0,'allinpython.com')
print(my_data)
Output:
['harshit', 'rahul', 'raj']
['harshit', 'saurabh', 'rahul', 'raj']
['allinpython.com', 'harshit', 'saurabh', 'rahul', 'raj']
extend() method
extend() method is used to add the whole data of the list into another list.
syntax –> list_name_1.extend(list_name_2)
Example:
my_data1 = ['A','B','C','D']
my_data2 = ['E','F','G','H']
my_data1.extend(my_data2)
print(my_data1)
Output:
['A', 'B', 'C', 'D', 'E', 'F', 'G', 'H']
Delete data from list
there are two methods and one operator (keyword) use to delete data from the list and they are.
- pop() method
- remove() method
- del keyword
pop() method
pop() method is used to remove data at the end of the list.
Syntax –> list_name.pop()
Example:
my_data1 = ['A','B','C','D']
print(my_data1)
my_data1.pop()
print(my_data1)
my_data1.pop()
print(my_data1)
Output:
['A', 'B', 'C', 'D']
['A', 'B', 'C']
['A', 'B']
NOTE: In the pop method if you write the index value of data then the pop method will delete data at that particular index.
example: mydata1.pop(0) {remove data at index 0}
remove() method
remove method is used to remove specific data from the list.
Syntax –> list_name.remove(your_data)
Example:
my_list = ['harshit','rahul','raj']
print(my_list)
my_list.remove('raj')
print(my_list)
Output:
['harshit', 'rahul', 'raj']
['harshit', 'rahul']
del keyword
del keyword is used to delete data at a specific index in a list and the del keyword is also used to delete the whole list.
Syntax –> del list_name[index_value]
Example:
my_data2 = ['E','F','G','H']
print(my_data2)
del my_data2[1] #remove F
print(my_data2)
Output:
['E', 'F', 'G', 'H']
['E', 'G', 'H']
Loop in list
for loop:
fruits = ['orange', 'apple', 'pear']
for i in fruits:
print(i)
Output:
orange
apple
pear
while loop:
fruits = ['orange', 'apple', 'pear']
i = 0
while i < len(fruits):
print(fruits[i])
i+=1
Output:
orange
apple
pear
List inside list
matrix = [[1,2,3],[4,5,6],[7,8,9]]
print('list inside list: ',matrix) # this type of list is known as 2d list
print('list elments: ',matrix[0])
print('elements of list inside list: ',matrix[0][1])
print('loop in 2d list')
for i in matrix:
for j in i:
print(j)
Output:
list inside list: [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
list elments: [1, 2, 3]
elements of list inside list: 2
loop in 2d list
1
2
3
4
5
6
7
8
9
Methods of list
1. count() | 2. sort() | 3.index() |
4. reverse() | 5. clear() | 6. copy() |
7. append() | 8. insert() | 9. extend() |
10. pop() | 11. remove() | 12. len() function |
Count() Method
the count() method returns the number of times specified items appear in a list.
cars = ['Toyota','Chevrolet','Renault','Toyota']
print(cars.count('Toyota'))
Output:
2
sort() Method
The sort() method sorts the list in ascending, descending, or alphabetical order.
# ascending order
numbers = [5,2,7,1,3]
numbers.sort()
print(numbers) # output: [1, 2, 3, 5, 7]
alphabates = ['A','F','B','K','D']
alphabates.sort()
print(alphabates) #output: ['A', 'B', 'D', 'F', 'K']
# descending order
num2 = [1,5,2,6,8]
num2.sort(reverse=True)
print(num2) # output: [8, 6, 5, 2, 1]
alphabates2 = ['A','F','B','K','D']
alphabates2.sort(reverse=True)
print(alphabates2) # output: ['K', 'F', 'D', 'B', 'A']
Output:
[1, 2, 3, 5, 7]
['A', 'B', 'D', 'F', 'K']
[8, 6, 5, 2, 1]
['K', 'F', 'D', 'B', 'A']
Sorted() Function
You can also use sorted()
function to sort the list.
The main difference between the sort() method and the sorted() function is that the sorted() function returns a new sorted list but the sort() method sorts in the same list.
# ascending order
numbers = [5,2,7,1,3]
rev_num = sorted(numbers)
print(rev_num)
# descending order
num2 = [1,8,5,9,3]
rev_num2 = sorted(num2,reverse=True)
print(rev_num2)
Output:
[1, 2, 3, 5, 7]
[9, 8, 5, 3, 1]
index() Method
The index() method returns the index value of the first occurs specified item of the list.
bikes = ['Suzuki','TVS','Hero','Suzuki']
print(bikes.index('Suzuki'))
Output:
0
reverse() Method
the reverse() method is used to reverse the list.
bikes = ['Suzuki','TVS','Hero','Yamaha']
bikes.reverse()
print(bikes)
Output:
['Yamaha', 'Hero', 'TVS', 'Suzuki']
clear() Method
The clear() method is used to clear (or empty) the list.
num = [1,2,3,4,5]
num.clear()
print(num)
Output:
[]
copy() Method
the copy() method is used to create a copy of the list.
l1 = [2,3,5,7,11,13]
l2 = l1.copy()
print(l2)
Output:
[2, 3, 5, 7, 11, 13]
Hope this post helped you to learn the list in python. -thank you.