In this post, we will learn how to draw an Indian flag using Turtle in Python. Let’s first write the code and see the output. After that, we will understand each part of the code one by one.
Source Code
import turtle
from turtle import *
# setting the screen for drawing
scr = turtle.Screen()
# Defining the instance of turtle
ttl = turtle.Turtle()
speed(500)
# keeping the pen up initially
ttl.penup()
ttl.goto(-150, 125)
ttl.pendown(
)
# Drawing the Orange Rectangle first
#and then the white rectangle
ttl.color("orange")
ttl.begin_fill()
ttl.forward(400)
ttl.right(90)
ttl.forward(84)
ttl.right(90)
ttl.forward(400)
ttl.end_fill()
ttl.left(90)
ttl.forward(84)
# now drawing the Green Rectangle
ttl.color("green")
ttl.begin_fill()
ttl.forward(84)
ttl.left(90)
ttl.forward(400)
ttl.left(90)
ttl.forward(84)
ttl.end_fill()
# Drawing the central Big Blue Circle
ttl.penup()
ttl.goto(35, 0)
ttl.pendown()
ttl.color("navy")
ttl.begin_fill()
ttl.circle(35)
ttl.end_fill()
# Drawing the in-circle Big White Circle
ttl.penup()
ttl.goto(30, 0)
ttl.pendown()
ttl.color("white")
ttl.begin_fill()
ttl.circle(30)
ttl.end_fill()
#Drawing the inside Mini Blue Circles of Flag
ttl.penup()
ttl.goto(-27, -4)
ttl.pendown()
ttl.color("navy")
for j in range(24):
ttl.begin_fill()
ttl.circle(2)
ttl.end_fill()
ttl.penup()
ttl.forward(7)
ttl.right(15)
ttl.pendown()
# drawing the Smaller Blue Circle
ttl.color("navy")
ttl.penup()
ttl.goto(10, 0)
ttl.pendown()
ttl.begin_fill()
ttl.circle(10)
ttl.end_fill()
#Drawing the 24 spokes of the Indian Flag
ttl.penup()
ttl.goto(0, 0)
ttl.pendown()
ttl.pensize(1)
for j in range(24):
ttl.forward(30)
ttl.backward(30)
ttl.left(15)
#Drawing the stick of the Indian flag
ttl.color("Brown")
ttl.pensize(10)
ttl.penup()
ttl.goto(-150,125)
ttl.right(180)
ttl.pendown()
ttl.forward(500)
#to hide the turtle pen we used hideturtle
ttl.hideturtle()
#holding the output on the window
turtle.done()
Output
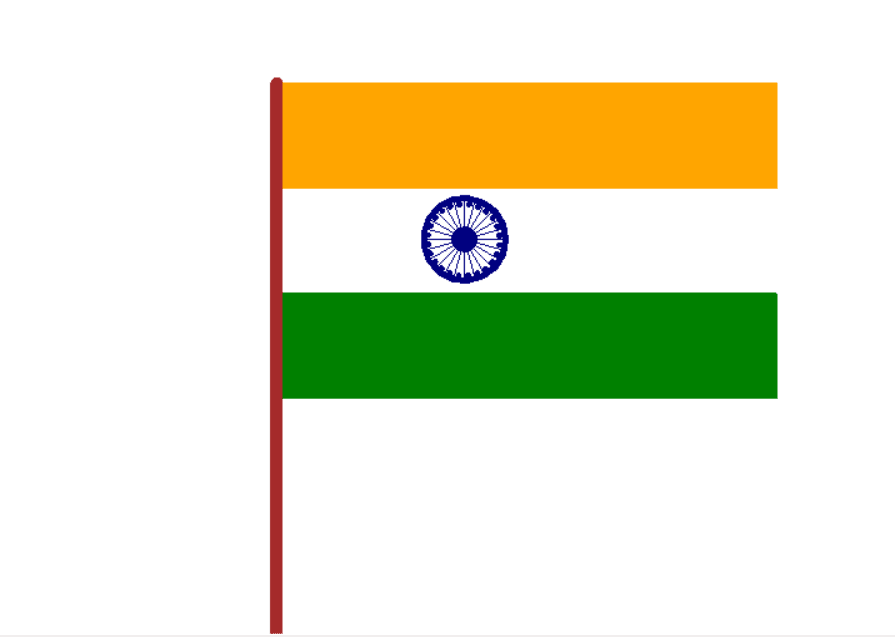
Explanation
Setting up the screen and turtle
import turtle
from turtle import *
# setting the screen for drawing
scr = turtle.Screen()
# Defining the instance of turtle
ttl = turtle.Turtle()
speed(500)
What happens here?
turtle.Screen()
: This initializes the drawing screen.turtle.Turtle()
: Creates a “turtle,” which is the pen used for drawing.speed(500)
: Sets the turtle’s drawing speed to maximum (500), so it draws quickly.
Positioning the turtle and drawing the orange rectangle
ttl.penup()
ttl.goto(-150, 125)
ttl.pendown()
# Drawing the Orange Rectangle first
ttl.color("orange")
ttl.begin_fill()
ttl.forward(400)
ttl.right(90)
ttl.forward(84)
ttl.right(90)
ttl.forward(400)
ttl.end_fill()
ttl.left(90)
ttl.forward(84)
What happens here?
penup()
lifts the pen so the turtle can move to the starting position.goto(-150, 125)
moves the turtle to the top-left corner of the screen.pendown()
lowers the pen, starting the drawing.- The turtle draws a rectangle with an orange color:
- The
forward(400)
moves the turtle forward by 400 units. - The
right(90)
turns the turtle by 90 degrees. - The
begin_fill()
starts filling the shape, andend_fill()
fills the rectangle with orange.
- The
The White Stripe:
In the Indian flag, the white stripe is the middle stripe between the orange and green stripes. The white background of the screen in the turtle graphics acts as the white stripe of the flag. So, the white part does not need to be drawn explicitly.
- Why no need to draw it?
- The background of the screen is already white by default, so the white stripe is naturally formed by the blank space between the orange and green rectangles. The turtle doesn’t need to draw anything for the white part—it’s just the empty space between the two colored stripes.
Drawing the green rectangle
ttl.color("green")
ttl.begin_fill()
ttl.forward(84)
ttl.left(90)
ttl.forward(400)
ttl.left(90)
ttl.forward(84)
ttl.end_fill()
What happens here?
- After finishing the orange rectangle, the turtle moves to draw the green rectangle just below it.
- The pen color changes to green, and the rectangle is drawn using similar commands as before but with a green fill.
Drawing the Ashoka Chakra
ttl.penup()
ttl.goto(35, 0)
ttl.pendown()
ttl.color("navy")
ttl.begin_fill()
ttl.circle(35)
ttl.end_fill()
What happens here?
penup()
andgoto(35, 0)
: The turtle moves to the center of the white stripe.color("navy")
andbegin_fill()
: The pen color changes to navy blue, and the circle drawn will be filled with blue.circle(35)
: Draws the outer blue circle of the Chakra.
Drawing the inner white circle of the Ashoka Chakra
ttl.penup()
ttl.goto(30, 0)
ttl.pendown()
ttl.color("white")
ttl.begin_fill()
ttl.circle(30)
ttl.end_fill()
What happens here?
- The turtle draws a smaller white circle inside the blue one, representing the inner circle of the Ashoka Chakra.
- The radius of the white circle is 30, and it’s centered inside the blue circle.
Drawing the mini blue circles around the inner circle (Chakra)
ttl.penup()
ttl.goto(-27, -4)
ttl.pendown()
ttl.color("navy")
for j in range(24):
ttl.begin_fill()
ttl.circle(2)
ttl.end_fill()
ttl.penup()
ttl.forward(7)
ttl.right(15)
ttl.pendown()
What happens here?
- The turtle starts near the edge of the inner white circle.
- Inside a
for
loop, it:- Draws a small filled circle (
circle(2)
). - Moves a bit forward (
forward(7)
) and rotates (right(15)
). - Repeats 24 times to create a pattern of small blue circles around the Chakra.
- Draws a small filled circle (
Drawing the smaller blue circle (center of Chakra)
ttl.color("navy")
ttl.penup()
ttl.goto(10, 0)
ttl.pendown()
ttl.begin_fill()
ttl.circle(10)
ttl.end_fill()
What happens here?
- The turtle draws a smaller blue circle inside the white circle, representing the central part of the Ashoka Chakra.
Adding 24 spokes to the Chakra
ttl.penup()
ttl.goto(0, 0)
ttl.pendown()
ttl.pensize(1)
for j in range(24):
ttl.forward(30)
ttl.backward(30)
ttl.left(15)
What happens here?
- The turtle moves to the center of the Chakra.
- Inside a
for
loop, it:- Draws a straight line forward (
forward(30)
). - Comes back to the center (
backward(30)
). - Rotates 15 degrees (
left(15)
) and repeats.
- Draws a straight line forward (
Drawing the flagpole
ttl.color("Brown")
ttl.pensize(10)
ttl.penup()
ttl.goto(-150, 125)
ttl.right(180)
ttl.pendown()
ttl.forward(500)
What happens here?
- The turtle’s pen color changes to brown, and the thickness of the pen is set to 10.
- The turtle moves to the top-left corner of the flag and draws a straight vertical line downward, representing the flagpole.
Final Touch:
ttl.hideturtle()
turtle.done()
What happens here?
hideturtle()
: Hides the turtle for a clean look.turtle.done()
: Keeps the screen open to view the drawing.
This is how you draw an Indian flag using Python turtle graphics. For more awesome Python turtle designs click here