In this post, you will learn about any() and all() function in python with detailed explanation and examples so let us start learning both functions one by one.
any() Function in Python
any()
Function returns True if any one element is True in iterable (list, tuple, etc).
Syntax : any(iterable)
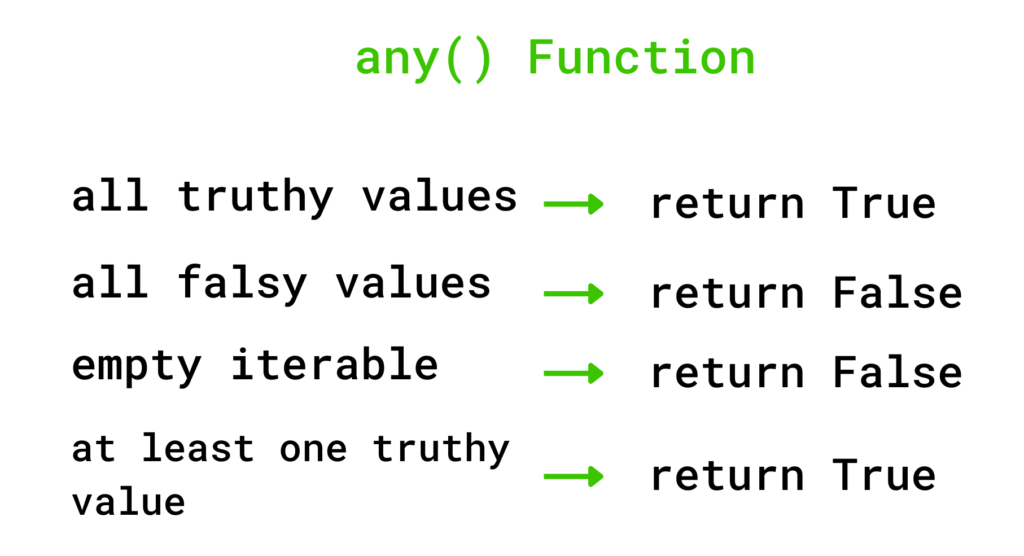
let us Understand any()
function with the help of examples.
Example – 1:
Suppose you have a list containing boolean values such as True and False. if at least one element is true inside a list, then any()
function returns True and if all the elements are false inside the list, then it returns False.
list1 = [True,False,False,False]
print(any(list1))
Output:
True
Example – 2:
list2 = [False,False,False,False]
print(any(list2))
Output:
False
Use of any() Function
Suppose you have a list of numbers and your task is to perform the addition of all the numbers present inside the list but there is one condition at least one number is a floating number then and then you can perform addition otherwise not.
This problem is easily tackled with the help of any()
function. let’s see how?
#problem : perform addition if
# ---> any one element in list is floating number
# ---> othereise print 'no floating number no addition'
my_list = [1,2,3,4,5,7]
total = 0
if any([type(num) == float for num in my_list]):
for i in my_list:
total+=i
print(f'Sum of list is {total}')
else:
print('no floating number no addition')
Output:
no floating number no addition
In our case, all the number inside the list is integer number that’s why we are not getting the sum of numbers.
NOTE: any()
function returns False if the list or tuple is empty.
all() Function in Python
all()
Function returns True if all the element is True in iterable (list, tuple, etc) otherwise it returns False.
Syntax: all(iterable)
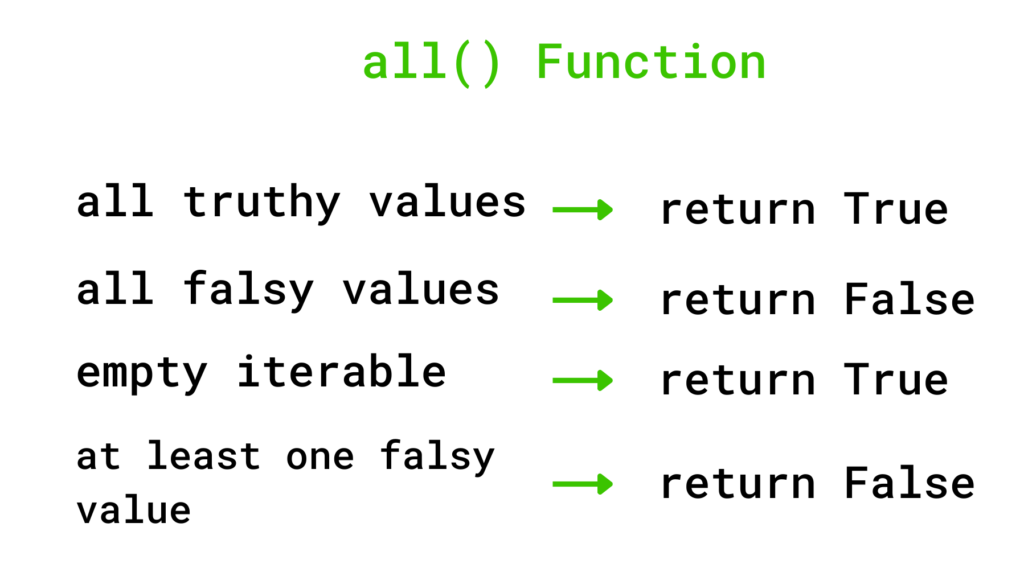
let us Understand all()
function with the help of examples.
Example – 1:
Suppose you have a list containing boolean values such as True and False. if all the element is true inside a list, then all()
function returns True and if anyone elements are false inside the list, then it returns False.
list1 = [True,True,True,True,True]
print(all(list1))
Output:
True
Example – 2:
list2 = [True,False,True,True,True]
print(all(list2))
Output:
False
Use of all() Function
there is many use of the all()
function but one of the best use of the all()
function is when you perform a mathematical operation on a list or tuple you can check whether all the elements are numeric values or not.
Let us see it with the help of an example.
my_list = [3,5,7,'allinpython','demo',True]
total = 0
if all([type(num) == int or type(num) == float for num in my_list]):
for i in my_list:
total+=i
print(f'sum of list is {total}')
else:
print('wrong input')
Output:
wrong input
In our case list is contain other datatypes also other than int
or float
that’s why we did not get a sum of the list.
NOTE: all()
function returns True if the list or tuple is empty.