Creating your own game might seem difficult, but with Python and a library called pygame
, it’s easier than you think. In this guide, we’ll show you how to build a classic Snake game in Python step by step.
Whether you’re just starting with Python or looking for a fun project to enhance your skills, this is the perfect beginner-friendly guide. By the end, you’ll have a playable game and a solid understanding of how game logic works!
So let’s get started…
What is Pygame?
Before we start, let’s understand what pygame
is. Pygame
is a free Python library that makes it easy to build games and multimedia applications. It handles things like graphics, sounds, and user input (such as the keyboard).
With that foundation in mind, let’s now dive into creating our snake game project using this step-by-step guid
Step 1: Installing Pygame
To get started, you need to install the pygame
library. If you don’t have it installed yet, run the following command:
pip install pygame
This will install the library that we needed to create the game.
Step 2: Writing the Code
Now that you have pygame
installed, let’s write the code. Below is the complete Python code for your Snake game. You can copy and paste it into a Python file (snake_game.py
).
Source Code
import pygame
import time
import random
# Initialize pygame
pygame.init()
# Colors
white = (255, 255, 255)
black = (0, 0, 0)
red = (213, 50, 80)
green = (0, 255, 0)
blue = (50, 153, 213)
# Screen size
dis_width = 800
dis_height = 600
# Create the game window
dis = pygame.display.set_mode((dis_width, dis_height))
pygame.display.set_caption('Snake Game')
# Clock to control the speed of the snake
clock = pygame.time.Clock()
# Snake settings
snake_block = 10
snake_speed = 15
font_style = pygame.font.SysFont("bahnschrift", 25)
# Function to display the score
def Your_score(score):
value = font_style.render("Your Score: " + str(score), True, white)
dis.blit(value, [0, 0])
# Function to draw the snake
def our_snake(snake_block, snake_list):
for x in snake_list:
pygame.draw.rect(dis, green, [x[0], x[1], snake_block, snake_block])
# Game loop function
def gameLoop():
game_over = False
game_close = False
x1 = dis_width / 2
y1 = dis_height / 2
x1_change = 0
y1_change = 0
snake_List = []
Length_of_snake = 1
foodx = round(random.randrange(0, dis_width - snake_block) / 10.0) * 10.0
foody = round(random.randrange(0, dis_height - snake_block) / 10.0) * 10.0
while not game_over:
while game_close:
dis.fill(black)
message = font_style.render("Game Over! Press Q-Quit or C-Play Again", True, red)
dis.blit(message, [dis_width / 6, dis_height / 3])
Your_score(Length_of_snake - 1)
pygame.display.update()
for event in pygame.event.get():
if event.type == pygame.KEYDOWN:
if event.key == pygame.K_q:
game_over = True
game_close = False
if event.key == pygame.K_c:
gameLoop()
for event in pygame.event.get():
if event.type == pygame.QUIT:
game_over = True
if event.type == pygame.KEYDOWN:
if event.key == pygame.K_LEFT:
x1_change = -snake_block
y1_change = 0
elif event.key == pygame.K_RIGHT:
x1_change = snake_block
y1_change = 0
elif event.key == pygame.K_UP:
y1_change = -snake_block
x1_change = 0
elif event.key == pygame.K_DOWN:
y1_change = snake_block
x1_change = 0
if x1 >= dis_width or x1 < 0 or y1 >= dis_height or y1 < 0:
game_close = True
x1 += x1_change
y1 += y1_change
dis.fill(black)
pygame.draw.rect(dis, blue, [foodx, foody, snake_block, snake_block])
snake_Head = []
snake_Head.append(x1)
snake_Head.append(y1)
snake_List.append(snake_Head)
if len(snake_List) > Length_of_snake:
del snake_List[0]
for x in snake_List[:-1]:
if x == snake_Head:
game_close = True
our_snake(snake_block, snake_List)
Your_score(Length_of_snake - 1)
pygame.display.update()
if x1 == foodx and y1 == foody:
foodx = round(random.randrange(0, dis_width - snake_block) / 10.0) * 10.0
foody = round(random.randrange(0, dis_height - snake_block) / 10.0) * 10.0
Length_of_snake += 1
clock.tick(snake_speed)
pygame.quit()
quit()
# Start the game
gameLoop()
Output
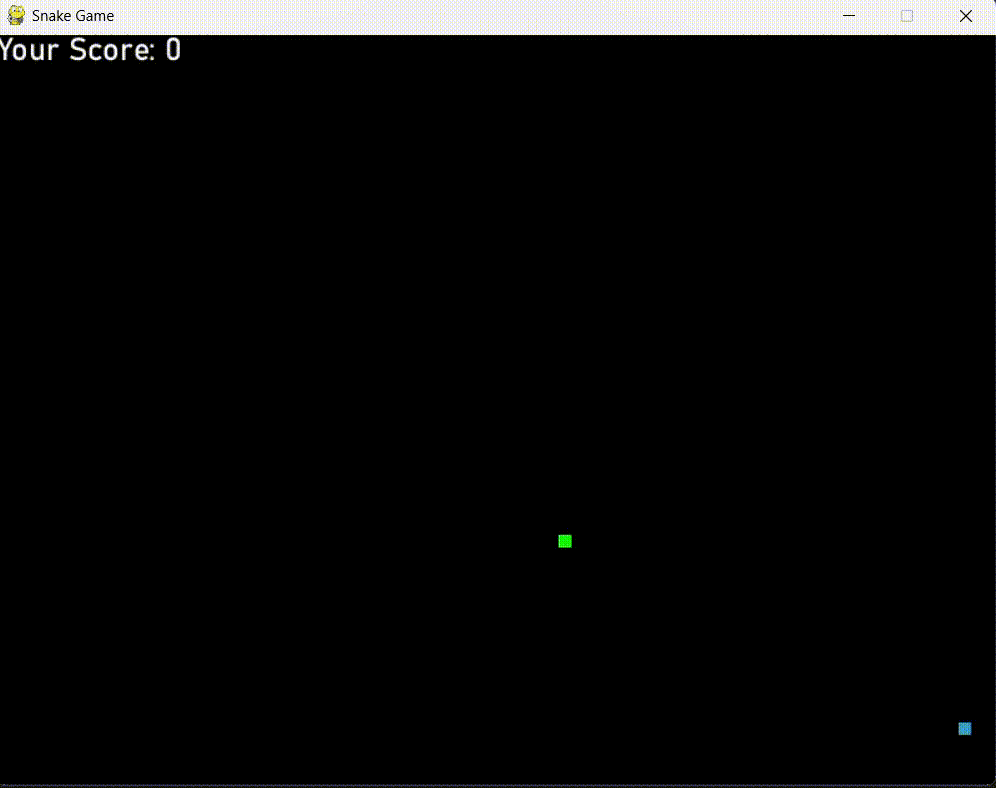
What the Code Does:
- Initializing Pygame: We begin by initializing
pygame
and defining the colors, screen size, and other settings. - Snake and Food: The snake is drawn using small rectangles. The food is a single rectangle that appears randomly on the screen.
- Movement: The snake moves when you press the arrow keys. Each time it eats food, the snake grows longer.
- Game Over: If the snake hits the wall or itself, the game is over. You can press “Q” to quit or “C” to play again.
- Displaying the Score: The score is displayed in the top left corner, showing how many pieces of food the snake has eaten.
Conclusion
Building a simple Snake game in Python is a great project to help you understand game development basics. With just a few lines of code, you’ve learned how to:
- Control a moving object with keyboard input
- Implement collision detection
- Update the game screen in real-time
I hope you enjoyed this guide! Feel free to modify the code, experiment with colors, or add new features to your game.
👉 For more Python projects click here